package com.example.data;
import android.support.v7.app.ActionBarActivity;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import android.content.Intent;
import android.os.Bundle;
public class MainActivity extends ActionBarActivity implements OnClickListener {
private EditText et1;
private TextView tv2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button btn=(Button)findViewById(R.id.button1);
btn.setOnClickListener(this);
et1 = (EditText) findViewById(R.id.editText1);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
// TODO Auto-generated method stub
tv2= (TextView) findViewById(R.id.textView2);
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == 1 && resultCode == 2) {
String adate = data.getStringExtra("data");
String cz = "您充值了" + adate + "元";
Toast.makeText(MainActivity.this, cz, Toast.LENGTH_LONG).show();;
}
else if (requestCode == 1 && resultCode == 3) {
String accdate = "充值失败";
Toast.makeText(MainActivity.this, accdate, Toast.LENGTH_LONG).show();
}
}
public void onClick(View v) {
Intent intent = new Intent(this,ScendActivity.class);
String phone = et1.getText().toString();
intent.putExtra("p",phone );
startActivityForResult(intent,1);
}
}
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.data.MainActivity" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_marginTop="50dp"
android:text="输入手机号码:"
android:textSize="20dp" />
<EditText
android:id="@+id/editText1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/textView1"
android:layout_alignBottom="@+id/textView1"
android:layout_toRightOf="@+id/textView1"
android:ems="10" >
<requestFocus />
</EditText>
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="164dp"
android:text="充值" />
</RelativeLayout>
package com.example.data;
import android.support.v7.app.ActionBarActivity;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.content.Intent;
import android.os.Bundle;
public class ScendActivity extends ActionBarActivity implements OnClickListener{
private TextView tv;
private EditText et2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.scend);
Button bt2= (Button) findViewById(R.id.button2);
Button bt3=(Button) findViewById(R.id.button3);
et2=(EditText)findViewById(R.id.editText2);
// TODO Auto-generated method stub
final Intent intent = getIntent();
Bundle bundle=intent.getExtras();//.getExtras()得到intent所附带的额外数据
String p=bundle.getString("p");//getString()返回指定key的值
tv=(TextView) findViewById(R.id.textView3);
tv.setText(p);
bt2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
String Data=et2.getText().toString();
Intent intent=new Intent();
intent.putExtra("data", Data);
setResult(2,intent);
finish();
}
});
bt3.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
Intent intent=new Intent();
setResult(3,intent);
finish();
}
});
}
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
}
}
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.data.ScendActivity" >
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBottom="@+id/textView1"
android:layout_alignTop="@+id/textView1"
android:layout_centerHorizontal="true"
android:text=" " />
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/button2"
android:layout_alignBottom="@+id/button2"
android:layout_toRightOf="@+id/textView3"
android:text="取消充值" />
<TextView
android:id="@+id/textView4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignRight="@+id/button3"
android:layout_alignTop="@+id/textView3"
android:text="充值"
android:textSize="20dp" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignRight="@+id/textView5"
android:layout_below="@+id/editText2"
android:layout_marginTop="97dp"
android:text="充值" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@+id/textView5"
android:layout_alignLeft="@+id/textView5"
android:text="你要为:"
android:textSize="20dp" />
<EditText
android:id="@+id/editText2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignTop="@+id/textView5"
android:layout_toRightOf="@+id/textView5"
android:ems="10" />
<TextView
android:id="@+id/textView5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_below="@+id/textView4"
android:layout_marginTop="74dp"
android:text="充值金额:"
android:textSize="20dp" />
</RelativeLayout>
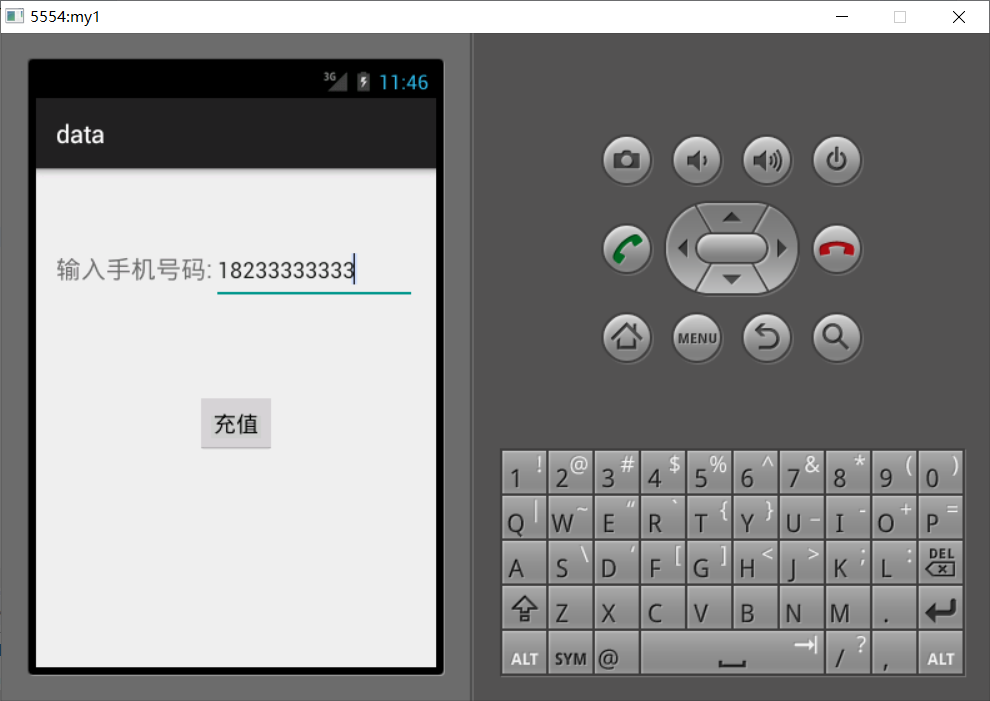
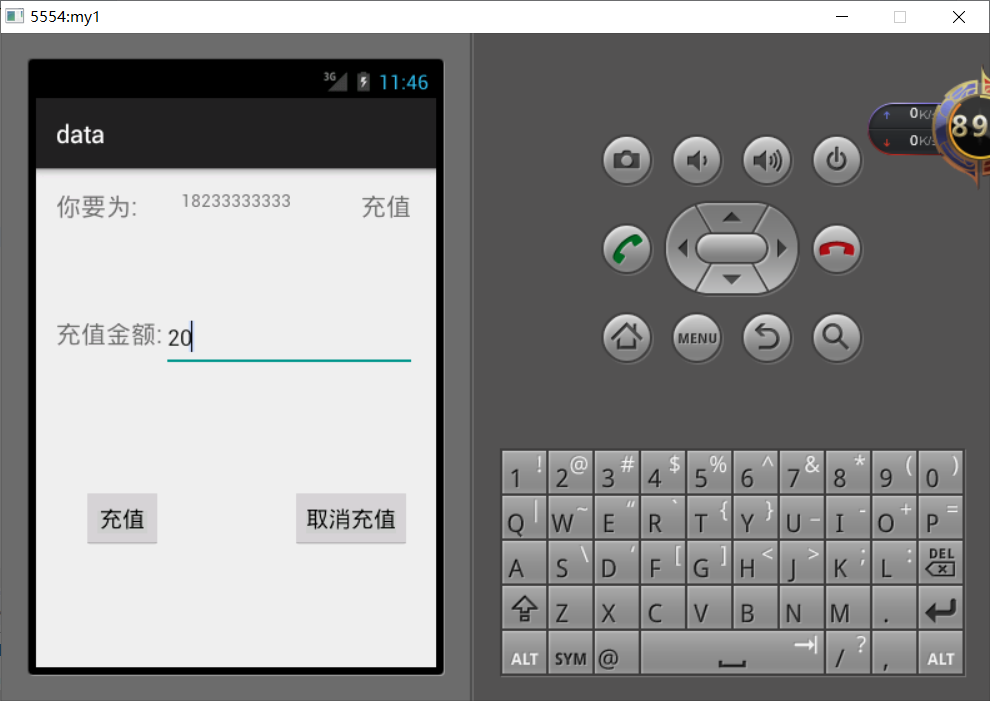
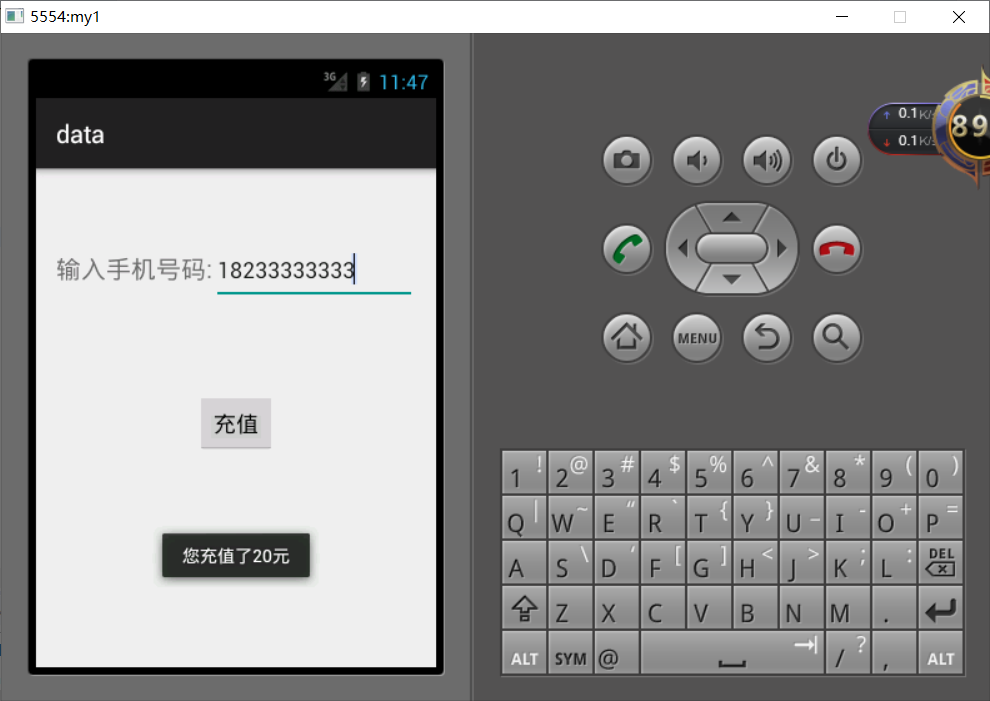
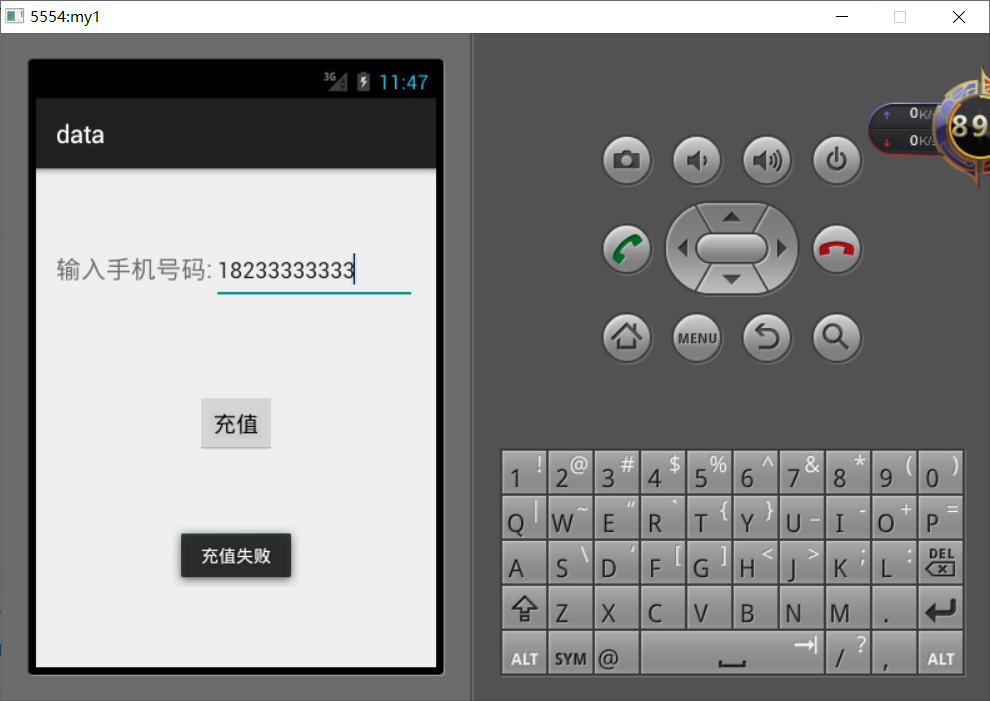