.NET 最好用的验证组件 FluentValidation
前言
一个 .NET 验证框架,支持链式操作,易于理解,功能完善,组件内提供十几种常用验证器,可扩展性好,支持自定义验证器,支持本地化多语言。
项目介绍
FluentValidation 是一个开源的 .NET 库,用于验证对象的属性。
它提供了一种简单而强大的方式来定义和执行验证规则,使验证逻辑的编写和维护更加直观和便捷。
相较于传统的数据注解,FluentValidation 提供了更灵活、可扩展的验证规则定义方式。
通过流畅且易于理解的语法,它显著提升了代码的可读性和可维护性。

项目使用
FluentValidation 11 支持以下平台:
.NET Core 3.1、.NET 5、.NET 6、.NET 7、.NET 8、.NET Standard 2.0
1、安装FluentValidation
通过 NuGet 包管理器或 dotnet CLI 进行安装。
dotnet add package FluentValidation
或NuGet 包管理器

2、Program.cs
using FluentValidation; using FluentValidation.AspNetCore; using MicroElements.Swashbuckle.FluentValidation.AspNetCore; var builder = WebApplication.CreateBuilder(args); var services = builder.Services; // Asp.Net stuff services.AddControllers(); services.AddEndpointsApiExplorer(); // Add Swagger services.AddSwaggerGen(); // Add FV services.AddFluentValidationAutoValidation(); services.AddFluentValidationClientsideAdapters(); // Add FV validators services.AddValidatorsFromAssemblyContaining<Program>(); // Add FV Rules to swagger services.AddFluentValidationRulesToSwagger(); var app = builder.Build(); // Use Swagger app.UseSwagger(); app.UseSwaggerUI(); app.MapControllers(); app.Run();
3、Startup.cs
public void ConfigureServices(IServiceCollection services) { // Asp.net stuff services.AddControllers(); // HttpContextValidatorRegistry requires access to HttpContext services.AddHttpContextAccessor(); // Register FV validators services.AddValidatorsFromAssemblyContaining<Startup>(lifetime: ServiceLifetime.Scoped); // Add FV to Asp.net services.AddFluentValidationAutoValidation(); // Add swagger services.AddSwaggerGen(c => { c.SwaggerDoc("v1", new OpenApiInfo { Title = "My API", Version = "v1" }); }); // [Optional] Add INameResolver (SystemTextJsonNameResolver will be registered by default) // services.AddSingleton<INameResolver, CustomNameResolver>(); // Adds FluentValidationRules staff to Swagger. (Minimal configuration) services.AddFluentValidationRulesToSwagger(); // [Optional] Configure generation options for your needs. Also can be done with services.Configure<SchemaGenerationOptions> // services.AddFluentValidationRulesToSwagger(options => // { // options.SetNotNullableIfMinLengthGreaterThenZero = true; // options.UseAllOffForMultipleRules = true; // }); // Adds logging services.AddLogging(builder => builder.AddConsole()); } public void Configure(IApplicationBuilder app, IHostingEnvironment env) { app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); // Adds swagger app.UseSwagger(); // Adds swagger UI app.UseSwaggerUI(c => { c.SwaggerEndpoint("/swagger/v1/swagger.json", "My API V1"); }); }
4、版本兼容
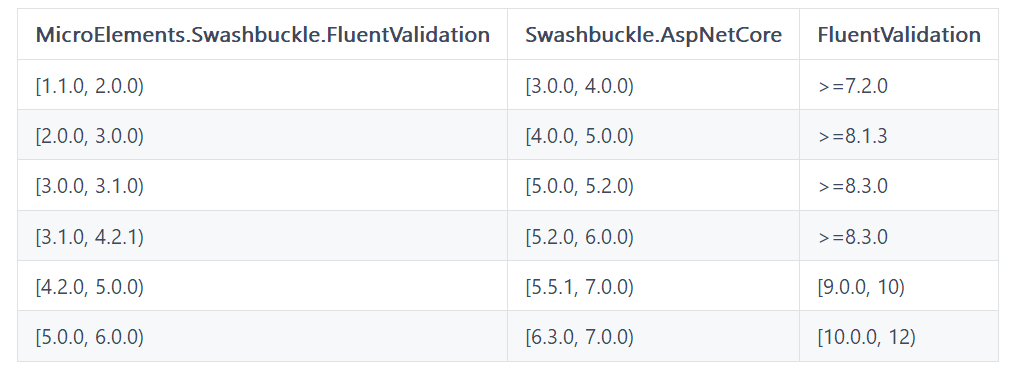
5、支持的验证器
- INotNullValidator(NotNull)
- INotEmptyValidator(NotEmpty)
- ILengthValidator(对于字符串:Length、MinimumLength、MaximumLength、ExactLength;对于数组:MinItems、MaxItems)
- IRegularExpressionValidator(Email、Matches)
- IComparisonValidator(GreaterThan、GreaterThanOrEqual、LessThan、LessThanOrEqual)
- IBetweenValidator(InclusiveBetween、ExclusiveBetween)
6、可扩展
可以将 FluentValidationRule 注册到 ServiceCollection 中。
自定义规则名称将替换具有相同名称的默认规则。
可以通过 FluentValidationRules.CreateDefaultRules() 获取默认规则的完整列表。
默认规则列表: Required(必填) NotEmpty(非空) Length(长度) Pattern(模式) Comparison(比较) Between(区间)
new FluentValidationRule("Pattern") { Matches = propertyValidator => propertyValidator is IRegularExpressionValidator, Apply = context => { var regularExpressionValidator = (IRegularExpressionValidator)context.PropertyValidator; context.Schema.Properties[context.PropertyKey].Pattern = regularExpressionValidator.Expression; } }
7、Swagger 模型和验证器
public class Sample { public string PropertyWithNoRules { get; set; } public string NotNull { get; set; } public string NotEmpty { get; set; } public string EmailAddress { get; set; } public string RegexField { get; set; } public int ValueInRange { get; set; } public int ValueInRangeExclusive { get; set; } public float ValueInRangeFloat { get; set; } public double ValueInRangeDouble { get; set; } } public class SampleValidator : AbstractValidator<Sample> { public SampleValidator() { RuleFor(sample => sample.NotNull).NotNull(); RuleFor(sample => sample.NotEmpty).NotEmpty(); RuleFor(sample => sample.EmailAddress).EmailAddress(); RuleFor(sample => sample.RegexField).Matches(@"(\d{4})-(\d{2})-(\d{2})"); RuleFor(sample => sample.ValueInRange).GreaterThanOrEqualTo(5).LessThanOrEqualTo(10); RuleFor(sample => sample.ValueInRangeExclusive).GreaterThan(5).LessThan(10); // WARNING: Swashbuckle implements minimum and maximim as int so you will loss fraction part of float and double numbers RuleFor(sample => sample.ValueInRangeFloat).InclusiveBetween(1.1f, 5.3f); RuleFor(sample => sample.ValueInRangeDouble).ExclusiveBetween(2.2, 7.5f); } }
8、包含验证器
public class CustomerValidator : AbstractValidator<Customer> { public CustomerValidator() { RuleFor(customer => customer.Surname).NotEmpty(); RuleFor(customer => customer.Forename).NotEmpty().WithMessage("Please specify a first name"); Include(new CustomerAddressValidator()); } } internal class CustomerAddressValidator : AbstractValidator<Customer> { public CustomerAddressValidator() { RuleFor(customer => customer.Address).Length(20, 250); } }
高级用法
1、异步验证
RuleForAsync(x => x.UserCode).MustAsync(async (usercode, cancellation) => { var code = await _userService.IsUserNameUniqueAsync(usercode); return code; }).WithMessage("用户编码已存在");
2、条件验证
When(x => x.IsAdmin, () => { RuleFor(x => x.Super).NotEmpty().WithMessage("管理必须是超级管理员"); });
3、自定义验证规则
RuleFor(x => x.Number).Custom((value, context) => { if (value < 10 || value > 1000) { context.AddFailure("数字必须在10 到1000之间"); } });
4、自定义错误消息
RuleFor(x => x.UserName).NotEmpty().WithMessage("用户名称不能为空") .Matches(@"^\d{6}$").WithMessage("请输入有效的6位数字用户名称");
项目地址
GitHub:https://github.com/FluentValidation/FluentValidation
总结
FluentValidation 是一个优雅且功能强大的验证库,它在提升代码可读性和可维护性的同时,保持了高度的灵活性。
无论是简单的验证需求还是复杂的业务规则,FluentValidation 都能让我们轻松确保数据的有效性。
如果大家项目中有验证需求的,可以试一试,提高开发效率。
如果你觉得这篇文章对你有帮助,不妨点个赞支持一下!你的支持是我继续分享知识的动力。如果有任何疑问或需要进一步的帮助,欢迎随时留言。
也可以加入微信公众号[DotNet技术匠] 社区,与其他热爱技术的同行一起交流心得,共同成长!优秀是一种习惯,欢迎大家留言学习!