ipc的remoting
远程对象:
using System;
using System.Collections.Generic;
using System.Text;
namespace Object
{
public class MyObject : MarshalByRefObject
{
public int Add(int a, int b)
{
return a + b;
}
}
}
using System.Collections.Generic;
using System.Text;
namespace Object
{
public class MyObject : MarshalByRefObject
{
public int Add(int a, int b)
{
return a + b;
}
}
}
1、配置文件方式
服务端:
using System;
using System.Collections.Generic;
using System.Text;
using System.Runtime.Remoting;
namespace Server
{
class Program
{
static void Main(string[] args)
{
RemotingConfiguration.Configure("Server.exe.config");
Console.ReadLine();
}
}
}
using System.Collections.Generic;
using System.Text;
using System.Runtime.Remoting;
namespace Server
{
class Program
{
static void Main(string[] args)
{
RemotingConfiguration.Configure("Server.exe.config");
Console.ReadLine();
}
}
}
服务端配置文件:
<configuration>
<system.runtime.remoting>
<application name="RemoteServer">
<service>
<wellknown type="Object.MyObject,Object" objectUri="Object.MyObject" mode="Singleton" />
</service>
<channels>
<channel ref="ipc" portName="testPipe" />
</channels>
</application>
</system.runtime.remoting>
</configuration>
<system.runtime.remoting>
<application name="RemoteServer">
<service>
<wellknown type="Object.MyObject,Object" objectUri="Object.MyObject" mode="Singleton" />
</service>
<channels>
<channel ref="ipc" portName="testPipe" />
</channels>
</application>
</system.runtime.remoting>
</configuration>
客户端:
using System;
using System.Collections.Generic;
using System.Text;
namespace Client
{
class Program
{
static void Main(string[] args)
{
Object.MyObject app = (Object.MyObject)Activator.GetObject(typeof(Object.MyObject), "ipc://testPipe/Object.MyObject");
Console.WriteLine(app.Add(1, 2));
Console.ReadLine();
}
}
}
using System.Collections.Generic;
using System.Text;
namespace Client
{
class Program
{
static void Main(string[] args)
{
Object.MyObject app = (Object.MyObject)Activator.GetObject(typeof(Object.MyObject), "ipc://testPipe/Object.MyObject");
Console.WriteLine(app.Add(1, 2));
Console.ReadLine();
}
}
}
2、代码方式
服务端:
using System;
using System.Collections.Generic;
using System.Text;
using System.Runtime.Remoting.Channels.Ipc;
using System.Runtime.Remoting.Channels;
using System.Runtime.Remoting;
namespace Server
{
class Program
{
static void Main(string[] args)
{
IpcChannel ipcCh = new IpcChannel("testPipe");
ChannelServices.RegisterChannel(ipcCh);
RemotingConfiguration.RegisterWellKnownServiceType
(typeof(Object.MyObject), "Object.MyObject", WellKnownObjectMode.Singleton);
Console.ReadLine();
}
}
}
using System.Collections.Generic;
using System.Text;
using System.Runtime.Remoting.Channels.Ipc;
using System.Runtime.Remoting.Channels;
using System.Runtime.Remoting;
namespace Server
{
class Program
{
static void Main(string[] args)
{
IpcChannel ipcCh = new IpcChannel("testPipe");
ChannelServices.RegisterChannel(ipcCh);
RemotingConfiguration.RegisterWellKnownServiceType
(typeof(Object.MyObject), "Object.MyObject", WellKnownObjectMode.Singleton);
Console.ReadLine();
}
}
}
客户端和配置文件方式相同
欢迎大家阅读我的极客时间专栏《Java业务开发常见错误100例》【全面避坑+最佳实践=健壮代码】
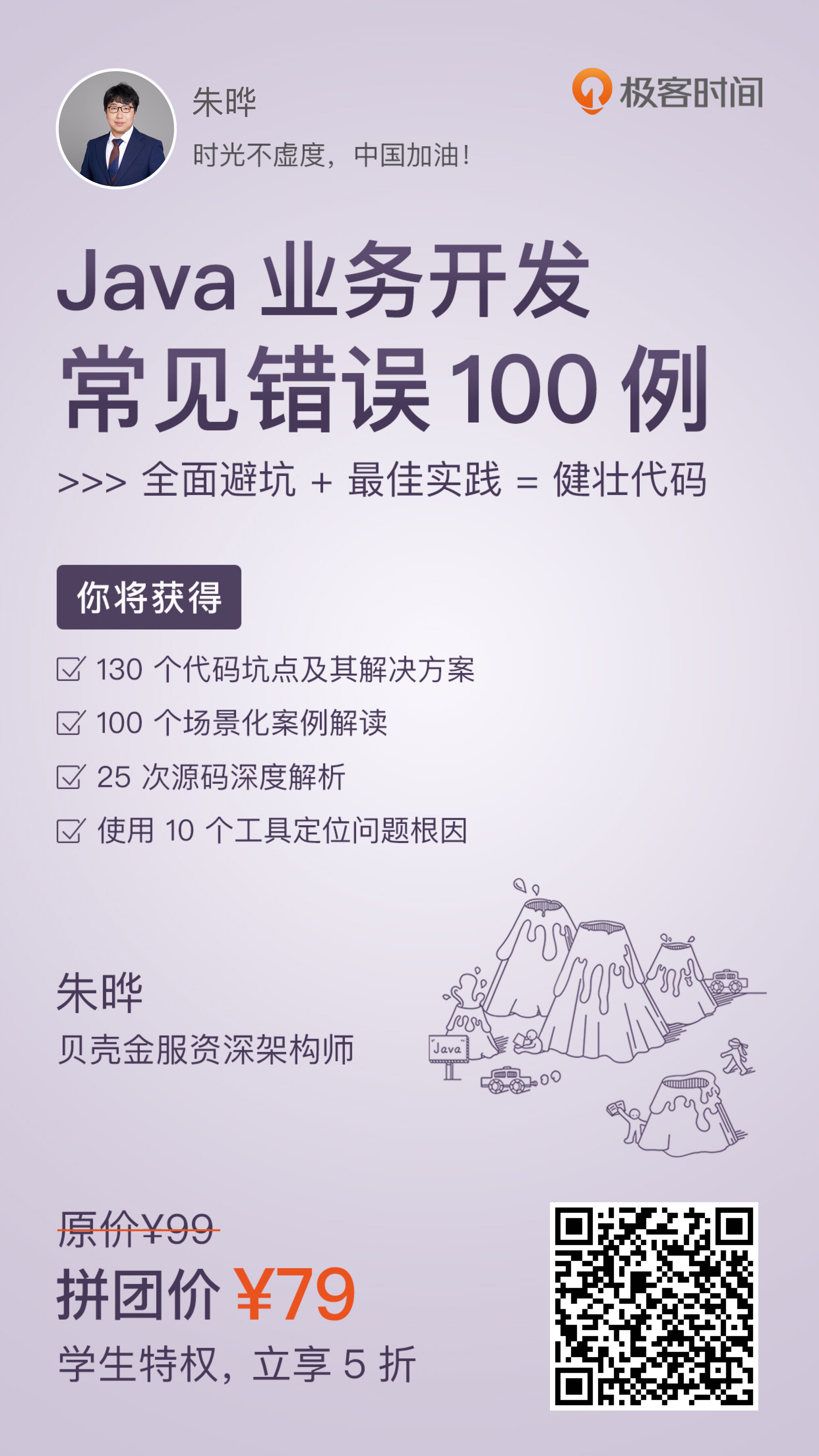