Template Specializations vs. function overloading
As convention, I prefer to pass the object as const reference below.
void foo(const A& a)
In most of cases, normal conversions are applied to the arguments when the client invokes the function. For example.
A a;
c.foo(a);
Object a will convert to const reference of class A.
However, when we use template specializations, conversions are not applied to argument types. In a call to specialized version of a template, the argument types in the call must match the specialized version function parameter type(s) exactly. If they don't, then the complier will instantiate an instantiation for the argument(s) from the template definition.
class A
{
};
class C
{
public:
template<typename T>
void foo(T t)
{
printf("void foo(T t)\n");
}
template<>
void foo(const int i)
{
printf("void foo(int i)\n");
}
template<>
void foo(const A& a)
{
printf("void foo(const A a)\n");
}
};
TEST(TemplateTest, TemplateSpecializationTest)
{
C c;
int i = 2;
c.foo(i);
A a;
c.foo(a);
}
Because the parameter to call foo is (A a) install of (const A& a), the complier pick the template version void foo(T t) instead of specialized version void foo(const A& a) .
So, in such case, I prefer to use function overloading instead of template specializations.
class A
{
};
class C
{
public:
template<typename T>
void foo(T t)
{
printf("void foo(T t)\n");
}
template<>
void foo(const int i)
{
printf("void foo(const int i)\n");
}
void foo(const A& a)
{
printf("void foo(const A a)\n");
}
};
TEST(TemplateTest, TemplateSpecializationTest)
{
C c;
int i = 2;
c.foo(i);
A a;
c.foo(a);
}
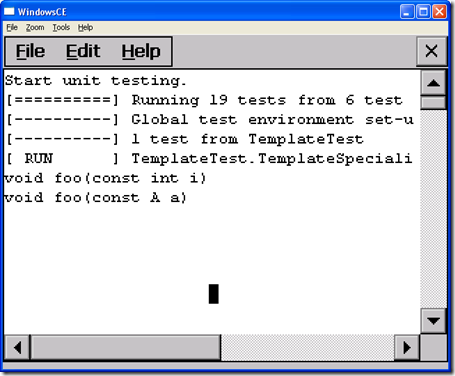
Works as I expect. Conversions are applied to argument when calls foo() function.
refer to C++ Primer.
Update
If we use template specializations and overloaded functions at the same time. The VC++(VS2005) complier will pick up the overloaded function.
class A
{
};
class C
{
public:
template<typename T>
void foo(T t)
{
printf("void foo(T t)\n");
}
template<>
void foo(const int i)
{
printf("void foo(int i)\n");
}
template<>
void foo(A a)
{
printf("template<> void foo(A a)\n");
}
void foo(A a);
};
TEST(TemplateTest, TemplateSpecializationTest)
{
C c;
int i = 2;
c.foo(i);
A a;
c.foo(a);
}
void C::foo(A a)
{
printf("void foo(A a)\n");
}
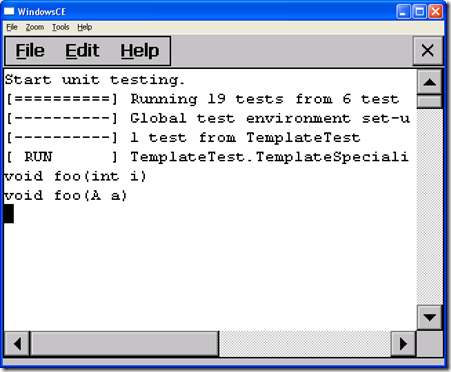
But I don’t recommend to use both function templates and nontemplate functions at the same time. Because it will surprise the users to use it.
出处:http://procoder.cnblogs.com
本作品由Jake Lin创作,采用知识共享署名-非商业性使用-禁止演绎 2.5 中国大陆许可协议进行许可。 任何转载必须保留完整文章,在显要地方显示署名以及原文链接。如您有任何疑问或者授权方面的协商,请给我留言。