#include <iostream> #include "graph.h" using namespace std; int main() { Graph graph1('*',5), graph2('$',7) ; // 定义Graph类对象graph1, graph2 graph1.draw(); // 通过对象graph1调用公共接口draw()在屏幕上绘制图形 graph2.draw(); // 通过对象graph2调用公共接口draw()在屏幕上绘制图形 graph1.setcolor('y','g'); graph1.draw(); graph2.setcolor('r','w'); graph2.draw(); return 0; }
// 类graph的实现 #include "graph.h" #include <iostream> #include <windows.h> using namespace std; // 带参数的构造函数的实现 Graph::Graph(char ch, int n): symbol(ch), size(n) { } void Graph::draw(){ int i,j; for(i=0;i<size;i++){ for(j=1;j<=2*size-1;j++){ if(j>=size-i&&j<=size+i) cout<<symbol; else cout<<' '; } cout<<endl; } SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE),(forecolor)|(backcolor)); }; int Graph::str(char a){ switch(a){ case 'b':return 0;break; case 'g':return 2;break; case 'r':return 4;break; case 'p':return 5;break; case 'y':return 6;break; case 'w':return 7;break; } } void Graph::setcolor(char fore,char back){ forecolor=str(fore); backcolor=str(back); } // 成员函数draw()的实现 // 功能:绘制size行,显示字符为symbol的指定图形样式 // size和symbol是类Graph的私有成员数据 // 补足代码,实现「实验4.pdf」文档中展示的图形样式
// 类Graph的声明 class Graph { public: Graph(char ch, int n); // 带有参数的构造函数 void draw(); // 绘制图形 void setcolor(char fore,char back); private: char symbol; int size; unsigned forecolor,backcolor; int str(char a); };
#include"Fraction.h" #include<iostream> #include<cmath> using namespace std; int Fraction::gct(int a,int b){ return a%b==0?b:gct(b,a%b); } Fraction::Fraction():top(0),bottom(1){ } Fraction::Fraction(int t):top(t),bottom(1){ } Fraction::Fraction(int t,int b):top(t),bottom(b){ } void Fraction::add(Fraction &p){ top=top*p.bottom+p.top*bottom; bottom=bottom*p.bottom; form(); op(); } void Fraction::sub(Fraction &p){ top=top*p.bottom-p.top*bottom; bottom=bottom*p.bottom; form(); op(); } void Fraction::mul(Fraction &p){ top=top*p.top; bottom=bottom*p.bottom; form(); op(); } void Fraction::div(Fraction &p){ top=top*p.bottom; bottom=p.top*bottom; form(); op(); } void Fraction::form(){ if(bottom<0){ bottom*=-1; top*=-1; } int g=gct(abs(top),abs(bottom)); top/=g; bottom/=g; } void Fraction::ip(){ cout<<"输入分子"<<endl; cin>>top; int bo; cout<<"输入分母"<<endl; cin>>bo; while(bo==0){ cout<<"请重新输入"<<endl; cin>>bo; } bottom=bo; } void Fraction::op(){ form(); if(bottom!=1) cout<<top<<"/"<<bottom<<endl; else cout<<top<<endl; }
#include"Fraction.h" #include<iostream> #include<cmath> using namespace std; int main(){ Fraction a; Fraction b(3,4); Fraction c(5); cout<<"输出a的初值"<<endl; a.op(); cout<<"输入a"<<endl; a.ip(); cout<<"a的新值"<<endl; a.op(); cout<<"输出b,c"<<endl; b.op(); c.op(); cout<<"b,c的加减乘除"<<endl; b.add(c); b.sub(c); b.mul(c); b.div(c); return 0; }
class Fraction { private: int top; int bottom; int gct(int a,int b); public: Fraction(); Fraction(int t); Fraction(int t,int b); void add(Fraction &p); void sub(Fraction &p); void mul(Fraction &p); void div(Fraction &p); void for
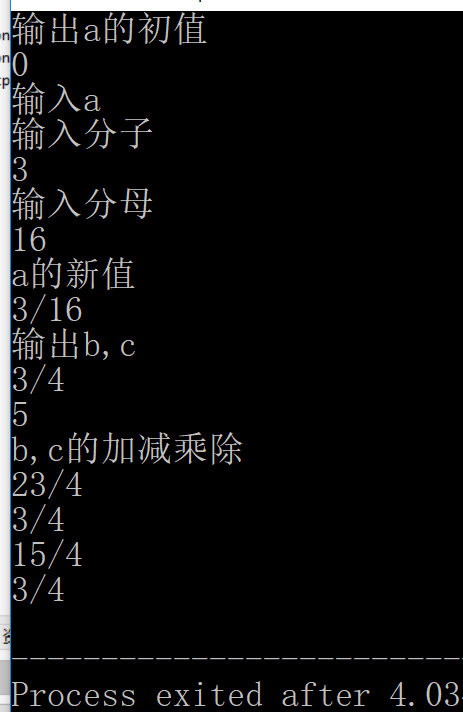
m(); void ip(); void op(); };