近来有用户反映系统老是重复添加记录,一查,原因是用户在提交数据的时候,浏览器反应较慢,用户误以为程序停止,于是再次点击提交按钮,于是问题出现.
于是想到一种办法,在用户提交数据之后,当前网页最顶端显示一个透明的大小和当前浏览器客户区域同等的DIV,提示用户当前操作正在进行. 如此,用户会看到数据执行过程,就不会再次提交.
时间仓促,代码公布如下,有几处属性需要添加设计师支持. 来日有时间再完善.

Code
1
using System;
2
using System.Collections.Generic;
3
using System.ComponentModel;
4
using System.Linq;
5
using System.Text;
6
using System.Web;
7
using System.Web.UI;
8
using System.Web.UI.WebControls;
9
using System.Drawing;
10
using System.Security.Permissions;
11
namespace Loading
12

{
13
[ToolboxData("<{0}:DongLoading runat=server></{0}:DongLoading>")]
14
[Localizable(true)]
15
public class DongLoading : WebControl
16
{
17
private string imagePath = "1";
18
private string backColor = "AliceBlue";
19
private string targetControlID = string.Empty;
20
//private Color dongBorderColor;
21
22
[Category("DongUI"), TypeConverter(typeof(WebColorConverter)), DefaultValue(typeof(Color), ""), NotifyParentProperty(true)]
23
public Color DongBorderColor
24
{
25
get
26
{
27
return (Color)this.ViewState["DongBorderColor"];
28
}
29
set
30
{
31
this.ViewState["DongBorderColor"] = value;
32
}
33
}
34
35
36
37
Property#region Property
38
[Category("DongUI"), Description("指定做为进度提示的背景透明图片的路径"),Browsable(true)]
39
public string ImagePath
40
{
41
get
{ return this.imagePath; }
42
set
43
{
44
imagePath = value;
45
}
46
}
47
48
[Category("DongUI")]
49
[Description("指定背景颜色")]
50
[Browsable(true)]
51
public string BackColor
52
{
53
get
{ return this.backColor; }
54
set
55
{
56
backColor = value;
57
}
58
}
59
60
[Category("DongUI")]
61
[Description("指定目标控件")]
62
[Browsable(true), TypeConverter(typeof(ControlIDConverter)), DefaultValue(typeof(Button), null)]
63
public string TargetControlID
64
{
65
get
{ return this.ViewState["TargetControlID"].ToString(); }
66
set
67
{
68
ViewState["TargetControlID"] = value;
69
}
70
}
71
#endregion
72
73
override#region override
74
/**//// <summary>
75
/// Renders the contents.
76
/// </summary>
77
/// <param name="output">The output.</param>
78
protected override void RenderContents(HtmlTextWriter output)
79
{
80
// 隐藏透明面板
81
output.Write("<script> window.document.all." + this.ClientID + ".style.display=\"none\";</script>");
82
}
83
84
/**//// <summary>
85
/// 将控件的 HTML 开始标记呈现到指定的编写器中。此方法主要由控件开发人员使用。
86
/// </summary>
87
/// <param name="writer"><see cref="T:System.Web.UI.HtmlTextWriter"/>,表示要在客户端呈现 HTML 内容的输出流。</param>
88
public override void RenderBeginTag(HtmlTextWriter writer)
89
{
90
string color = DongBorderColor.IsNamedColor == true ? DongBorderColor.Name : "#" + DongBorderColor.Name;
91
string html = "<div id=\"" + this.ClientID + "\" oncontextmenu=\"return false;\" style=\" left: 0;top: 0; position: absolute; width: expression( window.document.documentElement.offsetWidth );" +
92
" height: expression( window.document.documentElement.clientHeight > window.document.documentElement.scrollHeight ? window.document.documentElement.clientHeight : window.document.documentElement.scrollHeight ); " +
93
" z-index: 888; filter: progid:DXImageTransform.Microsoft.Alpha(opacity=80); display: inline; background-color: " + color + "\">";
94
html += " <img style=\" position: absolute;left: expression( document.documentElement.clientWidth / 2 - this.clientWidth / 2 ); top: expression( document.documentElement.clientHeight / 2 - this.clientHeight / 2 + document.documentElement.scrollTop ); \" src=\"" + ImagePath + "\">";
95
writer.Write(html);
96
}
97
98
/**//// <summary>
99
/// 将控件的 HTML 结束标记呈现到指定的编写器中。此方法主要由控件开发人员使用。
100
/// </summary>
101
/// <param name="writer"><see cref="T:System.Web.UI.HtmlTextWriter"/>,表示要在客户端呈现 HTML 内容的输出流。</param>
102
public override void RenderEndTag(HtmlTextWriter writer)
103
{
104
if (Page != null)
105
{
106
string js = "window.document.all[\'" + this.ClientID + "\'].style.display=\'inline\'";
107
Button bt = this.Page.FindControl(TargetControlID) as Button;
108
bt.Attributes.Add("onclick", js);
109
}
110
writer.Write("</div>");
111
}
112
#endregion
113
}
114
}
115
效果:
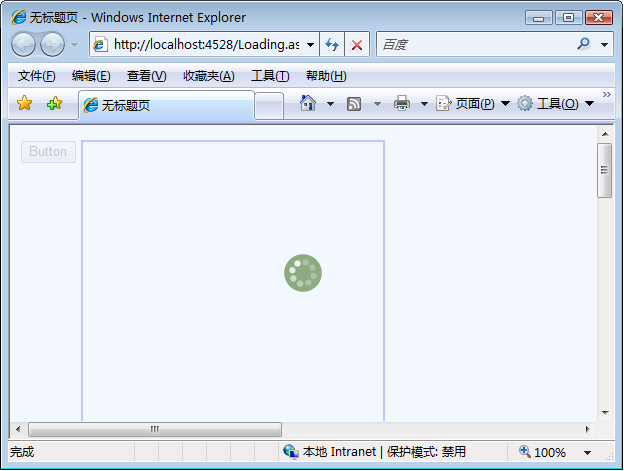
1 <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Loading.aspx.cs" Inherits="WebApplication1.Loading" %>
2 <%@ Register Assembly="Loading" Namespace="Loading" TagPrefix="cc1" %>
3 <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
4 <html xmlns="http://www.w3.org/1999/xhtml">
5 <head runat="server">
6 <title>无标题页</title>
7 </head>
8 <body>
9 <form id="form1" runat="server">
10 <div style="height: 1316px">
11 <cc1:DongLoading ID="DongLoading1" runat="server" BackColor="Aliceblue" ImagePath="hi.gif"
12 TargetControlID="Button1" DongBorderColor="AliceBlue" />
13 <asp:Button ID="Button1" runat="server" Text="Button" OnClick="Button1_Click" />
14 <div style="position:absolute; width:300px; height:1300px; border: solid 2px blue;"></div>
15 </div>
16 </form>
17 </body>
18 </html>