c++沉思录 学习笔记 第六章 句柄(引用计数指针雏形?)
一个简单的point坐标类
class Point {
public:
Point():xval(0),yval(0){}
Point(int x,int y):xval(x),yval(y){}
int x()const { return xval; }
int y()const { return yval; }
Point& x(int xv) { xval = xv; return *this; }
Point& y(int yv) { yval = yv; return *this; }
private:
int xval, yval;
};
将handle 绑定到其上 进行一层封装
通过handle控制那些操作可以公开 那些操作私有 阻止用户对point的地址的操作
更重要的是提供 point的引用计数 将多个handle绑定到ppint上 当全部释放对point的使用权时
才进行释放point的操作
那么增加两个类
一个引用计数类 class UPoint
class UPoint {
friend class Handle;
Point p;
int u;
UPoint() :u(1) {}
UPoint(int x, int y) :p(x, y), u(1) {}
UPoint(const Point& p0):p(p0),u(1){}
};
一个是handle句柄类 包含UPoint的指针 构造和析构的时候会将计数+1
当计数到0 则释放指针内存
class Handle {
public:
Handle();
Handle(int, int);
Handle(const Point&);
Handle(const Handle&);
Handle& operator=(const Handle&);
~Handle();
int x()const;
Handle& x(int);
int y()const;
Handle& y(int);
private:
UPoint* up;
};
全部代码如下:

1 // sample0.cpp: 定义控制台应用程序的入口点。 2 // 3 4 #include "stdafx.h" 5 #include <iostream> 6 7 class Point { 8 public: 9 Point():xval(0),yval(0){} 10 Point(int x,int y):xval(x),yval(y){} 11 int x()const { return xval; } 12 int y()const { return yval; } 13 Point& x(int xv) { xval = xv; return *this; } 14 Point& y(int yv) { yval = yv; return *this; } 15 private: 16 int xval, yval; 17 }; 18 19 class UPoint { 20 friend class Handle; 21 Point p; 22 int u; 23 24 UPoint() :u(1) {} 25 UPoint(int x, int y) :p(x, y), u(1) {} 26 UPoint(const Point& p0):p(p0),u(1){} 27 }; 28 29 class Handle { 30 public: 31 Handle(); 32 Handle(int, int); 33 Handle(const Point&); 34 Handle(const Handle&); 35 Handle& operator=(const Handle&); 36 ~Handle(); 37 int x()const; 38 Handle& x(int); 39 int y()const; 40 Handle& y(int); 41 private: 42 UPoint* up; 43 }; 44 45 Handle::Handle() :up(new UPoint) {} 46 Handle::Handle(int x, int y) : up(new UPoint(x, y)) {} 47 Handle::Handle(const Point& p) : up(new UPoint(p)) {} 48 49 Handle::~Handle() { 50 if (up->u == 0) 51 delete up; 52 } 53 54 Handle::Handle(const Handle& h) :up(h.up) { ++up->u; } 55 Handle& Handle::operator=(const Handle& h) { 56 ++h.up->u; 57 if (--up->u == 0) { 58 delete up; 59 } 60 return *this; 61 } 62 63 int Handle::x()const { return up->p.x(); } 64 int Handle::y()const { return up->p.y(); } 65 66 Handle& Handle::x(int x0) 67 { 68 up->p.x(x0); 69 return *this; 70 } 71 72 Handle& Handle::y(int y0) { 73 up->p.y(y0); 74 return *this; 75 } 76 77 78 int main() 79 { 80 Handle h; 81 std::cout << h.x() << " " << h.y() << std::endl; 82 return 0; 83 }
欢迎转帖 请保持文本完整并注明出处
技术博客 http://www.cnblogs.com/itdef/
B站算法视频题解
https://space.bilibili.com/18508846
qq 151435887
gitee https://gitee.com/def/
欢迎c c++ 算法爱好者 windows驱动爱好者 服务器程序员沟通交流
如果觉得不错,欢迎点赞,你的鼓励就是我的动力

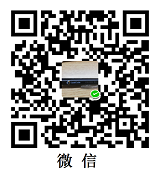