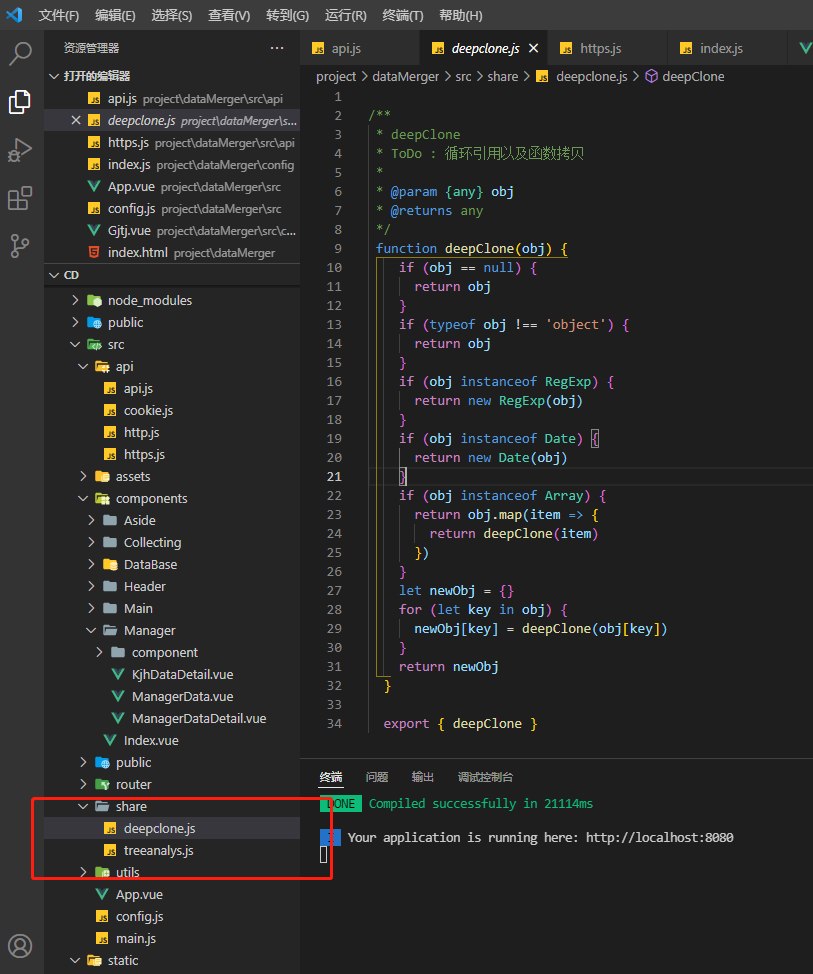

1 <template> 2 <el-tree 3 :data="datas" 4 node-key="code" 5 :highlight-current="bmhighlight" 6 @node-click="handleNodeClickbm" 7 :props="defaultProps" 8 ref="tree" 9 > 10 <span class="custom-tree-node" slot-scope="{ node, data }"> 11 <span style="font-size:0.45rem;display:flex;"> 12 {{ node.label }} 13 <span class="csse" style=""> ( {{ data.resourcecount }} ) </span> 14 </span> 15 </span> 16 </el-tree> 17 </template> 18 <script> 19 import { arrayToTree } from "../../share/treeanalys"; 20 export default{ 21 data{return{ 22 defaultProps: { 23 children: "children", 24 label: "name" 25 }, 26 datas:[] 27 } 28 } 29 methods:{ 30 getTreeData() { 31 let query = { 32 user_id: 1, 33 type: 3, 34 k_words:this.filterText 35 }; 36 getTreeList(query) 37 .then(res => { 38 // 成功之后的操作 39 console.log(res, "total"); 40 this.datas = res.data; 41 arrayToTree(this.datas); 42 const treeObj = arrayToTree(this.datas, "guid", "pguid"); 43 this.datas = treeObj.rooter.children; 44 console.log(this.datas, "this.datas"); 45 // this.datass = 46 // this.data[0].children = res.data; 47 // this.data[0].children.map((item,index)=>{ 48 // item.label = item.alias 49 // }) 50 }) 51 .catch(res => { 52 // 失败时候的操作 53 }); 54 }, 55 } 56 } 57 </script>
<template>
<el-tree
:data="datas"
node-key="code"
:highlight-current="bmhighlight"
@node-click="handleNodeClickbm"
:props="defaultProps"
ref="tree"
>
<span class="custom-tree-node" slot-scope="{ node, data }">
<span style="font-size:0.45rem;display:flex;">
{{ node.label }}
<span class="csse" style=""> ( {{ data.resourcecount }} ) </span>
</span>
</span>
</el-tree>
</template>
<script>
import { arrayToTree } from "../../share/treeanalys";
export default{
data{return{
defaultProps: {
children: "children",
label: "name"
},
datas:[]
}
methods:{
getTreeData() {
let query = {
user_id: 1,
type: 3,
k_words:this.filterText
};
getTreeList(query)
.then(res => {
// 成功之后的操作
console.log(res, "total");
this.datas = res.data;
arrayToTree(this.datas);
const treeObj = arrayToTree(this.datas, "guid", "pguid");
this.datas = treeObj.rooter.children;
console.log(this.datas, "this.datas");
// this.datass =
// this.data[0].children = res.data;
// this.data[0].children.map((item,index)=>{
// item.label = item.alias
// })
})
.catch(res => {
// 失败时候的操作
});
},
}
</script>
处理前的数据
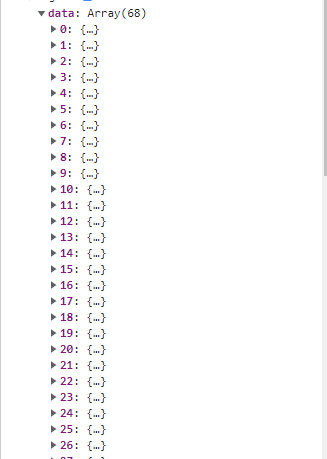
处理后的数据
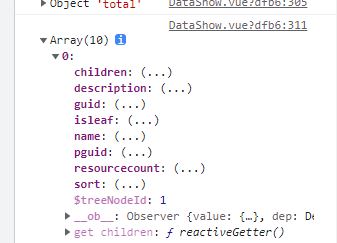
下面是两个处理js的源码
deepclone.js
/**
* deepClone
* ToDo : 循环引用以及函数拷贝
*
* @param {any} obj
* @returns any
*/
function deepClone(obj) {
if (obj == null) {
return obj
}
if (typeof obj !== 'object') {
return obj
}
if (obj instanceof RegExp) {
return new RegExp(obj)
}
if (obj instanceof Date) {
return new Date(obj)
}
if (obj instanceof Array) {
return obj.map(item => {
return deepClone(item)
})
}
let newObj = {}
for (let key in obj) {
newObj[key] = deepClone(obj[key])
}
return newObj
}
export { deepClone }
treeanalys.js
import {deepClone} from './deepclone'
/**
* 数组转换成树
*
* @param {Array} arr array
* @param {string} idField the id field
* @param {string} pidField the parent's id field
* @param {Funcction} rooterFunc the Function to judge array item is rooter node or is not
* @param {string} childrenName the name of node's children when createing tree,default 'children'
* @returns {Object{mapObj,rooter}} { mapObj, rooter }
*/
export function arrayToTree(arr, idField, pidField, rooterFunc, childrenName = 'children') {
// 克隆数组,否则当数组项为对象时,会修改原数组
let arrCloned = deepClone(arr)
// 先生成一个映射对象
const mapObj = arrCloned.reduce((total, current) => {
total[current[idField]] = current
return total
}, {})
let rooter = null
// 保存没有父节点的节点,如果没有传rooterFunc,自己创建一个根节点,把数组放进去返回根节点
const noParentNodes = []
// 循环挂载节点,返回根节点
arrCloned.forEach(element => {
if (rooterFunc && !rooter && rooterFunc(element)) {
rooter = element
}
const parentNode = mapObj[element[pidField]]
if (parentNode) {
if (!parentNode[childrenName]) {
parentNode[childrenName] = []
}
parentNode[childrenName].push(element)
} else {
noParentNodes.push(element[idField])
}
})
// 当根节点是空的时候,创建一个根节点,把没有父节点的节点放进去
if (rooter === null) {
rooter = {
[childrenName]: noParentNodes.map(noParentNodeId => {
return mapObj[noParentNodeId]
})
}
}
return { mapObj, rooter }
}