JS应用,表单上的一些东西
例:
<body> <form>我的生日是哪一年? <input type="text" value="" id="t1" name="t1" /> <input type="submit" onclick="checkName()" id="t2" name="t2" value="检查答案" /> </form> <script language="javascript"> function Space(s){ /* 这里是删去空格,如果输入了字符串 while((s.length>0)&&(s.charAt(0)==' ')) 并且第0位是空格,那么重新截取1-最后. s = s.substring(1, s.length); while循环直到不是空格的那位数,尾巴 while((s.length>0)&&(s.charAt(s.length-1)==' ')) 一样.*/ s = m.substring(0, s.length-1); return s; } function checkName() { var a = document.getElementById("t1"); a.value=Space(a.value) if(a.value.length == 0) { alert("不能为空"); } else if(a.value==1989) { alert("正确"); } else { alert("错误"); } } </script> </body>
这样看起来很麻烦,我们可以通过操作属性来判断.
通过ID获取值,var a = document.getElementById("id");
a.setAttribute("属性名","属性值"); 设置一个属性,添加或更改都可以:disabled
a.getAttribute("属性名");获取属性的值
a.removeAttribute("属性名");移除一个属性
<body> <input type="text" answer="1989" value="" id="t1" /> <input type="button" onclick="check()" value="检查答案" /> <script language="javascript"> function check() { var a=document.getElementById("t1"); var a1=a.value; var a2=a.getAttribute("answer"); if(a1==a2) { alert("答对了!"); } else { alert("再想想!"); } } </script> </body>
在标签里面添一条属性, answer="1989" 可以自己命名,然后通过js获取内容用getAttribute("answer")来对比输入的内容.
我们也可以用removeAttribute来移除一个标签属性,如果移除一个关键属性就可以实现一个功能,如
<body> <form> <input type="submit" id="b1" name="b1" value="同意(9)" disabled="disabled" /> </form> <script language="javascript"> var n=10; var a= document.getElementById("b1"); function bian() { n--; if(n==0) { a.removeAttribute("disabled"); a.value="同意"; return; } else { a.value= "同意("+n+")"; window.setTimeout("bian()",1000); //设置时间,("函数","执行间隔(毫秒)") } } window.setTimeout("bian()",1000); </script> </body>
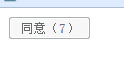
我们也可以用setAttribute来添加一个标签属性,如果移除一个关键属性就可以实现一个功能
setAttribute()里面 ("加入标签名","标签的属性值")
_________________________________________传说中的分割线___________________________________________
例子:换窗口的背图片,每隔三秒换一次,循环不止;点上一张、下一张可以切换,这时停止了自动转换。
<style type="text/css"> .pages { border:1px solid gray; padding:15px 15px 15px 15px; position:fixed; top:200px; text-align:center; color:white; background-color:#C3C; font-weight:bold; } #p1 { left:10px; } #p2 { right:10px; } </style> </head> <body style=" background-image:url(banner4.jpg)"> <iframe id="ff" src="preload.html" width="0" height="0" frameborder="0"></iframe> <div class="pages" id="p1" onclick="dodo(-1)">前一张</div> <div class="pages" id="p2" onclick="dodo(1)">后一张</div> </body> <script language="javascript"> var jpg =new Array(); jpg[0]="url(1.jpg)"; jpg[1]="url(2.jpg)"; jpg[2]="url(3.jpg)"; jpg[3]="url(4.jpg)"; var xb=0; var n=0; function huan() { xb++; if(xb == jpg.length) { xb=0; } document.body.style.backgroundImage=jpg[xb]; if(n==0) { var id = window.setTimeout("huan()",3000); } } function dodo(m) { n=1; xb = xb+m; if(xb < 0) { xb = jpg.length-1; } else if(xb >= jpg.length) { xb = 0; } document.body.style.backgroundImage=jpg[xb]; } window.setTimeout("huan()",3000); </script>
_________________________________________传说中的分割线___________________________________________
<style type="text/css"> #main { width:200px; background-color:#CCF; } .topic { font-weight:bold; text-align:center; background-color:#C39; color:white; font-size:14px; padding:5px 0px 5px 0px; margin-top:1px; } .list { border:1px solid navy; height:200px; background-color:#CFF; display:none; } </style> </head> <body> <div id="main"> <div class="topic" onclick="dodo('l1')">工作计划管理</div> <div class="list" id="l1"> </div> <div class="topic" onclick="dodo('l2')">个人资料管理</div> <div class="list" id="l2"></div> <div class="topic" onclick="dodo('l3')">部门外联管理</div> <div class="list" id="l3"></div> <div class="topic" onclick="dodo('l4')">系统设置</div> <div class="list" id="l4"></div> </div> </body> <script language="javascript"> function dodo(listid) { var dd = document.getElementById(listid); if(dd.style.display != "block") { dd.style.display="block"; } else { dd.style.display="none"; } } </script>
同一时间只允许打开一个
function dodo(listid) { var dd = document.getElementById(listid); var ll = document.getElementsByClassName("list"); for(var i=0;i<ll.length;i++) { ll[i].style.display="none"; } dd.style.display="block"; }