二叉树遍历
调试环境遇到点问题,还是不明觉厉。。。
题目描述
编一个程序,读入用户输入的一串先序遍历字符串,根据此字符串建立一个二叉树(以指针方式存储)。 例如如下的先序遍历字符串: ABC##DE#G##F### 其中“#”表示的是空格,空格字符代表空树。建立起此二叉树以后,再对二叉树进行中序遍历,输出遍历结果。
输入描述:
输入包括1行字符串,长度不超过100。
输出描述:
可能有多组测试数据,对于每组数据,
输出将输入字符串建立二叉树后中序遍历的序列,每个字符后面都有一个空格。
每个输出结果占一行。
输入例子:
abc##de#g##f###
输出例子:
c b e g d f a
先上网页的测试环境运行成功的代码:
import java.util.Scanner; public class Main { private static int index = 0; public static void main(String[] args) { Scanner in = new Scanner(System.in); while (in.hasNext()) { String str = in.next(); Node tree = rebuildTree(str); inOrderTraverse(tree); System.out.println(); } in.close(); } /** * 内部节点类 */ private static class Node { private Node left; private Node right; private char data; public Node(char data) { this.left = null; this.right = null; this.data = data; } } private static Node rebuildTree(String nlr){ int []index = new int[]{0};// 模拟指针,递归过程中都是对index[0]进行修改;也可用全局变量实现 return createTree(nlr,index); } /** * 根据先序序列构建二叉树,递归 * @param str * @return */ public static Node createTree(String str,int[] index) { //if(index<=str.length()){ char ch = str.charAt(index[0]); //System.out.println(ch); if (ch == '#') return null; else { Node root = new Node(ch); index[0]++; root.left = createTree(str,index); index[0]++; root.right = createTree(str,index); //System.out.println(index); return root; } //}else // return null; } /** * 二叉树中序遍历,递归 */ private static void inOrderTraverse(Node root) { if (root == null) return; inOrderTraverse(root.left); System.out.print(root.data + " "); inOrderTraverse(root.right); } }
然后是eclipse中的成功的代码 但就是不能再网页里编译成功。。。
package test726; import java.util.Scanner; public class 二叉树遍历 { private static int index = 0; public static void main(String[] args) { Scanner in = new Scanner(System.in); while (in.hasNext()) { String str = in.next(); Node tree = createTree(str); inOrderTraverse(tree); System.out.println(); } in.close(); } /** * 内部节点类 */ private static class Node { private Node left; private Node right; private char data; public Node(char data) { this.left = null; this.right = null; this.data = data; } } /** * 根据先序序列构建二叉树,递归 * @param str * @return */ public static Node createTree(String str) { if(index<str.length()){ char ch = str.charAt(index); //System.out.println(ch); if (ch == '#') return null; else { Node root = new Node(ch); index++; root.left = createTree(str); index++; root.right = createTree(str); //System.out.println(index); return root; } }else return null; } /** * 二叉树中序遍历,递归 */ private static void inOrderTraverse(Node root) { if (root == null) return; inOrderTraverse(root.left); System.out.print(root.data + " "); inOrderTraverse(root.right); } }
最后还是发现是自己犯了蠢,index在操作完一组数据后没有清零,导致了输出的错误。
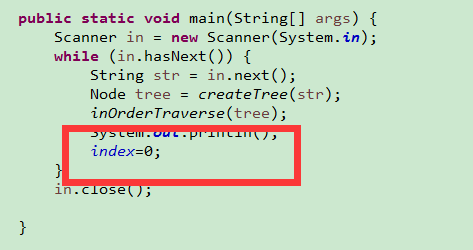
之后调试成功。。加上这句就行。
再接再厉!
心有猛虎,细嗅蔷薇 转载请注明:https://www.cnblogs.com/ygh1229/