在测试框架中使用Log4J 2
之前的测试框架:http://www.cnblogs.com/tobecrazy/p/4553444.html
配合Jenkins可持续集成:http://www.cnblogs.com/tobecrazy/p/4529399.html
这次demo的代码已经放到github:https://github.com/tobecrazy/Demo
打log是一个测试框架必备的功能之一,trace测试执行的内容,使测试分析更容易和有规律可循。进而进一步处理测试log,实现自动分析测试结果。
现在java项目写日志一般使用Log4j 2
log4j 2是一个开源的记录log的框架,比log4j 效率更高
更多内容:http://logging.apache.org/log4j/2.x/manual/index.html
首先下载相应的jar包,放到工程:
接下来创建Log类,使用
clazz.getCanonicalName() 获取类名字,为将来case执行获取case名和page名和action名
package com.log; import org.apache.logging.log4j.LogManager; import org.apache.logging.log4j.Logger; public class Log { private final Class<?> clazz; private Logger logger; /** * * @param clazz */ Log(Class<?> clazz) { this.clazz = clazz; this.logger = LogManager.getLogger(this.clazz); } /** * @author Young * @param message * */ public void info(String message) { logger.info(clazz.getCanonicalName() + ": " + message); } /** * @author Young * @param message */ public void debug(String message) { logger.debug(clazz.getCanonicalName() + ": " + message); } /** * @author Young * @param message */ public void error(String message) { logger.error(clazz.getCanonicalName() + ": " + message); } /** * @author Young * @param message */ public void trace(String message) { logger.trace(clazz.getCanonicalName() + ": " + message); } /** * @author Young * @param message */ public void warn(String message) { logger.warn(clazz.getCanonicalName() + ": " + message); } /** * @author Young * @param message */ public void fatal(String message) { logger.fatal(clazz.getCanonicalName() + ": " + message); } }
接下来就可以直接调用这个类:
package com.log; public class test { public static void main(String[] args) { // TODO Auto-generated method stub Log log=new Log(test.class); log.info("this is my test"); log.debug("this is a debug"); log.error("this is an error "); log.fatal("this is a fatal"); log.trace("this is a trace"); } }
然后, run as application 吧
结果如下: holy shit,damn it 居然报错
ERROR StatusLogger No log4j2 configuration file found. Using default configuration: logging only errors to the console.
23:52:47.679 [main] ERROR com.log.test - com.log.test: this is an error
23:52:47.680 [main] FATAL com.log.test - com.log.test: this is a fatal
原来是缺少config
于是乎准备一份log4j2.xml文件,放在该package下:

<?xml version="1.0" encoding="UTF-8"?> <Configuration status="WARN"> <Appenders> <Console name="Console" target="SYSTEM_OUT"> <PatternLayout pattern="%d{YYYY-MM-dd HH:mm:ss} [%t] %-5level %logger{36} - %msg%n"/> </Console> <File name="log" fileName="logs/test.log" append="false"> <PatternLayout pattern="%d{YYYY-MM-dd HH:mm:ss.SSS} %-5level %class{36} %L %M - %msg%xEx%n"/> </File> </Appenders> <Loggers> <Logger name="com.dbyl.libarary.utils.Log" level="all"> <AppenderRef ref="Console"/> <AppenderRef ref="log"/> </Logger> <Root level="trace"> <AppenderRef ref="Console"/> <AppenderRef ref="log"/> </Root> </Loggers> </Configuration>
但是依然不行,没法加参数
加上:
File config=new File("C:/Users/Young/workspace/Log4j/src/com/log/log4j2.xml"); ConfigurationSource source = new ConfigurationSource(new FileInputStream(config),config); Configurator.initialize(null, source);
妥妥的 , 运行结果如下:
2015-06-07 00:06:31 [main] INFO com.log.test - com.log.test: this is my test
2015-06-07 00:06:31 [main] DEBUG com.log.test - com.log.test: this is a debug
2015-06-07 00:06:31 [main] ERROR com.log.test - com.log.test: this is an error
2015-06-07 00:06:31 [main] FATAL com.log.test - com.log.test: this is a fatal
2015-06-07 00:06:31 [main] TRACE com.log.test - com.log.test: this is a trace
并且logs目录下生城相应的log文件
当然,这并不完美,log4j可以放在src目录下,这要就不需要指定位置
接下来把这个log类放在测试框架里:
在basePage加入相应的代码,如下:
package com.dbyl.libarary.utils; import java.io.IOException; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.interactions.Actions; import org.openqa.selenium.support.ui.ExpectedCondition; import org.openqa.selenium.support.ui.WebDriverWait; public class BasePage { protected WebDriver driver; protected String[][] locatorMap; protected Log log= new Log(this.getClass()); protected BasePage(WebDriver driver) throws IOException { this.driver = driver; locatorMap = ReadExcelUtil.getLocatorMap(); } protected void type(Locator locator, String values) throws Exception { WebElement e = findElement(driver, locator); log.info("type value is: "+ values); e.sendKeys(values); } protected void click(Locator locator) throws Exception { WebElement e = findElement(driver, locator); log.info("click button"); e.click(); } protected void clickAndHold(Locator locator) throws IOException { WebElement e = findElement(driver, locator); Actions actions = new Actions(driver); actions.clickAndHold(e).perform(); } public WebDriver getDriver() { return driver; } public void setDriver(WebDriver driver) { this.driver = driver; } public WebElement getElement(Locator locator) throws IOException { return getElement(this.getDriver(), locator); } /** * get by parameter * * @author Young * @param driver * @param locator * @return * @throws IOException */ public WebElement getElement(WebDriver driver, Locator locator) throws IOException { locator = getLocator(locator.getElement()); WebElement e; switch (locator.getBy()) { case xpath: log.debug("find element By xpath"); e = driver.findElement(By.xpath(locator.getElement())); break; case id: log.debug("find element By id"); e = driver.findElement(By.id(locator.getElement())); break; case name: log.debug("find element By name"); e = driver.findElement(By.name(locator.getElement())); break; case cssSelector: log.debug("find element By cssSelector"); e = driver.findElement(By.cssSelector(locator.getElement())); break; case className: log.debug("find element By className"); e = driver.findElement(By.className(locator.getElement())); break; case tagName: log.debug("find element By tagName"); e = driver.findElement(By.tagName(locator.getElement())); break; case linkText: log.debug("find element By linkText"); e = driver.findElement(By.linkText(locator.getElement())); break; case partialLinkText: log.debug("find element By partialLinkText"); e = driver.findElement(By.partialLinkText(locator.getElement())); break; default: e = driver.findElement(By.id(locator.getElement())); } return e; } public boolean isElementPresent(WebDriver driver, Locator myLocator, int timeOut) throws IOException { final Locator locator = getLocator(myLocator.getElement()); boolean isPresent = false; WebDriverWait wait = new WebDriverWait(driver, 60); isPresent = wait.until(new ExpectedCondition<WebElement>() { @Override public WebElement apply(WebDriver d) { return findElement(d, locator); } }).isDisplayed(); return isPresent; } /** * This Method for check isPresent Locator * * @param locator * @param timeOut * @return * @throws IOException */ public boolean isElementPresent(Locator locator, int timeOut) throws IOException { return isElementPresent(driver,locator, timeOut); } /** * * @param driver * @param locator * @return */ public WebElement findElement(WebDriver driver, final Locator locator) { WebElement element = (new WebDriverWait(driver, locator.getWaitSec())) .until(new ExpectedCondition<WebElement>() { @Override public WebElement apply(WebDriver driver) { try { return getElement(driver, locator); } catch (IOException e) { // TODO Auto-generated catch block log.error("can't find element "+locator.getElement()); return null; } } }); return element; } public Locator getLocator(String locatorName) throws IOException { Locator locator; for (int i = 0; i < locatorMap.length; i++) { if (locatorMap[i][0].endsWith(locatorName)) { return locator = new Locator(locatorMap[i][1]); } } return locator = new Locator(locatorName); } }
在UITest类也加入相应的代码:

/** * */ package com.dbyl.libarary.utils; import org.openqa.selenium.WebDriver; /** * @author Young * */ public class UITest { WebDriver driver; Log log=new Log(this.getClass()); public WebDriver getDriver() { return driver; } /** * init test case * * @param driver */ public void setDriver(WebDriver driver) { this.driver = driver; } public void init(WebDriver driver) { log.info("Start WebDriver"); setDriver(driver); } /** * stop webdriver * * @param driver */ public void stop() { log.info("Stop WebDriver"); driver.quit(); } }
接下来就是见证奇迹的时候:
运行一下:log能够清晰记录测试时所做的内容
也有相应的file log
今天的工程下载地址:http://pan.baidu.com/s/1kTqvzZx
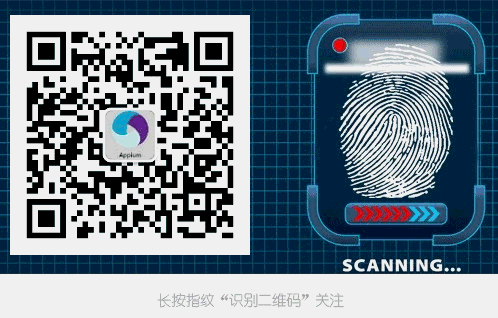