python函数和常用模块(二),Day4
- 内置函数2
- 装饰器
- 字符串格式化
- 生成器
- 迭代器
- 递归
- 模块
- 序列化相关
- time模块
- datetime模块
内置函数2
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 | callable () # 是否可以被执行,是否可以被调用 chr () # ascii转字符 ord () # 字符转ascii compile () # 编译 eval () # 执行 exec () # 执行 dict () dir () # 快速查看对象为提供了哪些功能 help () # divmod () #输出(商,余数) isinstance () # 判断对象是否是某个类的实例 filter () # 函数返回True 将元素添加到结果中 map () # 函数的返回值添加到结果中 float () # format () # frozenset () # globals () # 所有的全部变量 localse() #所有的局部变量 hash () # 生成哈希值 id () # 查看内存地址 issubclass () # 查看一个类是不是它的子类(派生类) iter () # len () # 查看长度 "李杰"3.x 长度为2(按字符计算)2.7.x中 长度为6 按字节计算 max () min () sum () memoryview() # 跟内存地址相关的一个类 iter () next () object () # 一个类 pow () # 次方 property () range () repr () reversed () # 反转 round () # 四舍五入 slice () # sorted # 排序 vars () # 当前模块有哪些可以调用? zip () # 实例 :随机验证码 improt random random.randrange( 1 , 5 ) import random r = random.randrange( 65 , 91 ) li = [] for i in range ( 6 ): r = random.randrange( 0 , 5 ) if r = = 2 or r = = 4 : temp = random.randrange( 0 , 10 ) li.append( str (temp)) else : temp = random.randrange( 65 , 91 ) c = chr (temp) li.append(c) result = "".join(li) print (result) |
装饰器
1 2 3 4 5 6 7 | 1. 定义函数,为调用,函数内部不执行 2. 函数名 > 代指函数 @ + 函数名 功能: 1. 自动执行outer函数并且将其下面的函数f1当做参数传递 2. 将outer函数的返回值,重复赋值给f1 |
字符串格式化
百分号
format
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | tpl = "i am {}, age {}, {}" . format ( "seven" , 18 , 'alex' ) print (tpl) tpl = "i am {}, age {}, {}" . format ( * [ "seven" , 18 , 'alex' ]) print (tpl) tpl = "i am {0}, age {1}, really {0}" . format ( "seven" , 18 ) print (tpl) tpl = "i am {0}, age {1}, really {0}" . format ( * [ "seven" , 18 ]) print (tpl) tpl = "i am {name}, age {age}, really {name}" . format (name = "seven" , age = 18 ) print (tpl) tpl = "i am {name}, age {age}, really {name}" . format ( * * { "name" : "seven" , "age" : 18 }) print (tpl) tpl = "i am {0[0]}, age {0[1]}, really {0[2]}" . format ([ 1 , 2 , 3 ], [ 11 , 22 , 33 ]) print (tpl) tpl = "i am {:s}, age {:d}, money {:f}" . format ( "seven" , 18 , 88888.1 ) print (tpl) tpl = "i am {:s}, age {:d}" . format ( * [ "seven" , 18 ]) print (tpl) tpl = "i am {name:s}, age {age:d}" . format (name = "seven" , age = 18 ) print (tpl) tpl = "i am {name:s}, age {age:d}" . format ( * * { "name" : "seven" , "age" : 18 }) print (tpl) tpl = "numbers: {:b},{:o},{:d},{:x},{:X}, {:%}" . format ( 15 , 15 , 15 , 15 , 15 , 15.87623 , 2 ) print (tpl) tpl = "numbers: {:b},{:o},{:d},{:x},{:X}, {:%}" . format ( 15 , 15 , 15 , 15 , 15 , 15.87623 , 2 ) print (tpl) tpl = "numbers: {0:b},{0:o},{0:d},{0:x},{0:X}, {0:%}" . format ( 15 ) print (tpl) tpl = "numbers: {num:b},{num:o},{num:d},{num:x},{num:X}, {num:%}" . format (num = 15 ) print (tpl) |
生成器
迭代器
递归
模块
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | * py:模块 * 其他:类库 1. 内置模块 2. 自定义模块 3. 第三方模块 安装: * pip3 * 源码 * 先导入 * 再使用 可以是文件 可以是文件夹 |
序列化相关
json
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | #导入json模块 import json # 将python基本数据类型转换成字符串形式 json.dumps() # 将python字符串形式转换成基本数据类型 json.loads() # 将python基本数据类型转换成字符串形式,并写入文件 json.dump() # 读取文件字符串,字符串形式转换成基本数据类型 json.load() 通过loads()反序列化时,一定要使用"" # 例 import json dic = { 'k1' : 'v1' } print (dic, type (dic)) # 将python的基本数据类型转换成字符串形式 result = json.dumps(dic) print (result, type (result)) # 将python字符串形式转换成基本数据类型 s1 = '{"k1": 123}' dic1 = json.loads(s1) print (dic1, type (dic1)) li = [ 11 , 22 , 33 ] json.dump(li, open ( 'db' , 'w' )) li = json.load( open ( 'db' , 'r' )) print (li, type (li)) |
pickle
1 2 3 4 5 6 7 8 9 10 11 | import pickle li = [ 11 , 22 , 33 ] r = pickle.dumps(li) print (r) restult = pickle.loads(r) print (restult) pickle.dumps() pickle.loads() pickle.dump() pickle.load() |
json更适合跨语言,字符串,基本类型做操作
pickle,python所有类型做操作
time模块
1 2 3 4 5 6 7 8 9 10 11 12 13 | # time module print (time.clock()) # 返回处理器时间 3.3开始废弃 print (time.process_time()) # 返回处理器时间 3.3开始废弃 print (time.time()) # 返回当前系统时间戳 print (time.ctime()) # 输出Wed Sep 14 16:16:10 2016, 当前系统时间 print (time.ctime(time.time() - 86640 )) # 将时间戳转为字符串格式 print (time.gmtime(time.time() - 86640 )) # 将时间戳转换struct_time格式 print (time.gmtime()) print (time.localtime()) # 将时间戳转换struct_time格式,但返回的是本地时间 print (time.mktime(time.localtime())) # 与time.localtiem()功能想反,将struct_time格式转回时间戳格式 # time.sleep(4) print (time.strftime( "%Y-%m-%d %H:%M:%S" , time.localtime())) print (time.strptime( "2016-09-14" , "%Y-%m-%d" )) |
datetime模块
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | # datetime module print (datetime.date.today()) # 输出格式2016-09-14 print (datetime.date.fromtimestamp(time.time())) # 输出格式2016-09-14 时间戳转成日期 current_time = datetime.datetime.now() print (current_time) # 输出格式2016-09-14 17:53:24.499480 print (current_time.timetuple()) # 返回struct_time格式 print (current_time.replace( 2014 , 9 , 12 )) # 输出2014-09-12 18:17:20.264601 返回当前时间,但是指定值将被替换 str_to_date = datetime.datetime.strptime( "21/11/06 16:30" , "%d/%m/%y %H:%M" ) print (str_to_date) print (datetime.datetime.now() + datetime.timedelta(days = 10 )) # 比现在加10天 print (datetime.datetime.now() + datetime.timedelta(days = - 10 )) # 比现在减10天 print (datetime.datetime.now() + datetime.timedelta(hours = 10 )) # 比现在加10小时 print (datetime.datetime.now() + datetime.timedelta(seconds = 120 )) # 比现在加120秒 |
logging模块
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | import logging # 打印输出日志 logging.warning( "user [alex] attempted wrong password more then 4 times" ) logging.critical( "server is down" ) # 将级别高于等于info的 写入日志文件 logging.basicConfig(filename = 'example.log' , level = logging.INFO) logging.debug( 'This message should go to the log file' ) logging.info( 'So should this' ) logging.warning( 'And this, to' ) logging.basicConfig(filename = 'example.log' , level = logging.INFO, format = '%(asctime)s %(message)s' , datefmt = '%m/%d/%Y %I:%M:%S %p' ) logging.debug( 'This message should go to the log file' ) logging.info( 'So should this' ) logging.warning( 'And this, to' ) |
logging还要扩展用法 以后补充
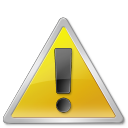
【推荐】国内首个AI IDE,深度理解中文开发场景,立即下载体验Trae
【推荐】编程新体验,更懂你的AI,立即体验豆包MarsCode编程助手
【推荐】抖音旗下AI助手豆包,你的智能百科全书,全免费不限次数
【推荐】轻量又高性能的 SSH 工具 IShell:AI 加持,快人一步