python学习笔记(九) glob模块、单元测试框架、单元测试生成报告、单元测试生成漂亮报告、parameterized循环遍历单元测试方法、查找测试用例
1.glob模块
#glob文件查找模块,返回的文件名只包括当前目录里的文件名,不包括子文件夹里的文件 import glob g = glob.glob('*.py')#获取当前目录下以*.py结尾的文件 for filename in g: print(filename) 测试结果: 单元测试框架.py 单元测试框架2.py
g = glob.glob('/Users/wangxiaoyu/PycharmProjects/python_automated_development/day10/*.py')#获取指定目录下以*.py结尾的文件 for filename in g: print(filename) 测试结果: /Users/wangxiaoyu/PycharmProjects/python_automated_development/day10/单元测试框架.py /Users/wangxiaoyu/PycharmProjects/python_automated_development/day10/单元测试框架2.py
2.单元测试框架(运行方式,Run->Run...)
import unittest class ClaTestCase(unittest.TestCase):#1.类继承了unittest.TestCase那么这个类就是测试类 def test_atest1(self):#2.方法必须以test开头,否则不运行; print('1') def test_etest1(self):#3.类中每个方法执行顺序:按方法名称test_后面首字母排序顺序执行 print('2') def test_dtest1(self): print('3') def ftest1(self):#4.不带test的方法,在单元测试类调用的时候不会执行 print('haha') unittest.main() 测试结果:
import unittest def sum(a,b): return a/b class ClaTestCase(unittest.TestCase): def test_sum1(self): '''测试sum方法正常返回值情况''' result = sum(9,3) self.assertEqual(3.0,result) def test_sum2(self): '''测试sum方法返回结果与对比结果不一致情况''' result = sum(9, 3) self.assertEqual(3.1,result,'期望结果是3.0') unittest.main() 测试结果:
import unittest class ClaTestCase(unittest.TestCase): @classmethod def setUpClass(cls): print('setUpClass,所有方法执行前执行一次') @classmethod def tearDownClass(cls): print('tearDownClass,所有方法执行后执行一次') def setUp(self): print('setUp,每个方法执行前执行一次') def tearDown(self): print('tearDown,每个方法执行结束后执行一次') def test_sayHello(self): print('Hello1!') def test_buy(self): print('buy!') def test_eat(self): print('eat!') unittest.main() 测试结果:
3.单元测试生成报告
import traceback import unittest import requests import HTMLTestRunner import nnlog log = nnlog.Logger('login.log') class LoginCase(unittest.TestCase): def test_login1(self): '''登录接口--正常登录''' url = 'http://api.nnzhp.cn/api/user/login' d = {'username':'wangxiaoyu','passwd':'Yu9251314'} result = requests.post(url,data=d).text self.assertIn('userId',result,'没有返回userId') def test_login2(self): '''登录接口--密码为空''' url = 'http://api.nnzhp.cn/api/user/login' d = {'username':'wangxiaoyu','passwd':''} result = requests.post(url,data=d).text self.assertIn('userId',result,'没有返回userId') suite = unittest.makeSuite(LoginCase)#把测试用例假如到集合中 with open('test_report.html','wb') as fw: runner = HTMLTestRunner.HTMLTestRunner(fw,title='登录模块测试',description='登录模块测试情况如下') runner.run(suite) fw.close() 测试结果: .F<_io.TextIOWrapper name='<stderr>' mode='w' encoding='UTF-8'> Time Elapsed: 0:00:00.359809
查看生成的报告:test_report.html
...
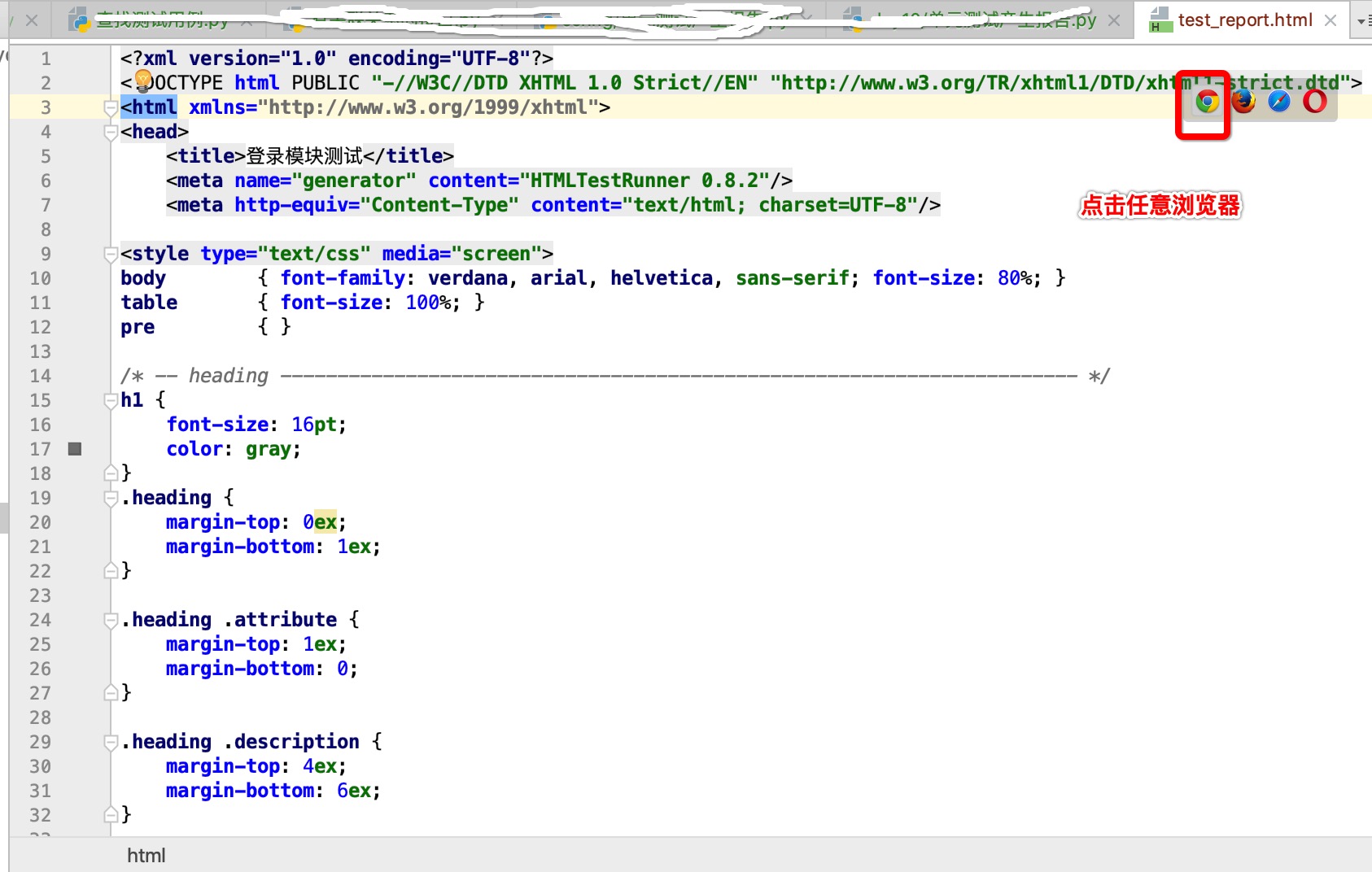
在浏览器中查看
……
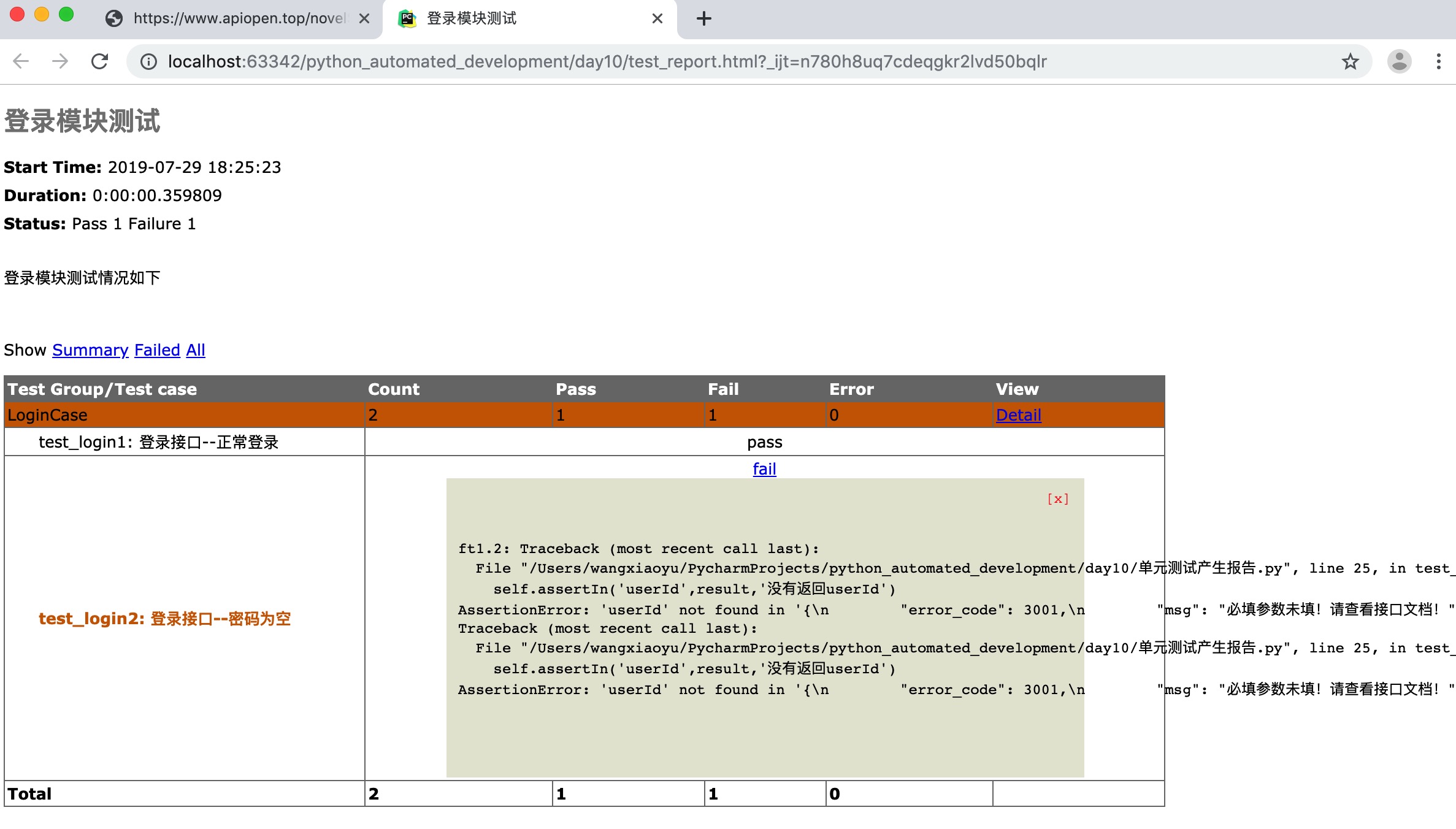
4.单元测试生成漂亮报告
import unittest from BeautifulReport import BeautifulReport import requests class LoginCase(unittest.TestCase): def test_login1(self): '''登录接口--正常登录''' url = 'http://api.nnzhp.cn/api/user/login' d = {'username':'wangxiaoyu','passwd':'Yu9251314'} result = requests.post(url,data=d).text self.assertIn('userId',result,'没有返回userId') def test_login2(self): '''登录接口--密码为空''' url = 'http://api.nnzhp.cn/api/user/login' d = {'username':'wangxiaoyu','passwd':''} result = requests.post(url,data=d).text self.assertIn('userId',result,'没有返回userId') suite = unittest.makeSuite(LoginCase)#把测试用例假如到集合中 bf = BeautifulReport(suite) bf.report(description='登录用例测试详情:',filename='beautiful_report.html') 测试结果: .F 测试已全部完成, 可前往/Users/wangxiaoyu/PycharmProjects/python_automated_development/day10查询测试报告
查看生成的报告beautiful_report.html
……
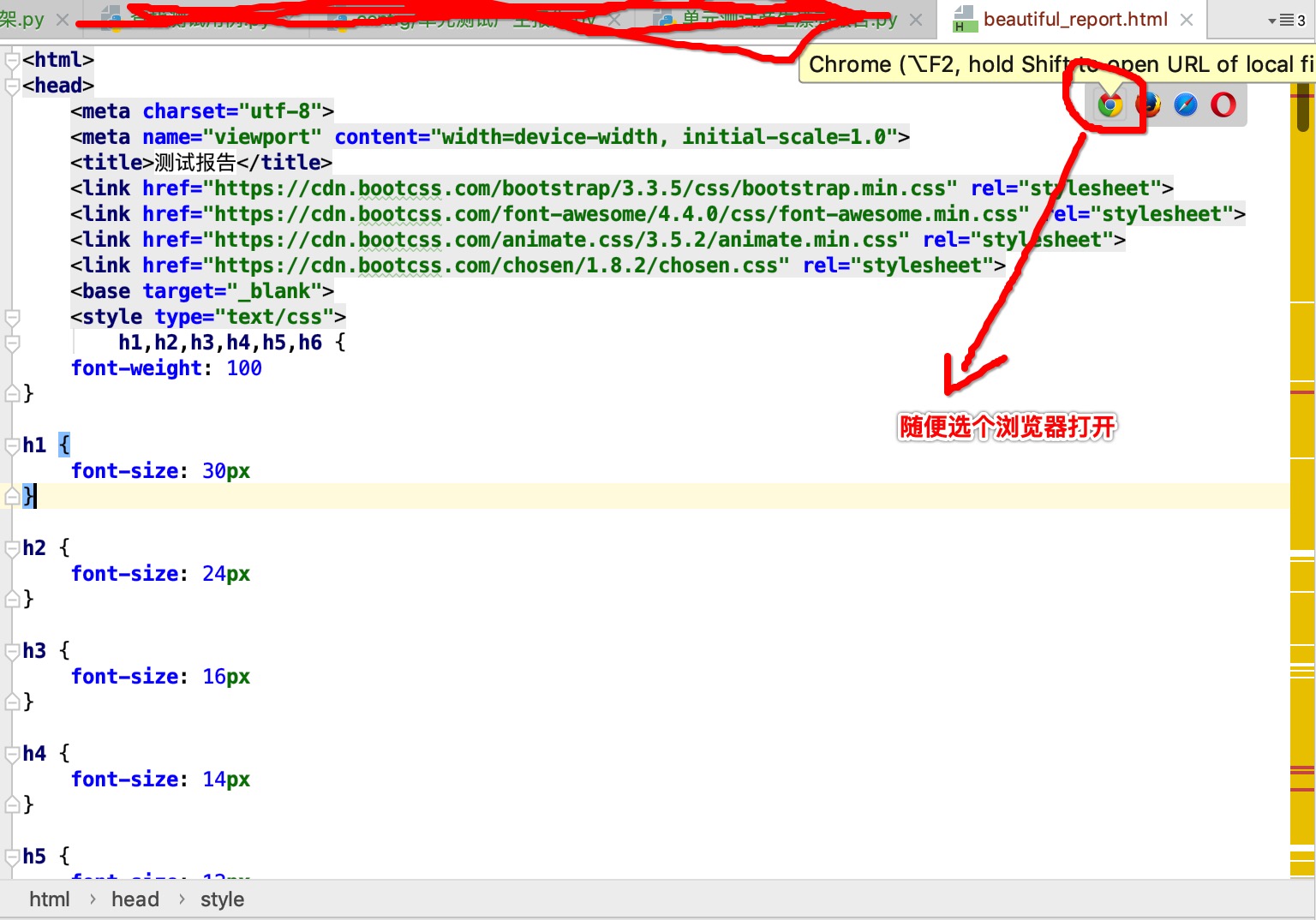
在浏览器中查看效果
……
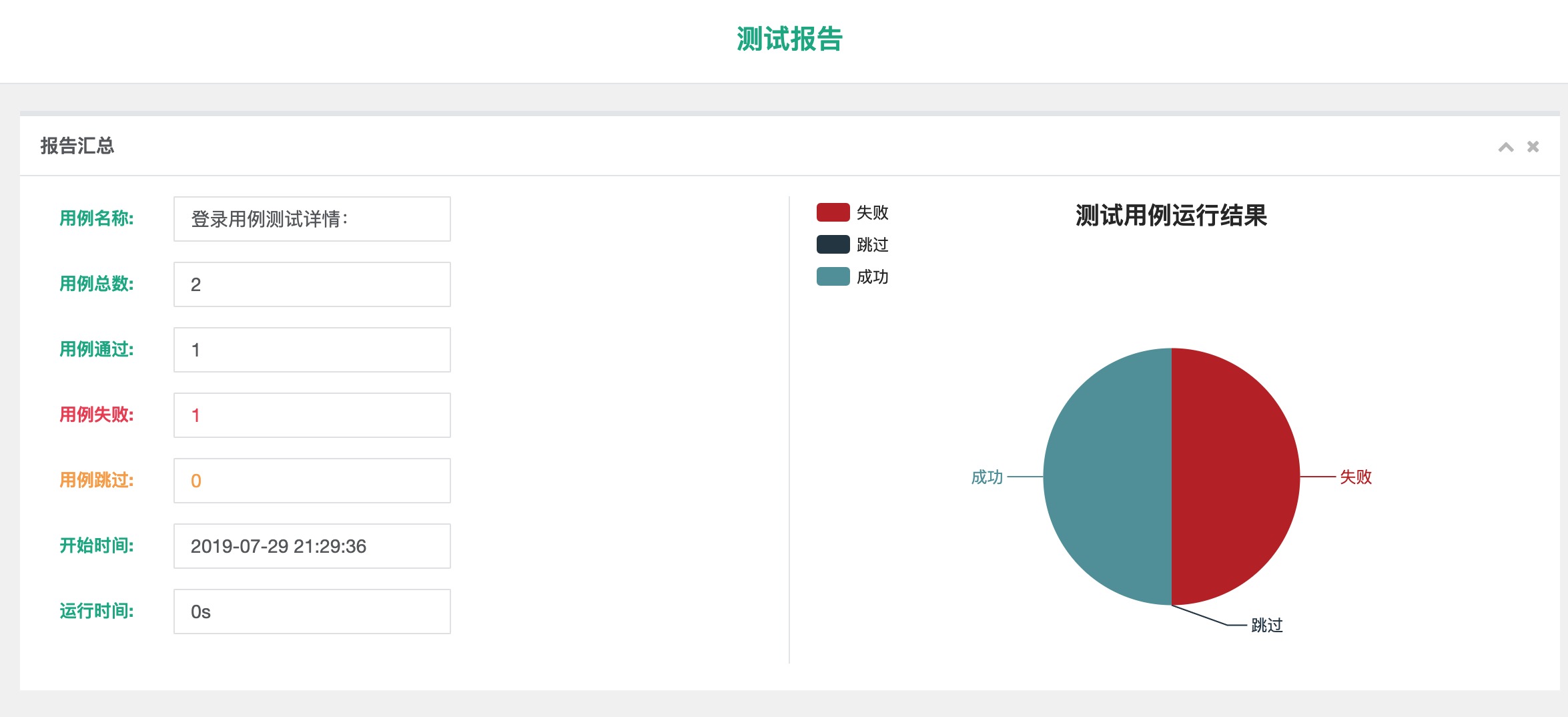
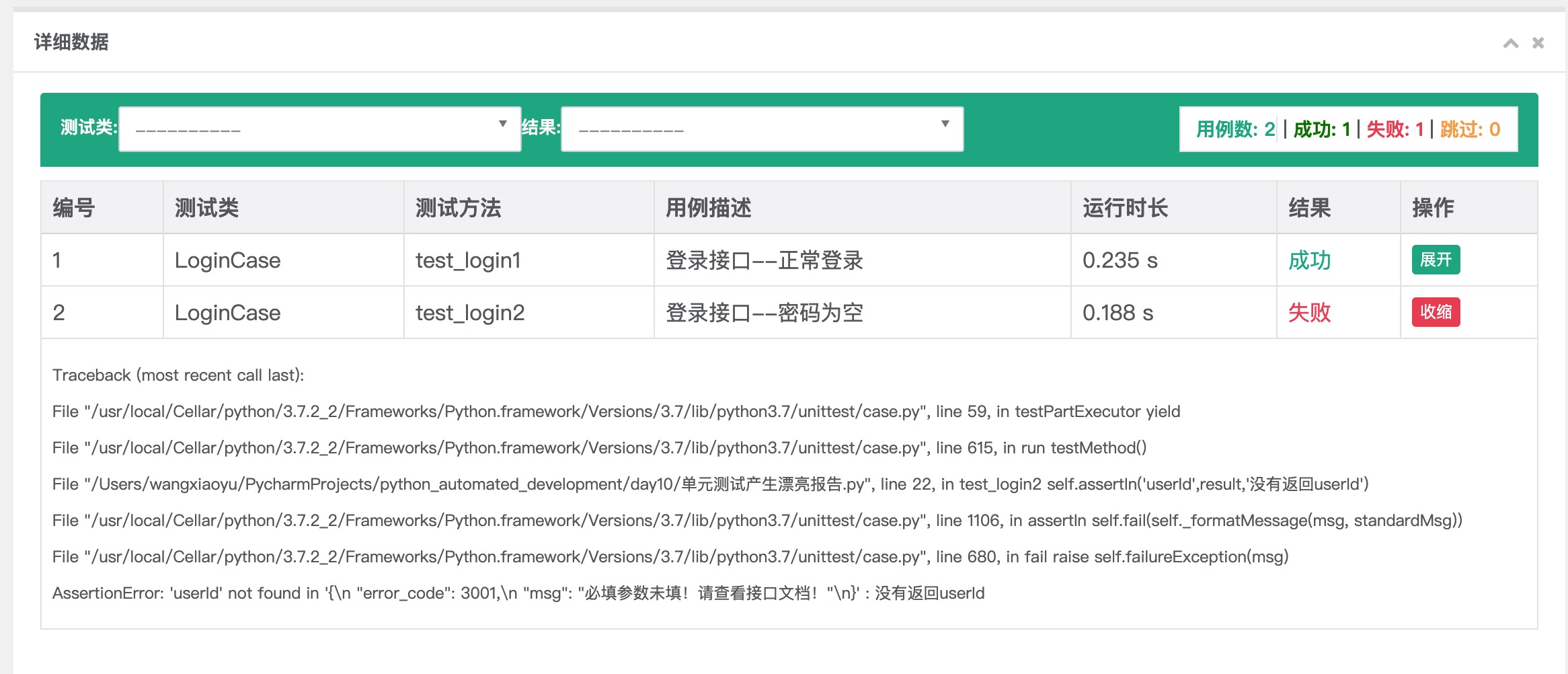
5.parameterized循环遍历单元测试方法
方法1:
import unittest from BeautifulReport import BeautifulReport import requests from parameterized import parameterized class LoginCase(unittest.TestCase): @parameterized.expand([ ['wangxiaoyu','123456','用户名/密码错误!'], ['wangxiaoyu','Yu9251314','userId'], ['wangxiaoyu','','必填参数未填!请查看接口文档!'], ['','Yu9251314','必填参数未填!请查看接口文档!'], ['wangxiaoyu1','Yu9251314','用户名/密码错误!'], ]) def test_login(self,username,passwod,success_flag): '''登录接口--''' url = 'http://api.nnzhp.cn/api/user/login' d = {'username':username,'passwd':passwod} result = requests.post(url,data=d).text self.assertIn(success_flag,result,'登录失败!') suite = unittest.makeSuite(LoginCase)#把测试用例假如到集合中 bf = BeautifulReport(suite) bf.report(description='登录用例测试详情:',filename='beautiful_report.html') 测试结果:
/usr/local/bin/python3 /Users/wangxiaoyu/PycharmProjects/python_automated_development/day10/单元测试产生漂亮报告.py
.....
测试已全部完成, 可前往/Users/wangxiaoyu/PycharmProjects/python_automated_development/day10查询测试报告
浏览器查看beautiful_report.html
……
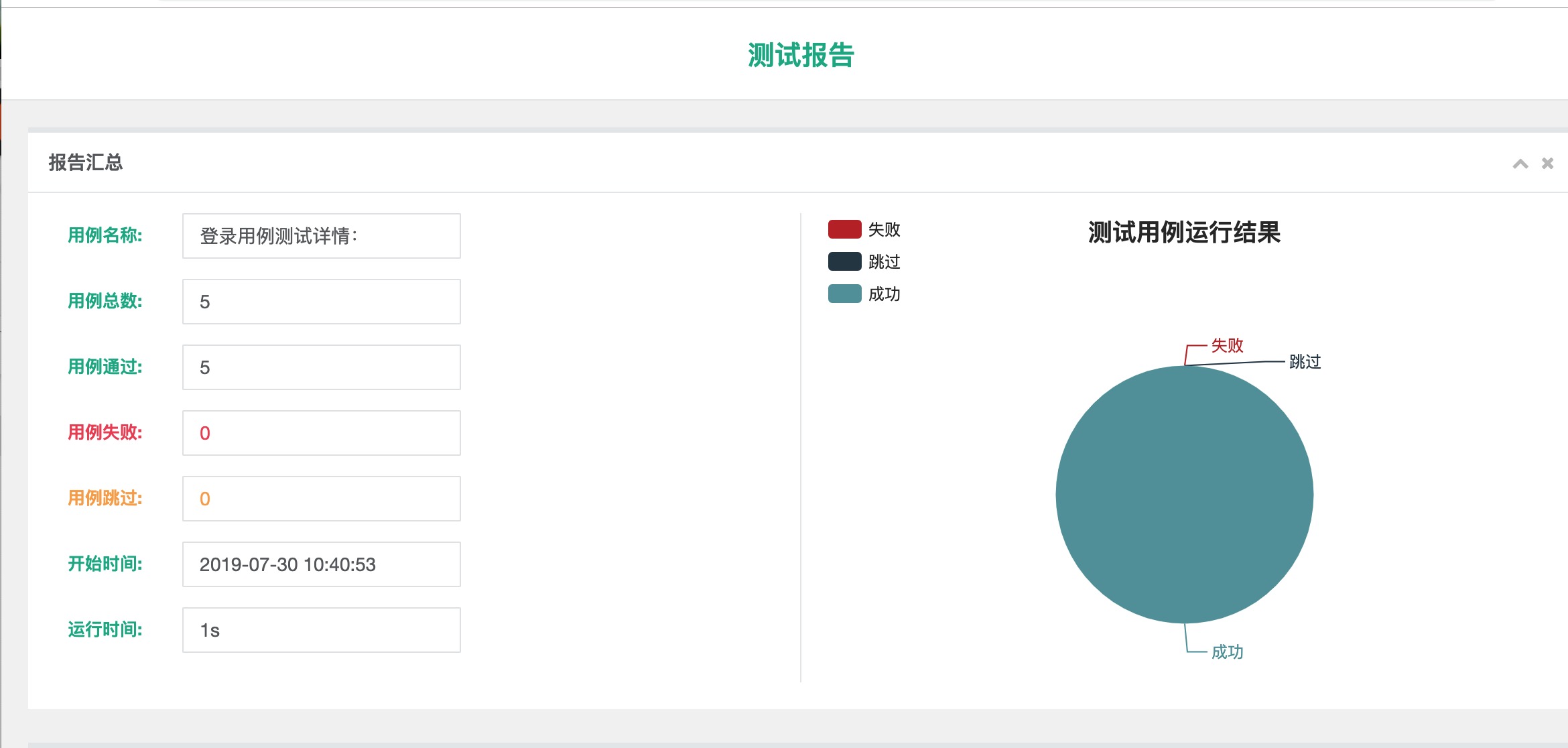
方法2(从文件里面读,当然还可以从excel,db等,这里就不一一列举了):
import unittest from BeautifulReport import BeautifulReport import requests from parameterized import parameterized def get_data(filename): ''' 获取文件中的数据 :param filename: :return: 返回格式[['','',''],['','',''],['','','']] ''' list = [] with open(filename,encoding='utf-8') as f: for i in f: line = i.strip() if line: list.append(line.split(',')) return list class LoginCase(unittest.TestCase): @parameterized.expand(get_data('user')) def test_login(self,username,passwod,success_flag): '''登录接口''' url = 'http://api.nnzhp.cn/api/user/login' d = {'username':username,'passwd':passwod} result = requests.post(url,data=d).text self.assertIn(success_flag,result,'登录失败!') suite = unittest.makeSuite(LoginCase)#把测试用例假如到集合中 bf = BeautifulReport(suite) bf.report(description='登录用例测试详情:',filename='beautiful_report.html') 测试结果: F.F 测试已全部完成, 可前往/Users/wangxiaoyu/PycharmProjects/python_automated_development/day10查询测试报告
user中的内容:
浏览器中查看beautiful_report.html
……
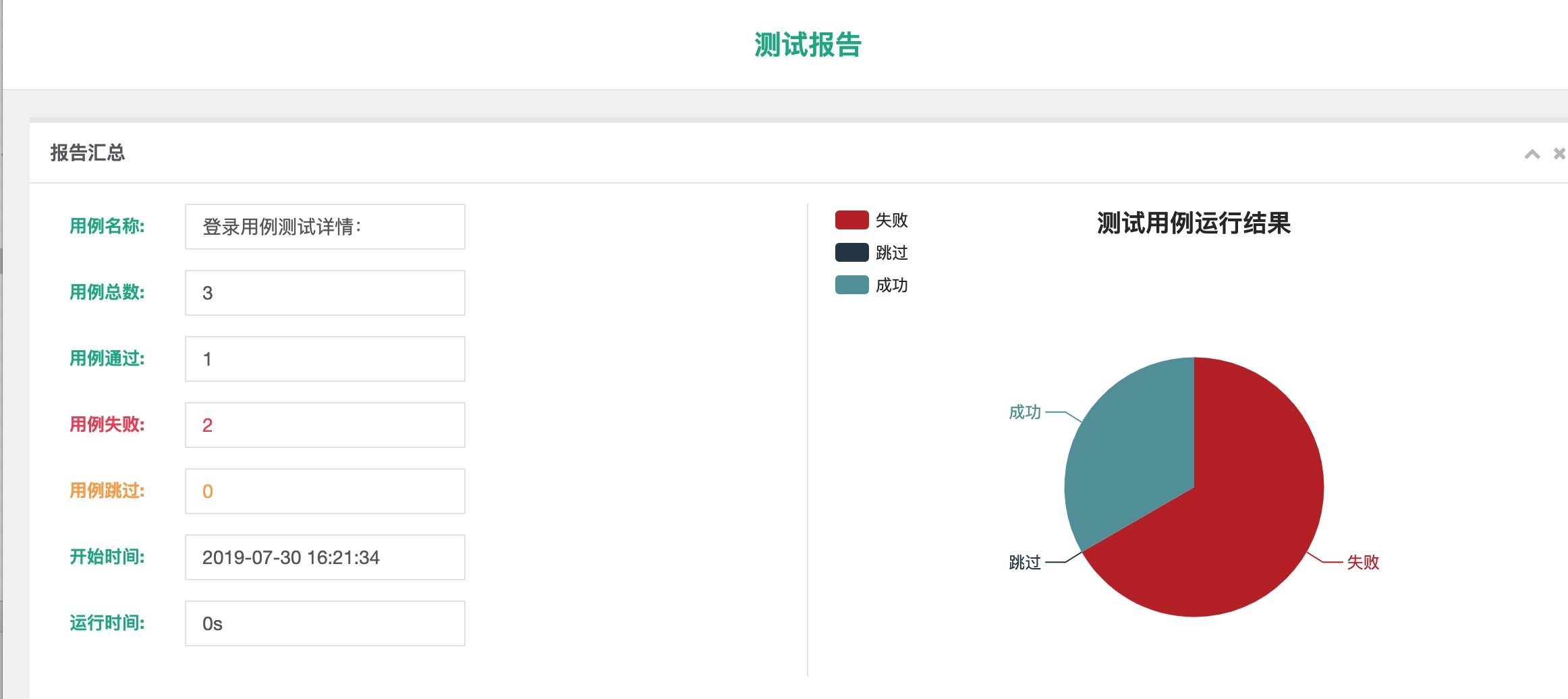
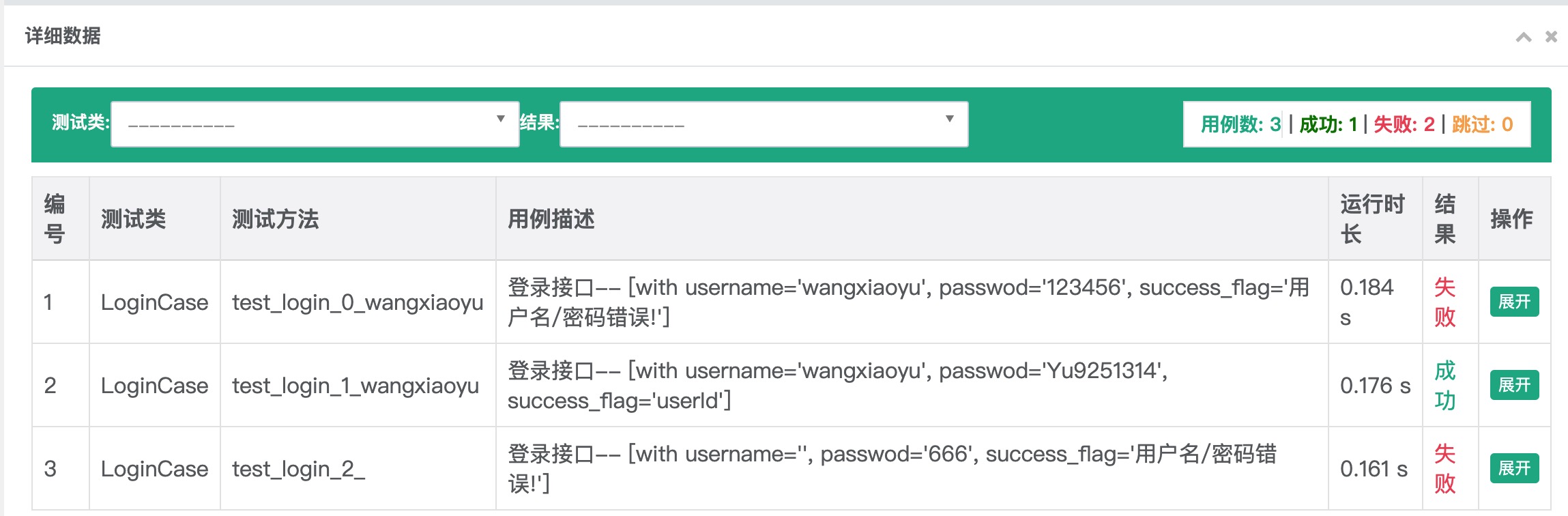
6.查找测试用例
a.代码结构
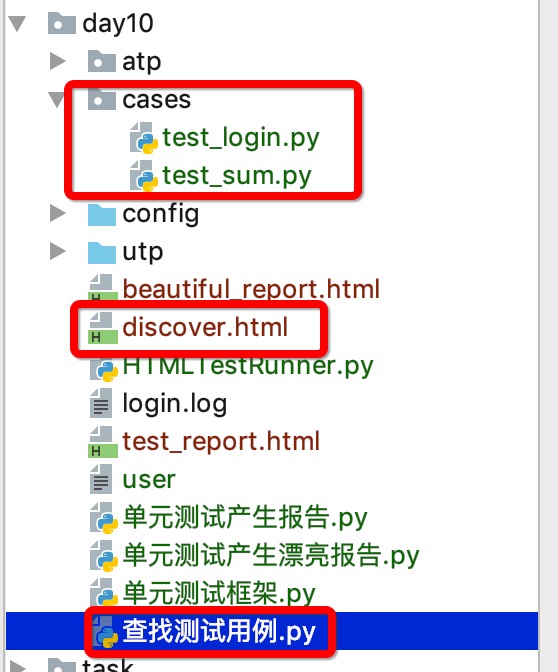
b.cases中内容:
test_login.py
import unittest import requests class LoginCla(unittest.TestCase): def test_login(self): '''登录接口--必填参数''' url = 'http://api.nnzhp.cn/api/user/login' d = {'username': 'wangxiaoyu', 'passwd': 'Yu9251314'} result = requests.post(url, data=d).text self.assertIn('userId', result, '返回结果中没有找到userId') def test_login2(self): '''登录接口--必填为空''' url = 'http://api.nnzhp.cn/api/user/login' d = {'username': '', 'passwd': 'Yu9241314'} result = requests.post(url, data=d).text self.assertIn('userId', result, '返回结果中没有找到userId')
test_sum.py中内容:
import unittest def sum(a,b): return a+b class SumCla(unittest.TestCase): def test_sum(self): '''加法运算--正常情况''' self.assertEqual(3,sum(1,2)) def test_sum2(self): '''加法运算--异常情况''' self.assertEqual(6,sum(2,3),'期望结果应该是5')
c.查找测试用例
import unittest from BeautifulReport import BeautifulReport suite = unittest.defaultTestLoader.discover('cases','*.py') bf = BeautifulReport(suite) bf.report(description='所有模块测试情况如下:',filename='discover.html') 测试结果: .F.F 测试已全部完成, 可前往/Users/wangxiaoyu/PycharmProjects/python_automated_development/day10查询测试报告
浏览器访问discover.html
……
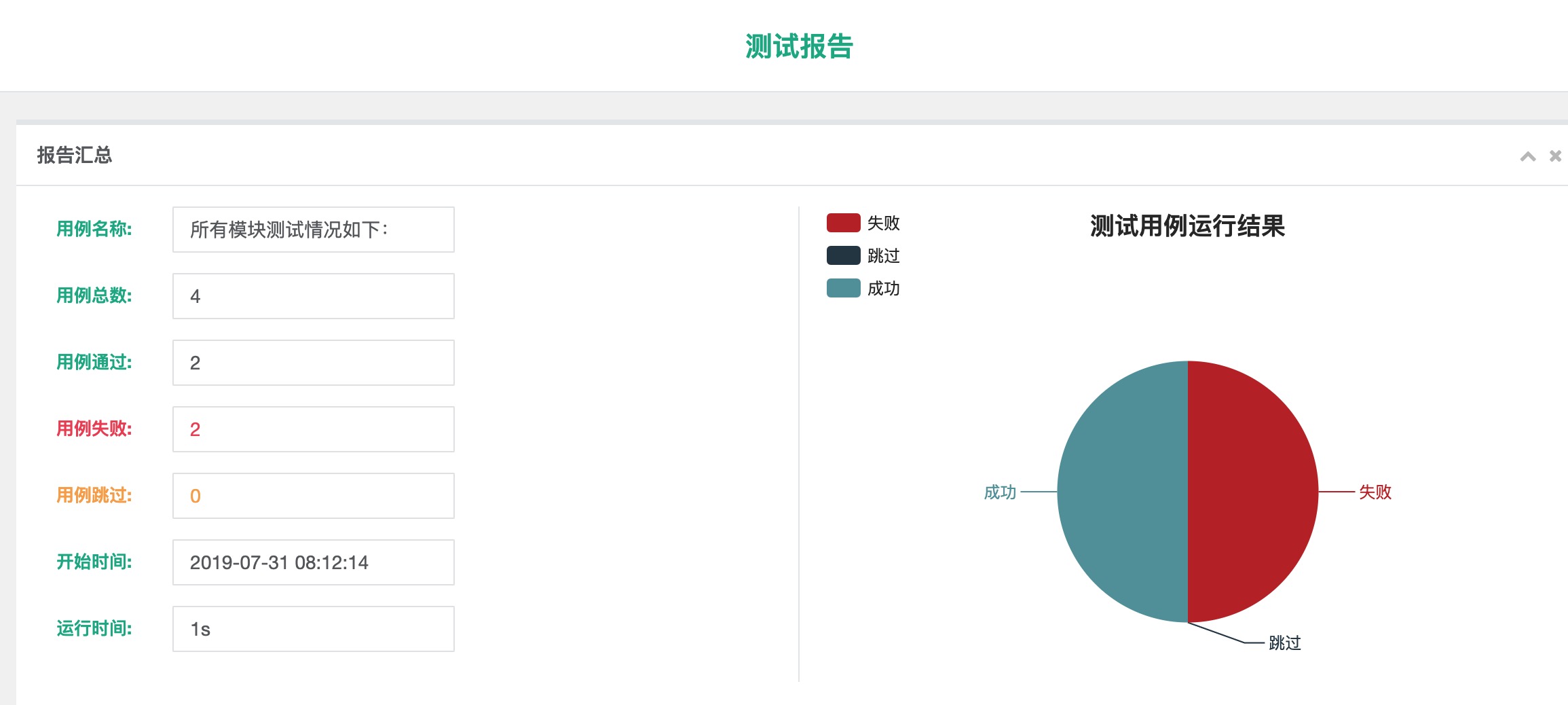
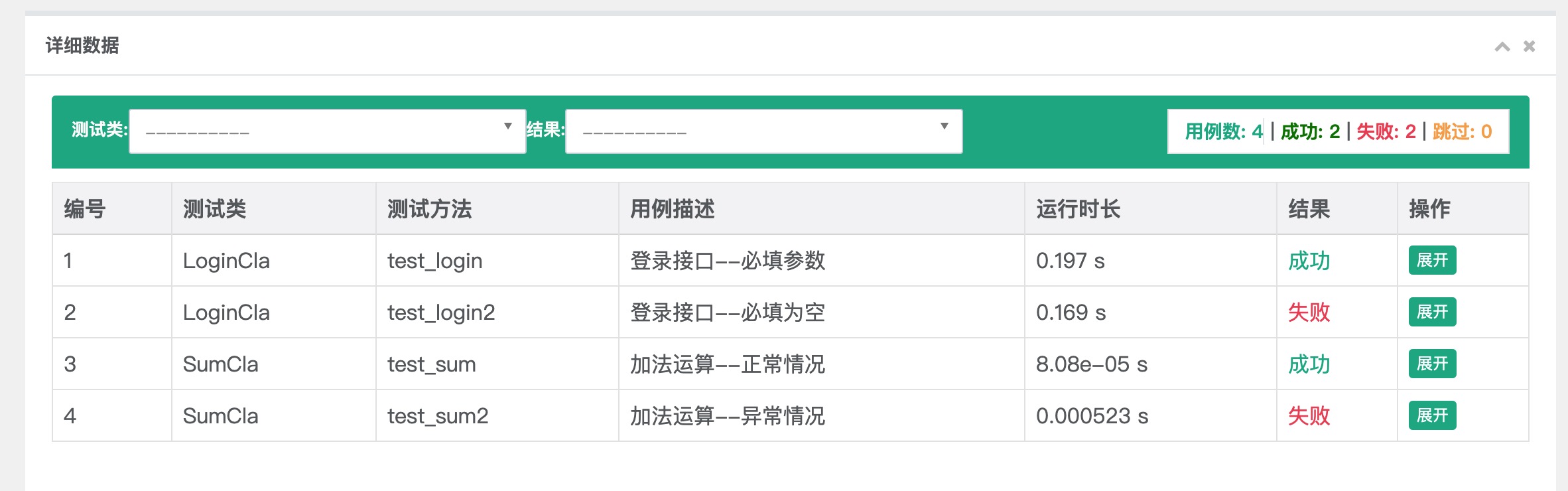