Chapter 0: Learn OpenTK in 15'
第0章:在15年学习OpenTK
So, you have downloaded the latest version of OpenTK - what now?
所以,现在你已经下载了最新版本的OpenTK - 接下来该怎么办?
This is a short tutorial that will help you get started with OpenTK in 3 simple steps.
这是一个简短的教程,帮助你在简单的3步之内上手OpenTK。
[Step 1: Installation]
第一步:安装
Open the zip you downloaded and extract it to a folder of your choosing. I usually create a 'Projects' folder on my desktop or in my documents but any folder will do.
打开你下载的zip文件,解压到一个文件夹。
[Step 2: Use OpenTK]
第二步:使用OpenTK
Open the folder you just extracted. Inside, you will find three solutions: OpenTK.sln, Generator.sln and QuickStart.sln. The first two contain the OpenTK source code - no need to worry about them right now. The QuickStart solution is what we are interested in.
打开你解压后的文件夹。里面,你会看到三个解决方案:OpenTK.sln,Generator.sln和QuickStart.sln。前两个包含OpenTK源码 - 不需要担心目前。QucikStart.sln才是我们目前感兴趣的。
Double-click QuickStart.sln. This will launch your .Net IDE (don't have a .Net IDE? Check out MonoDevelop or Visual Studio Express).
双击QuickStart.sln。这会加载你的.NET编辑器。(没有.NET IDE?尝试一下MonoDevelop或者Visual Studio Express)
Take a few moments to take in the contents of the QuickStart project:
花一点时间看一下QuickStart工程的目录:
Game.cs: this contains the code for the game. Scroll to the bottom: the Main() method is where everything begins.
Game.cs:这个文件包含游戏代码。划到最后的Main()函数,那里是一切开始的地方。
References: click on the '+' sign to view the project references. The 'OpenTK' reference is the only one you need in order to use OpenTK.
References:点击'+'符号查看工程的参考。'OpenTK'参考是唯一一个你使用OpenTK所需的选项。
Now, press F5 to run the project. A window with a colored triangle will show up - not very interesting, is it? Press escape to close it.
现在,按F5运行项目。一个带有彩色三角形的窗口会出现——不是很有趣,是吗?按escape将其关闭。
[Step 3: Play]
第3步:开始
Now it's time to start playing with the code. This is a great way to learn OpenGL and OpenTK at the same time.
现在是时候开始玩一下代码了。这是一种同时学习OpenGL和OpenTK很好的方式。
Every OpenTK game will contain 4 basic methods:
每一个OpenTK游戏都包含3个基本的方法:
1. OnLoad: this is the place to load resources from disk, like images or music.
这是加载资源的地方。例如图像或者音乐。
2. OnUpdateFrame: this is a suitable place to handle input, update object positions, run physics or AI calculations.
这是处理输入、更新对象位置、运行物理或AI计算的合适场所。
3. OnRenderFrame: this contains the code that renders your graphics. It typically begins with a call to GL.Clear() and ends with a call to SwapBuffers.
它包含渲染图形的代码。它通常以调用GL.Clear()开始,以调用SwapBuffers结束。
4. OnResize: this method is called automatically whenever your game window changes size. Fullscreen applications will typically call it only once.
每当你的游戏窗口改变大小时,这个方法就会自动调用。全屏应用程序通常只调用它一次。
Windowed applications may call it more often. In most circumstances, you can simply copy & paste the code from Game.cs.
窗口化应用程序可能会更频繁地调用它。在大多数情况下,您可以简单地从Game.cs复制并粘贴代码。
Why don't you try modifying a few things? Here are a few suggestions:1. Change the colors of the triangle or the window background (OnLoad and OnRenderFrame methods).
你为什么不尝试修改一些东西?以下是一些建议:1.更改三角形或窗口背景的颜色(OnLoad和OnRenderFrame方法)。
2. Make the triangle change colors when you press a key (OnUpdateFrame and OnRenderFrame methods).
2.按下一个键时,使三角形改变颜色(OnUpdateFrame和OnRenderFrame方法)。
3. Make the triangle move across the screen. Use the arrow keys or the mouse to control its position (OnUpdateFrame and OnRenderFrame methods).
3.使三角形在屏幕上移动。使用箭头键或鼠标控制其位置(OnUpdateFrame和OnRenderFrame方法)。
4. Use a for-loop to render many triangles arranged on a plane (OnRenderFrame method).
4.使用for循环渲染平面上排列的许多三角形(OnRenderFrame方法)。
5. Rotate the camera so that the plane above acts as ground (OnRenderFrame method).
5.旋转相机,使上面的平面用作地面(OnRenderFrame方法)。
6. Use the keyboard and mouse to walk on the ground. Make sure you can't fall through it! (OnUpdateFrame and OnRenderFrame methods).
6.使用键盘和鼠标在地面上行走。确保你不会掉下去!(OnUpdateFrame和OnRenderFrame方法)。
Some things you might find useful: Vector2, Vector3, Vector4 and Matrix4 classes for camera manipulations. Mouse and Keyboard properties for interaction with the mouse and keyboard, respectively. Joysticks property for interaction with joystick devices.
您可能会发现一些有用的东西:用于相机操纵的Vector2、Vector3、Vector4和Matrix4类。用于分别与鼠标和键盘交互的鼠标和键盘属性。用于与操纵杆设备交互的操纵杆属性。
Don't be afraid to try things and see the results. OpenTK lends itself to explorative programming - even if something breaks, the library will help you pinpoint the cause of the error.
[Step: next]
不要害怕尝试并看到结果。OpenTK有助于探索性编程——即使出现问题,该库也会帮助您找出错误的原因。
[步骤:下一步]
There's a lot of functionality that is not visible at first glance: audio, advanced opengl, display devices, support for GUIs through GLControl... Then there's the subject of proper engine and game design, which could cover a whole book by itself.
有很多功能乍一看是看不到的:音频、高级opengl、显示设备、通过GLControl对GUI的支持……然后是正确的引擎和游戏设计,它可以单独覆盖整本书。
Hopefully, you'll have gained a feel of the library by now and you'll be able to accomplish more complex tasks. You might wish to consult the complete documentation for the more advanced aspects of OpenTK and, of course, don't hesitate to post at the forums if you hit any roadblocks!
希望您现在已经熟悉了这个库,并且能够完成更复杂的任务。您可能希望查阅OpenTK更高级方面的完整文档,当然,如果遇到任何障碍,请不要犹豫,在论坛上发布!
设置启动程序:
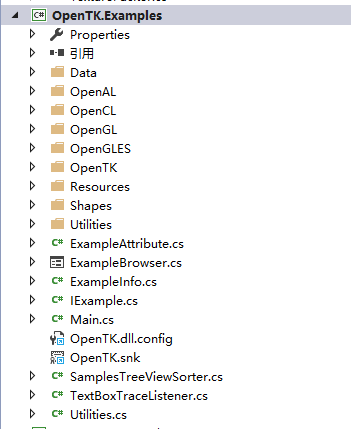
参考:
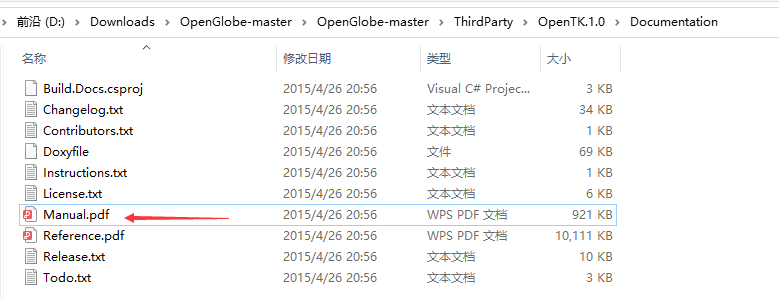
>>opentk键盘捕捉失效(Keyboard类出了问题,好像是NativeWindow.InputDriver过时了):试一下新版
NuGet\Install-Package OpenTK -Version 3.3.1
https://www.nuget.org/packages/OpenTK/3.3.1 https://opentk.net/
可以看到tests
但是OpenTK Manual.打不开
GitHub - opentk/LearnOpenTK: A port of learnopengl.com's tutorials to OpenTK and C#.