第二道算法题(500分)
题目要求:双倍超立方数是指一个正整数可以正好被拆分为两种不同的a^3+b^3的方式,其中a,b均为整数且0<a<=b。对于任何一个指定的 int n, 返回所有的小于等于n的双倍超立方数的个数。

Code
1 using System;
2 using System.Collections.Generic;
3 using System.Linq;
4 using System.Text;
5 using System.Collections;
6
7 namespace ConsoleApplication1
8 {
9 class Program
10 {
11 static void Main(string[] args)
12 {
13 int n = int.Parse(Console.ReadLine());
14
15 System.Diagnostics.Stopwatch watch = new System.Diagnostics.Stopwatch();
16 watch.Start();
17 List<int> baseNums = new List<int>();
18 Dictionary<int, int> Results = new Dictionary<int, int>();
19 int ResultCount = 0;
20
21 for (int a = 1; a * a * a < n; a++)
22 {
23 baseNums.Add(a * a * a);
24 }
25 for (int a = 0; a < baseNums.Count; a++)
26 {
27 for (int b = a; b < baseNums.Count; b++)
28 {
29 int _temp = baseNums[a] + baseNums[b];
30 if (_temp < n + 1)
31 {
32 if (Results.ContainsKey(_temp))
33 {
34 Results[_temp] += 1;
35 }
36 else
37 {
38 Results.Add(_temp, 1);
39 }
40 }
41 }
42 }
43
44 foreach (var item in Results)
45 {
46 if (item.Value == 2)
47 {
48 ResultCount += 1;
49 }
50 }
51
52 watch.Stop();
53 Console.WriteLine("we found {0} results in {1} milliseconds", ResultCount, watch.ElapsedMilliseconds);
54 }
55 }
56 }
57
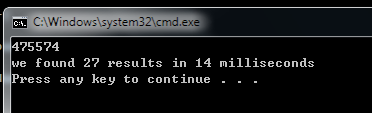
这一题大概是这么算的吧。。有更好的算法吗?还望指教