c++沉思录 学习笔记 第五章 代理类
Vehicle 一个车辆的虚基类
class Vehicle {
public:
virtual double weight()const = 0;
virtual void start() = 0;
virtual Vehicle* copy() = 0;
virtual ~Vehicle() {};
};
衍生出RoadVehicle AutoVehicle两个类
如果有一个停车场类 可以容纳100辆车
一开始计划 有Vehicle[100]这种变量来表示停车场
但是Vehicle是虚基类 不能有实例化的实体 也就不会有数组
所以需要一个代理类来保存Vehicle的指针 它可以指向任意的Vehicle的衍生类
同时考虑到拷贝操作 添加了拷贝函数
class VehicelSurrogate {
public:
VehicelSurrogate() :vp(0) {};
VehicelSurrogate( Vehicle& v) :vp(v.copy()) {};
VehicelSurrogate(const VehicelSurrogate& v):vp(v.vp ? v.vp->copy() : 0) {};
~VehicelSurrogate() {
delete vp;
};
VehicelSurrogate& operator=(const VehicelSurrogate& v) {
if (this != &v) {
delete vp;
vp = (v.vp ? v.vp->copy() : 0);
}
return *this;
};
double weight() {
return vp->weight();
}
private:
Vehicle* vp;
};
如此 就可以使用VehicelSurrogate[100]来表示停车场的概念
完整代码如下

1 // test5.cpp: 定义控制台应用程序的入口点。 2 // 3 4 #include "stdafx.h" 5 #include <iostream> 6 7 class Vehicle { 8 public: 9 virtual double weight()const = 0; 10 virtual void start() = 0; 11 virtual Vehicle* copy() = 0; 12 virtual ~Vehicle() {}; 13 }; 14 15 class RoadVehicle:public Vehicle{ 16 public: 17 virtual double weight() const{ 18 return 1; 19 } 20 virtual void start() {} 21 virtual Vehicle* copy() { 22 return new RoadVehicle(*this); 23 } 24 virtual ~RoadVehicle() {} 25 }; 26 class AutoVehicle:public RoadVehicle{ 27 public: 28 virtual double weight() const{ 29 return 2; 30 } 31 virtual void start() {} 32 virtual Vehicle* copy() { 33 return new AutoVehicle(*this); 34 } 35 virtual ~AutoVehicle() {} 36 37 }; 38 39 40 class VehicelSurrogate { 41 public: 42 VehicelSurrogate() :vp(0) {}; 43 VehicelSurrogate( Vehicle& v) :vp(v.copy()) {}; 44 VehicelSurrogate(const VehicelSurrogate& v):vp(v.vp ? v.vp->copy() : 0) {}; 45 ~VehicelSurrogate() { 46 delete vp; 47 }; 48 VehicelSurrogate& operator=(const VehicelSurrogate& v) { 49 if (this != &v) { 50 delete vp; 51 vp = (v.vp ? v.vp->copy() : 0); 52 } 53 return *this; 54 }; 55 double weight() { 56 return vp->weight(); 57 } 58 59 private: 60 Vehicle* vp; 61 }; 62 63 64 int main() 65 { 66 VehicelSurrogate parking_lot[100]; 67 AutoVehicle x; 68 parking_lot[0] = x; 69 std::cout << parking_lot[0].weight() << std::endl;; 70 return 0; 71 }
欢迎转帖 请保持文本完整并注明出处
技术博客 http://www.cnblogs.com/itdef/
B站算法视频题解
https://space.bilibili.com/18508846
qq 151435887
gitee https://gitee.com/def/
欢迎c c++ 算法爱好者 windows驱动爱好者 服务器程序员沟通交流
如果觉得不错,欢迎点赞,你的鼓励就是我的动力

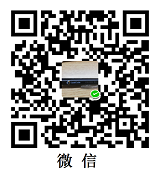