spring-boot - demo
当我发现把最初的一个demo整的面目全非的时候,突然想要找一个简单的demo做测试,发现与其在原来的上面该,还不如新建一个demo。
官方入门:http://projects.spring.io/spring-boot/
最熟悉maven,这次先做一个maven的demo。
创建maven project。
pom:

<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.test</groupId> <artifactId>demo</artifactId> <version>1.0-SNAPSHOT</version> <repositories> <!--<repository>--> <!--<id>spring-snapshots</id>--> <!--<url>http://maven.oschina.net/content/groups/public/</url>--> <!--<snapshots>--> <!--<enabled>true</enabled>--> <!--</snapshots>--> <!--</repository>--> <repository> <id>springsource-repos</id> <name>SpringSource Repository</name> <url>http://repo.spring.io/release/</url> </repository> <repository> <id>central-repos</id> <name>Central Repository</name> <url>http://repo.maven.apache.org/maven2</url> </repository> <repository> <id>central-repos2</id> <name>Central Repository 2</name> <url>http://repo1.maven.org/maven2/</url> </repository> </repositories> <dependencyManagement> <dependencies> <dependency> <!-- Import dependency management from Spring Boot --> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-dependencies</artifactId> <version>1.4.0.RELEASE</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <optional>true</optional> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> <configuration> <fork>true</fork> </configuration> <dependencies> <!-- spring热部署--> <dependency> <groupId>org.springframework</groupId> <artifactId>springloaded</artifactId> <version>1.2.6.RELEASE</version> </dependency> </dependencies> </plugin> </plugins> </build> </project>
这里添加了web基础依赖,模板为thymeleaf, 添加一个简单的security配置。
创建src/resources/application.yml:
spring:
profiles:
active: dev
创建src/resources/application-dev.yml:
#用户名 密码配置
security:
user:
name: admin
password: test
创建启动入口hello.SampleController:

package hello; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; @Controller @SpringBootApplication public class SampleController { @RequestMapping("/") public String home() { return "redirect:/hello/index"; } public static void main(String[] args) throws Exception { SpringApplication.run(SampleController.class, args); } }
创建一个Controller: hello.HelloController

package hello; import hello.entity.User; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.validation.Errors; import org.springframework.web.bind.annotation.ModelAttribute; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.ResponseBody; import javax.validation.Valid; import java.util.HashMap; import java.util.Map; /** * Created by miaorf on 2016/8/2. */ @RequestMapping("/hello") @Controller public class HelloController { @RequestMapping("/index") public String hello(Model model, @RequestParam(defaultValue = "Ryan") String name){ model.addAttribute("name",name); return "index"; } @ResponseBody @RequestMapping("/info") public Map info(@Valid @ModelAttribute("user")User user, Errors errors){ Map map = new HashMap(); if (errors.hasErrors()){ map.put("error",errors.getAllErrors()); }else{ map.put("user",user); } return map; } }
这里连个路由。一个指向模板文件index,一个返回json并添加了参数校验。
需要使用的实体类:hello.entity.User

package hello.entity; import org.hibernate.validator.constraints.Length; import javax.validation.constraints.NotNull; import javax.validation.constraints.Pattern; /** * Created by miaorf on 2016/8/2. */ public class User { @NotNull(message = "用户名不能为空") @Length(min=5, max=20, message="用户名长度必须在5-20之间") @Pattern(regexp = "^[a-zA-Z_]\\w{4,19}$", message = "用户名必须以字母下划线开头,可由字母数字下划线组成") private String name; @NotNull(message = "age不能为空") private int age; @NotNull(message = "sex不能为空") private String sex; public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getSex() { return sex; } public void setSex(String sex) { this.sex = sex; } }
需要用到的模板文件:src/resources/templates/index.html

<!DOCTYPE HTML> <html xmlns:th="http://www.thymeleaf.org"> <head> <title>Getting Started: Serving Web Content</title> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> </head> <body> <p th:text="'Hello, ' + ${name} + '!'" /> </body> </html>
github: https://github.com/chenxing12/spring-boot-demo
关注我的公众号
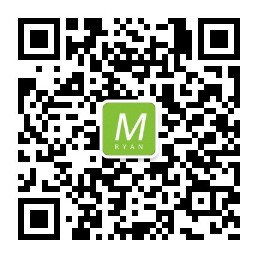