实验三
实验任务一
#include <stdio.h> #include <stdlib.h> #include <time.h> #include <windows.h> #define N 80 void print_text(int line, int col, char text[]); void print_spaces(int n); void print_blank_lines(int n); int main() { int line, col, i; char text[N] = "hi, November~"; srand(time(0)); for(i = 1; i <= 10; ++i) { line = rand() % 25; col = rand() % 80; print_text(line, col, text); Sleep(1000); // } return 0; } void print_spaces(int n) { int i; for(i = 1; i <= n; ++i) printf(" "); } void print_blank_lines(int n) { int i; for(i = 1; i <= n; ++i) printf("\n"); } void print_text(int line, int col, char text[]) { print_blank_lines(line-1); print_spaces(col-1); printf("%s", text); }
随机出现 hi,Noveber
实验任务二
#include<stdio.h> #include<stdlib.h> long long fac(int ); int main(){ int i,n; printf("Enter n :"); scanf("%d",&n); for(i=1;i<=n;++i) printf("%d!=%lld\n",i,fac(i)); system("pause"); return 0; } long long fac(int n) {static long long p=1; p=p*n; return p; }
#include<stdio.h> #include<stdlib.h> int func(int, int); int main() { int k = 4, m = 1, p1, p2; p1 = func(k, m); p2 = func(k, m); printf("%d, %d\n", p1, p2); system("pause"); return 0; } int func(int a, int b) { static int m = 0, i = 2; i += m + 1; m = i + a + b; return m; }
一致
实验任务三
#include <stdio.h> #include<stdlib.h> long long func(int n); int main() { int n; long long f; while (scanf("%d", &n) != EOF) { f = func(n); printf("n = %d, f = %lld\n", n, f); } system("pause"); return 0; } long long func(int n){ int i; if(n==1) return 1; else return 2*func(n-1)+1; }
实验任务四
#include <stdio.h> #include<stdlib.h> int func(int n, int m); int fac(int ); int main() { int n, m; while(scanf("%d%d", &n, &m) != EOF) printf("n = %d, m = %d, ans = %d\n", n, m, func(n, m)); system("pause"); return 0; } int fac(int n) { int p=1;int i; if(n>=1){ for(i=1;i<=n;++i) p=p*i;} if(n==0) p=1; return p; } int func(int n,int m){ return fac(n)/(fac(m)*fac(n-m)); }
#include <stdio.h>
#include<stdlib.h>
int func(int n, int m);
#include<stdlib.h>
int func(int n, int m);
int main() {
int n, m;
int n, m;
while(scanf("%d%d", &n, &m) != EOF)
printf("n = %d, m = %d, ans = %d\n", n, m, func(n, m));
system("pause");
return 0;
}
printf("n = %d, m = %d, ans = %d\n", n, m, func(n, m));
system("pause");
return 0;
}
int func(int n,int m){
if (n==m) return 1;
else if(m==0)
if (n==m) return 1;
else if(m==0)
return 1;
else if(n<m)
return 0;
else if(n<m)
return 0;
return func(n-1,m)+func(n-1,m-1);
}
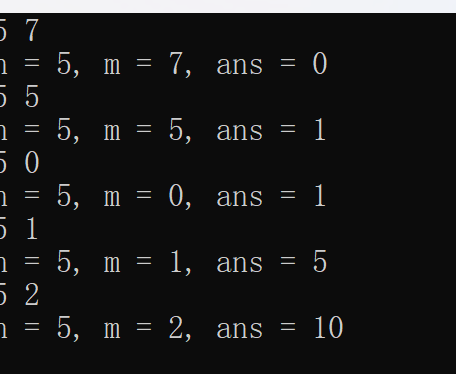
实验任务五
#include <stdio.h> #include<stdlib.h> int s=0; void move(char,char); void hn(int ,char,char ,char); int main() { int n; char A,B,C; while(scanf("%d %c %c %c",&n,&A,&B,&C)!=EOF){ hn(n,A,B,C); printf("一共移动%d次",s); s=0; } system("pause"); return 0; } void move (char a,char b){ printf("%c-->%c\n",a,b); ++s; } void hn(int n,char b,char m,char e){ if(n==1) move(b,e); else { hn(n-1,b,e,m); move(b,e); hn(n-1,m,b,e); } }
实验任务六
#include <stdio.h>
#include<stdlib.h>
long func(long s);
int main() {
long s,t;
printf("Enter a number:");
while(scanf("%ld",&s)!=EOF){
t=func(s);
printf("new number is :%ld\n\n",t);
printf("Enter a number:");}
system("pause");
return 0;
}
long func(long s){
int b,i,k=-1;
double m=0;
do{
b=s%10;
if(b%2!=0) {m=m*0.1+b;++k;}
s=s/10;
}while(s!=0);
for(i=1;i<=k;++i)
m=m*10;
return m;
}
#include <stdio.h> #include<stdlib.h> long long func(long s); int main() { long s,t; printf("Enter a number:"); while(scanf("%lld",&s)!=EOF){ t=func(s); printf("new number is :%ld\n\n",t); printf("Enter a number:");} system("pause"); return 0; } long long func(long s){ int b,i,k=-1; double m=0; do{ b=s%10; if(b%2!=0) {m=m*0.1+b;++k;} s=s/10; }while(s!=0); for(i=1;i<=k;++i) m=m*10; return m; }