求二叉搜索树的下一个节点(中序遍历的下一个节点)
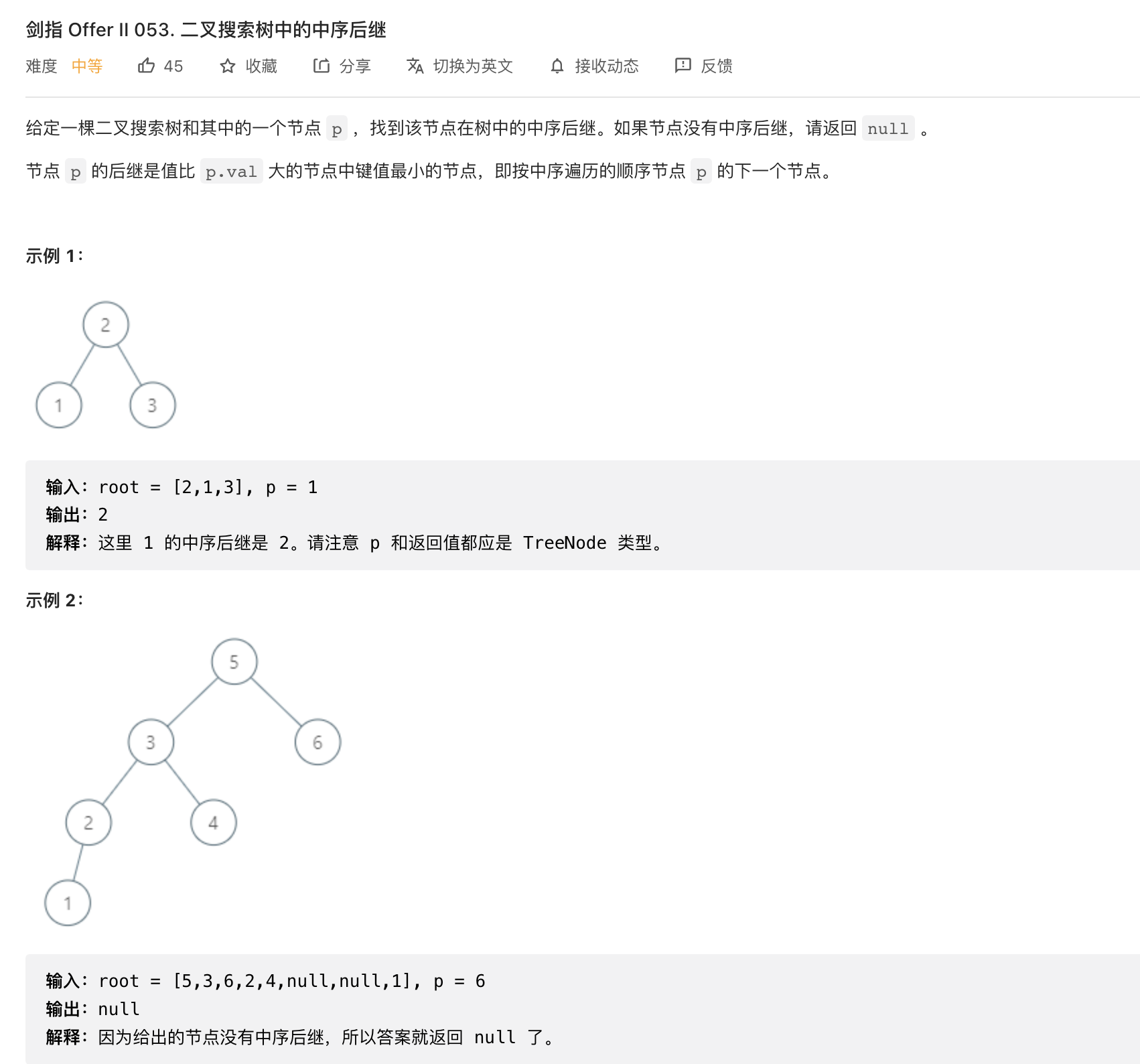
https://leetcode.cn/problems/P5rCT8/
/** * Definition for a binary tree node. * type TreeNode struct { * Val int * Left *TreeNode * Right *TreeNode * } */ func inorderSuccessor(root *TreeNode, p *TreeNode) *TreeNode { stack := []*TreeNode{} var pre *TreeNode cur:=root for len(stack) > 0 ||cur!=nil{ for cur != nil {//用栈遍历二叉树 stack = append(stack, cur) cur = cur.Left } cnt:=len(stack)-1 cur = stack[cnt] //出栈 stack = stack[:cnt] if pre == p { return cur } pre = cur cur = cur.Right } return nil } //建一棵树 func insert(root *TreeNode,x int){ if root==nil{ tmp:=&TreeNode{ Val:x, } return tmp } if x<=root.Val{//这里是按照中序遍历的方式建立二叉树,如果要层序遍历的方式建立二叉树的话,需要根据索引的奇偶做判断 root.Left=insert(root.Left,x) }else{ root.Right=insert(root.Right,x) } return root }
二叉树的建立:https://www.cnblogs.com/-citywall123/p/16434923.html
等风起的那一天,我已准备好一切