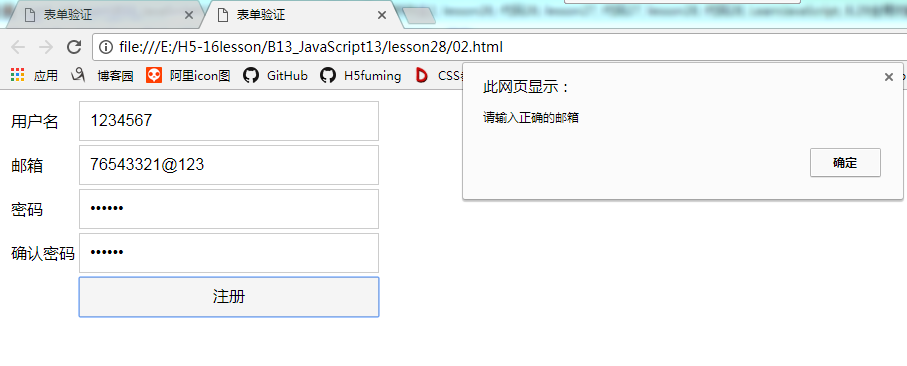
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>表单验证</title>
<style>
input,button{
box-sizing: border-box;
width:300px;
padding:10px;
font-size:16px;
line-height: 18px;
border:1px solid #ccc;
}
button{
background:#f5f5f5;
}
</style>
</head>
<body>
<form action="1.php" name="myForm" onsubmit="return checkForm()">
<table>
<tr>
<td>用户名</td>
<td>
<input type="text" name="usernameInput" autofocus tabindex="0">
</td>
</tr>
<tr>
<td>邮箱</td>
<td>
<input type="text" name="emailInput">
</td>
</tr>
<tr>
<td>密码</td>
<td>
<input type="password" name="pwdInput">
</td>
</tr>
<tr>
<td>确认密码</td>
<td>
<input type="password" name="repwdInput">
</td>
</tr>
<tr>
<td></td>
<td>
<button>注册</button>
</td>
</tr>
</table>
</form>
<script>
/**
* 表单很特殊
* 可以不通过document.getElementById获取元素
* 直接通过name属性获取元素
*/
function checkForm(){
//验证 用户名
var usernameValue = document.myForm.usernameInput.value;
if (usernameValue.search(/^\w{6,12}$/) === -1) {
alert("用户名必须是6~12位的数字、字母、下划线");
return false;
}
//验证邮箱
var emailValue = document.myForm.emailInput.value;
if (emailValue.match(/^[\w-]+@[\w-]+(\.\w+){1,3}$/) === null) {
alert("请输入正确的邮箱")
return false;
}
//验证密码
var pwdValue = document.myForm.pwdInput.value;
if (pwdValue.length < 6 || pwdValue.length > 18) {
alert("密码必须是6~18位");
return false;
}
//确认密码
var repwdValue = document.myForm.repwdInput.value;
if (repwdValue !== pwdValue) {
alert("两次密码不一致");
return false;
}
return true;
}
/**
* 邮箱格式:
* test@qq.com
user@sina.com.cn
user-name@abc.com
user_name@qq.com
*/
</script>
</body>
</html>