[SpringBoot] - 配置文件的多种形式及优先级
学习两个注解: @PropertySource @ImportResource ↓
@ConfigurationProperties
-
与 @Bean 结合为属性赋值
-
与 @PropertySource (只能用于properties文件)结合读取指定文件
:: @PropertySource : 加载指定的文件
将原来application.properties中的person的配置字段
放入单独的person.properties中.
并在person类上加上该注解
@PropertySource(value = {"classpath:person.properties"})
public class Person {
@ImportResource 读取外部配置文件 不推荐
导入Spring的配置文件,让配置文件里面的内容生效.
SpringBoot里面没有Spring的配置文件,我们自己编写的配置文件,也不能自动识别.
想让Spring的配置文件生效,加载进来, 需要@ImportResource标注在一个配置类上
这里放在启动类上:
@ImportResource(locations = {"classpath:beans.xml"})
@SpringBootApplication
public class Demo11Application {
public static void main(String[] args) {
SpringApplication.run(Demo11Application.class, args);
}
}
不推荐:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beanshttp://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="helloService" class="com.example.demo11.service.HelloService"></bean>
</beans>
SpringBoot推荐给容器中添加组件的方式: 推荐使用全注解的方式:
-
配置类 ===== 类似Spring配置文件
-
使用@Bean给容器中添加组件:
package com.example.demo11.config;
import com.example.demo11.service.HelloService;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
/**
* @Configuration : 指明当前类是一个配置类,就是来替代之前的Spring配置文件
* 在配置文件中用<bean></bean>标签添加组件.
*/
@Configuration
public class MyAppConfig {
//将方法的返回值添加到容器中;容器中这个组件默认的id就是方法名
@Bean
public HelloService helloService(){
System.out.println("配置类@Bean给容器添加组件了.");
return new HelloService();
}
}
>
|
>
之前的@ConfigurationProperties(prefix = "person")默认从全局配置文件中获取值.
使用@PropertySource注解可以从自建的配置文件中获取值,((只能用于properties文件)
新建一个person.properties文件,将原本的application.properties中person的字段剪切过去.
在bean上这样声明:
@PropertySource(value = {"classpath:person.properties"})
public class Person {
同样能获取值
Spring官方不推荐这种方式,不仅要写xml也与SpringBoot宗旨不符合.
推荐使用一个配置类来做配置:
创建一个config包,创建一个配置类:
MyAppConfig:
package com.example.demo11.config; import com.example.demo11.service.HelloService; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; /** * @Configuration : 指明当前类是一个配置类,就是来替代之前的Spring配置文件 * 在配置文件中用<bean></bean>标签添加组件. */ @Configuration public class MyAppConfig { //将方法的返回值添加到容器中;容器中这个组件默认的id就是方法名 @Bean public HelloService helloService(){ System.out.println("配置类@Bean给容器添加组件了."); return new HelloService(); } }
Profile
1.多Profile文件
我们在主配置文件编写的时候,文件名可以是: application-{profile}.properties.yml
程序会默认使用application.properties的配置:
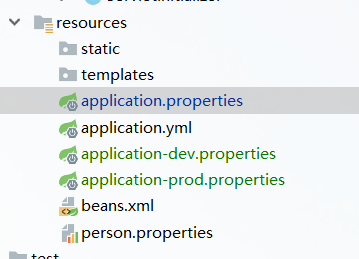
在默认的配置文件application.properties中,激活application-dev.properties环境
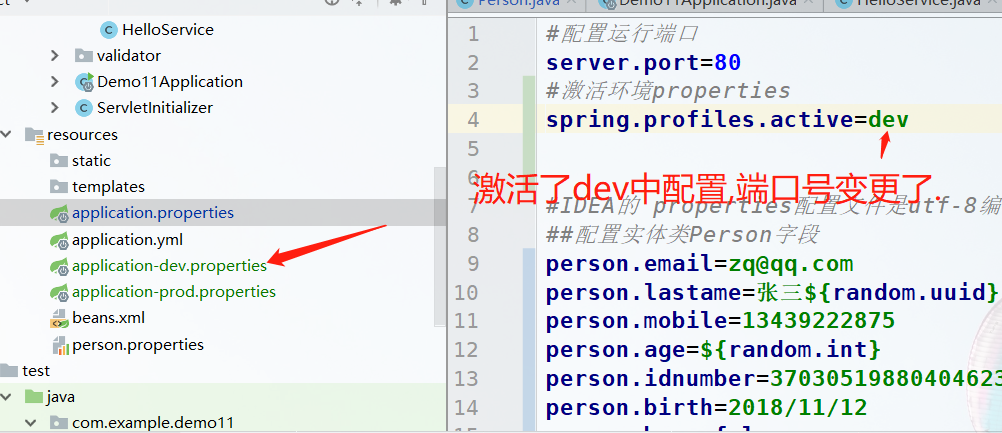
2.yml支持多文档块方式 ( 以 --- 隔开配置 用active激活某个文档块中的配置 )
多profile文档块模式:
application.yml :
server:
port: 8081
spring:
profiles:
# active: prod
active: dev #做为指定环境,激活哪个环境
---
server:
port: 8083
spring:
profiles: dev
---
server:
port: 8084
spring:
profiles: prod #指定属于哪个环境
---
3.激活指定profile
- 在配置文件中指定要激活哪个配置: ( application.properties )
application.properties :
#激活环境properties
spring.profiles.active=dev
- 命令行方式激活某配置 :
除了在IDEA的Terminal也可以右键target, [Show in Explorer],找到jar或war包文件目录,在路径上覆盖写入cmd回车即打开该文件目录的cmd
java -jar demoxxxxx.war --spring.profiles.active=dev
可以看到其运行端口已经换位dev的8083
- 配置IDEA的Edit Configurations设置 : ( Program arguments 程序参数 )
在IDEA的Edit Configurations设置: Program arguments 字段 :
--spring.profiles.active=dev
- 配置JVM ( java虚拟机 ) 参数 : ( VM options 虚拟机选项 )
-Dspring.profiles.active=dev
补充:
配置文件加载位置:
springboot启动会扫描以下位置的application.properties或者application.yml文件做为springboot的默认配置文件
-
file: ../config/
-
file:../
-
classpath:/config/
-
classpath:/
以上都是按照优先级从高到底的顺序,所有位置的文件都会被加载,高优先级配置内容会覆盖低优先级配置内容.
我们也可以通过配置spring.config.location来改变默认配置
SpringBoot会从这四个位置全部加载主配置文件: 互补配置.(下面提到)
重要:补充
在整体项目中建立config文件夹: 其中的application.properties会最优先执行.
也就是在项目的最外层建立文件夹config为最优先级,即上面的file:../config/ 其次是直接不在config文件夹直接在外面建立配置文件,
这样的配置文件都要比classpath也就是 resources 下面的优先级要高.
在有config文件夹时,如果外面classpath还有配置application.yml时,如果
只是
server:
port: 8081
那么还是会执行config文件夹下的配置.
但是如果在classpath中使用的是:
spring:
profiles:
active: dev
---
server:
port: 8083
spring:
profiles: dev
那么还是会先执行这里的yml激活的8083端口.
如果在config文件夹下有application.properties和application.yml
指定了不同端口而yml没有激活active指定配置代码块,那么会读取properties的配置.
同样,在IDEA的Edit配置中,使用-Dspring.profiles.active=dev,如果在config和classpath中的yml
都有dev环境时,会优先指定config目录下的.
在使用java -jar xxxx.jar --spring.profiles.active=prod 时,即使在Edit Configurations指定了-Dspring.profiles.active=dev,
但是还是会使用prod的配置.这是命令行的最优先级.
上面提到互补配置:
即是说在config中的配置端口会执行,但是如果classpath中的配置文件多了一个配置如:
server.servlet.context-path=/boot123
那么在项目启动后,该路径也会加载进来.
本文来自博客园,作者:ukyo--君君小时候,转载请注明原文链接:https://www.cnblogs.com/ukzq/p/9952085.html