模板学习实践三 functor
#include <iostream>
#include <typeinfo>
void foo()
{
std::cout << "foo() called" << std::endl;
}
typedef void FooT(); // FooT is a function type,
// the same type as that of function foo()
int main()
{
foo(); // direct call
// print types of foo and FooT
std::cout << "Types of foo: " << typeid(foo).name()
<< '\n';
std::cout << "Types of FooT: " << typeid(FooT).name()
<< '\n';
FooT* pf = foo; // implicit conversion (decay)
pf(); // indirect call through pointer
(*pf)(); // equivalent to pf()
// print type of pf
std::cout << "Types of pf: " << typeid(pf).name()
<< '\n';
FooT& rf = foo; // no implicit conversion
rf(); // indirect call through reference
// print type of rf
std::cout << "Types of rf: " << typeid(rf).name()
<< '\n';
}
//==========================================================
#include <iostream>
// class for function objects that return constant value
class ConstantIntFunctor {
private:
int value; // value to return on ``function call''
public:
// constructor: initialize value to return
ConstantIntFunctor(int c) : value(c) {
}
// ``function call''
int operator() () const {
return value;
}
};
// client function that uses the function object
void client(ConstantIntFunctor const& cif)
{
std::cout << "calling back functor yields " << cif() << '\n';
}
int main()
{
ConstantIntFunctor seven(7);
ConstantIntFunctor fortytwo(42);
client(seven);
client(fortytwo);
}
//===========================================================
/* The following code example is taken from the book
* "C++ Templates - The Complete Guide"
* by David Vandevoorde and Nicolai M. Josuttis, Addison-Wesley, 2002
*
* (C) Copyright David Vandevoorde and Nicolai M. Josuttis 2002.
* Permission to copy, use, modify, sell and distribute this software
* is granted provided this copyright notice appears in all copies.
* This software is provided "as is" without express or implied
* warranty, and with no claim as to its suitability for any purpose.
*/
#include <vector>
#include <iostream>
#include <cstdlib>
// wrapper for function pointers to function objects
template<int(*FP)()>
class FunctionReturningIntWrapper {
public:
int operator() () {
return FP();
}
};
// example function to wrap
int random_int()
{
return std::rand(); // call standard C function
}
// client that uses function object type as template parameter
template <typename FO>
void initialize(std::vector<int>& coll)
{
FO fo; // create function object
for (std::vector<int>::size_type i = 0; i<coll.size(); ++i) {
coll[i] = fo(); // call function for function object
}
}
int main()
{
// create vector with 10 elements
std::vector<int> v(10);
// (re)initialize values with wrapped function
initialize<FunctionReturningIntWrapper<random_int> >(v);
// output elements
for (std::vector<int>::size_type i = 0; i<v.size(); ++i) {
std::cout << "coll[" << i << "]: " << v[i] << std::endl;
}
}
欢迎转帖 请保持文本完整并注明出处
技术博客 http://www.cnblogs.com/itdef/
B站算法视频题解
https://space.bilibili.com/18508846
qq 151435887
gitee https://gitee.com/def/
欢迎c c++ 算法爱好者 windows驱动爱好者 服务器程序员沟通交流
如果觉得不错,欢迎点赞,你的鼓励就是我的动力

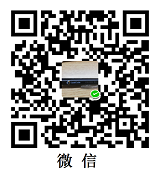