2019 GDUT Rating Contest I : Problem G. Back and Forth
题面:
G. Back and Forth
On Tuesday, he takes a bucket from the first barn, fills it, and carries the milk to the second barn, where he pours it into the storage tank. He leaves the bucket at the second barn.
On Wednesday, he takes a bucket from the second barn (possibly the one he left on Tuesday), fills it, and carries the milk to the first barn, where he pours it into the storage tank. He leaves the bucket at the first barn.
On Thursday, he takes a bucket from the first barn (possibly the one he left on Wednesday), fills it, and carries the milk to the second barn, where he pours it into the tank. He leaves the bucket at the second barn.
On Friday, he takes a bucket from the second barn (possibly the one he left on Tuesday or Thursday), fills it, and carries the milk to the first barn, where he pours it into the tank. He leaves the bucket at the first barn.
- 1000: FJ could carry the same bucket back and forth in each trip, leaving the total amount in the first barn’s tank unchanged.
- 1003: FJ could carry 2 units on Tuesday, then 5 units on Wednesday, then 1 unit on Thursday, and 1 unit on Friday.
- 1004: FJ could carry 1 unit on Tuesday, then 5 units on Wednesday, then 1 unit on Thursday, and 1 unit on Friday.
- 1007: FJ could carry 1 unit on Tuesday, then 5 units on Wednesday, then 2 units on Thursday, and 5 units on Friday.
- 1008: FJ could carry 1 unit on Tuesday, then 5 units on Wednesday, then 1 unit on Thursday, and 5 units on Friday.
题目描述:
题目分析:
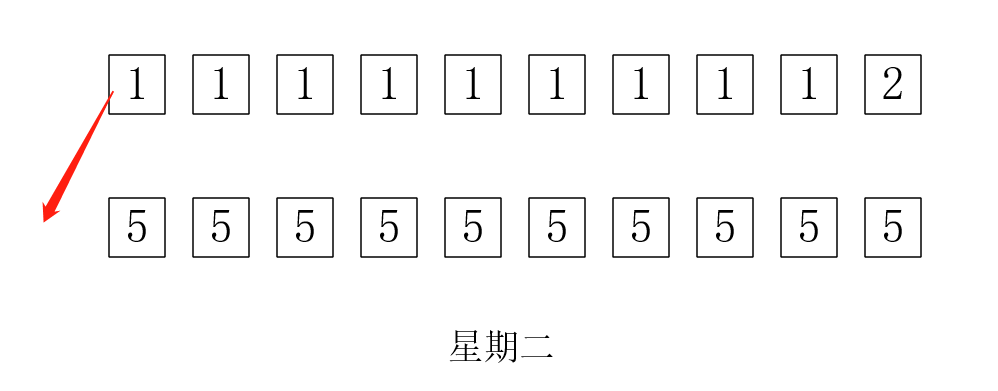
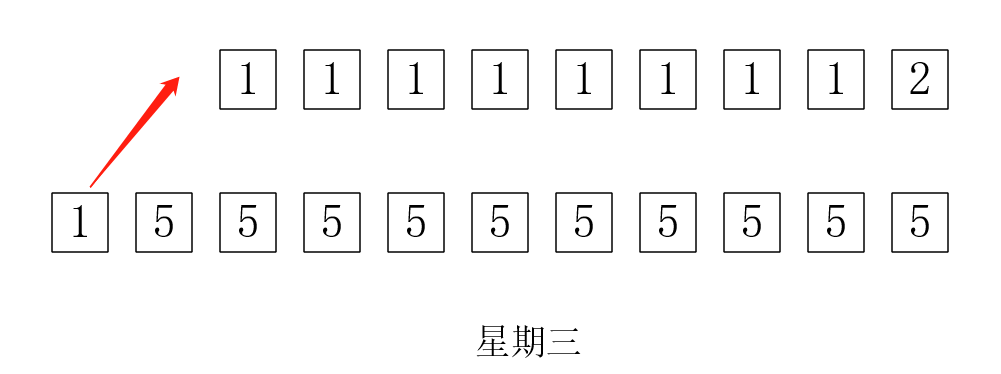
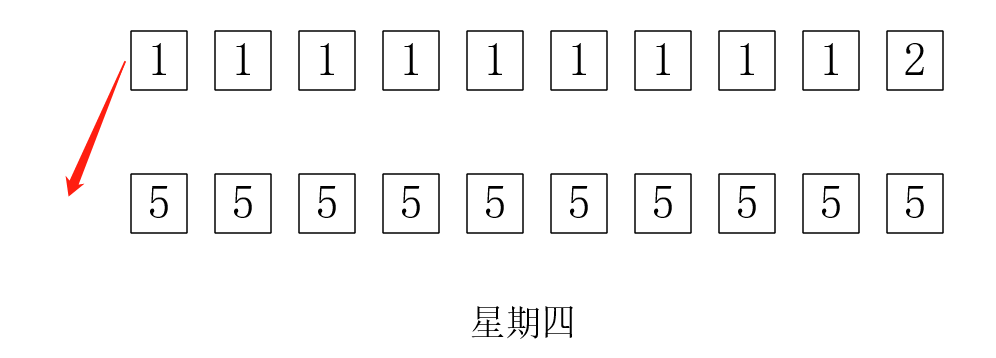
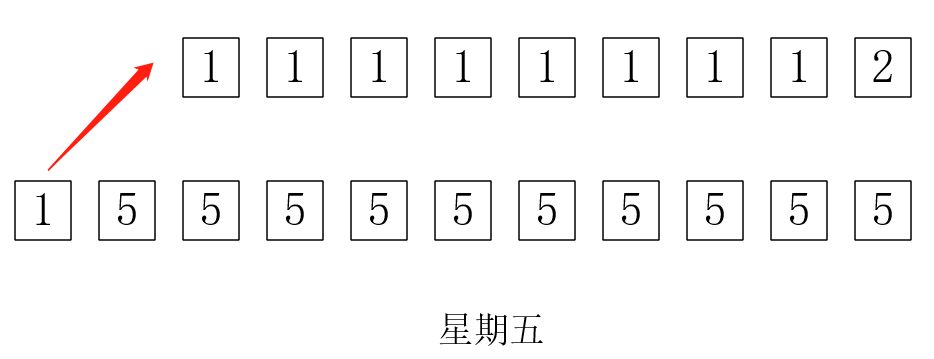
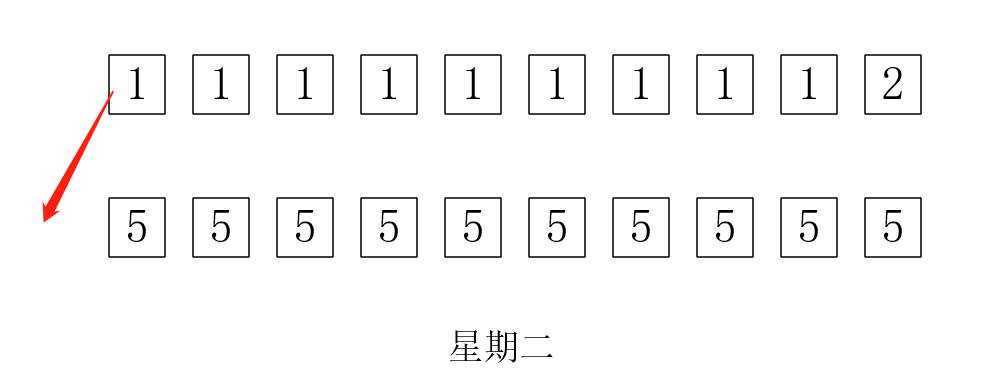
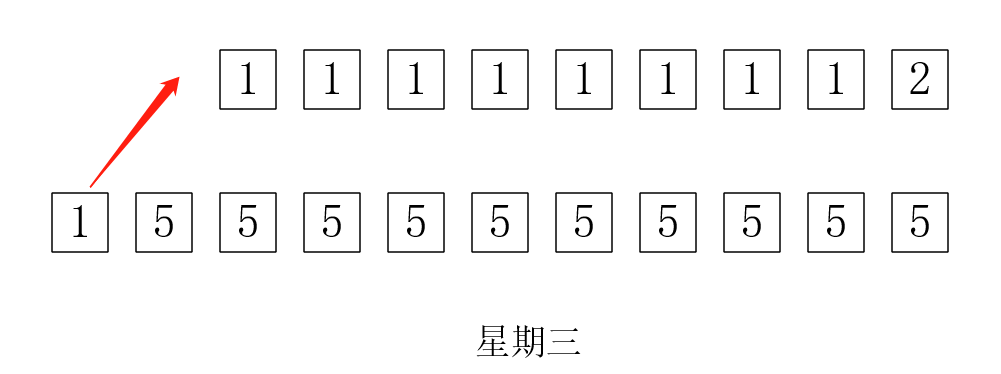
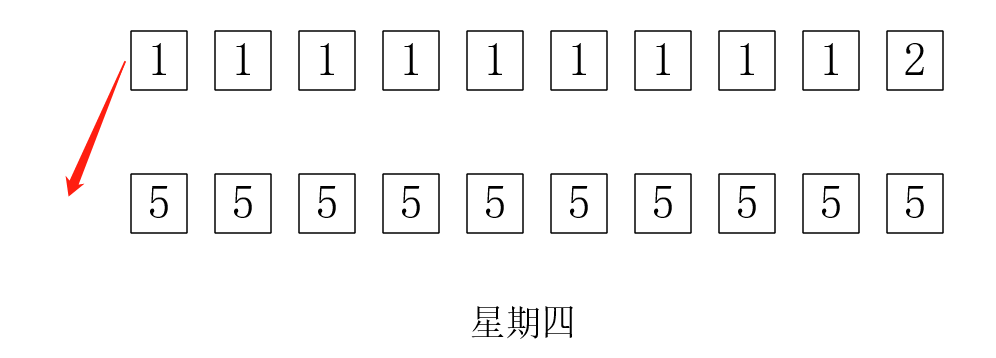
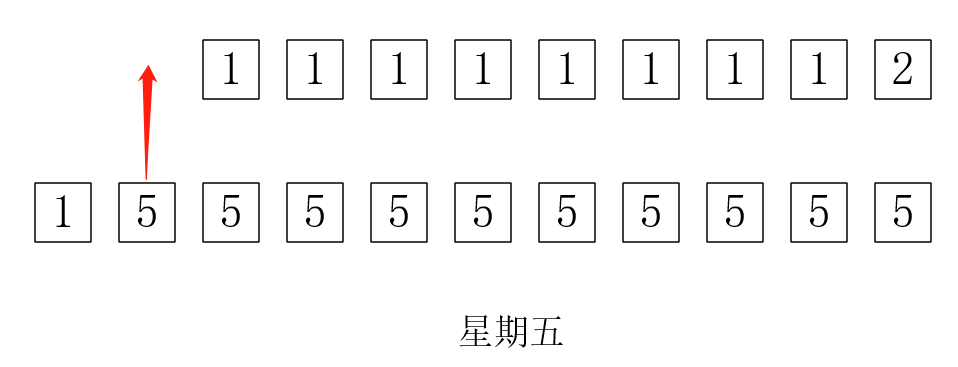
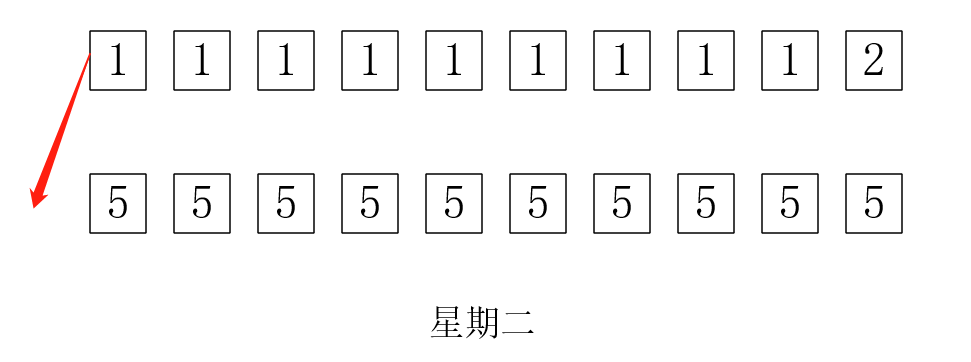
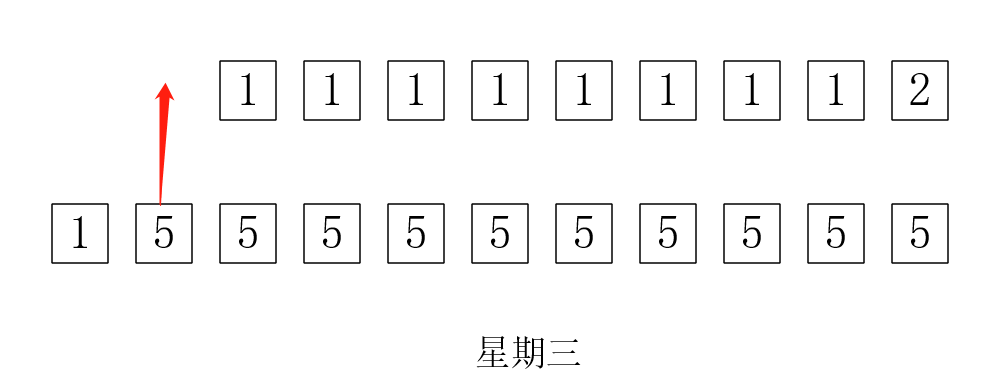
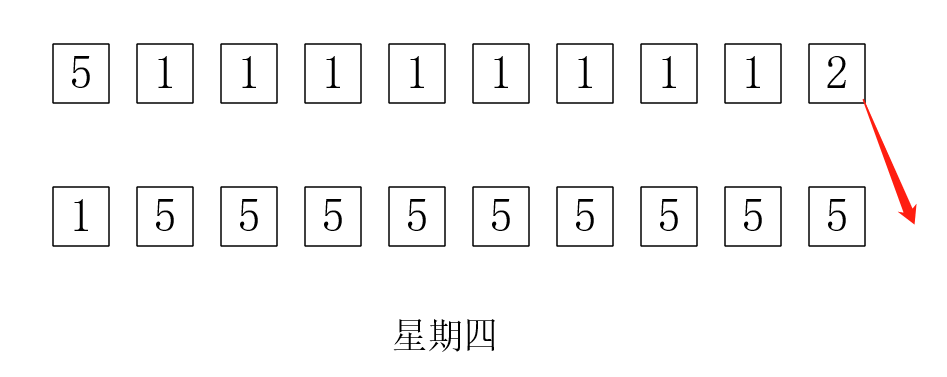
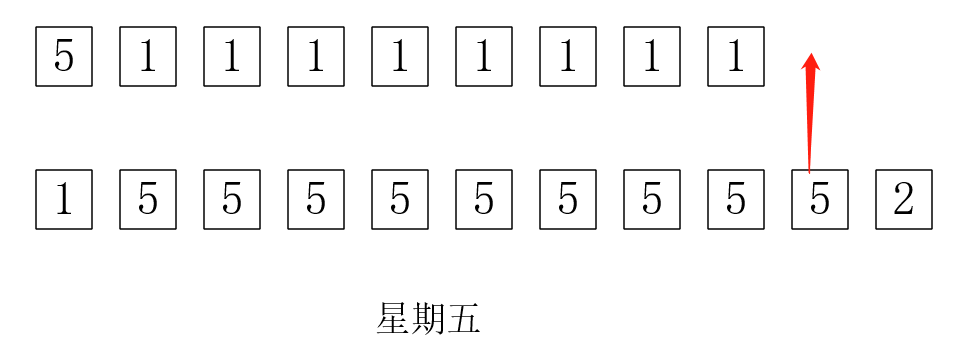
1 #include <cstdio> 2 #include <iostream> 3 #include <set> 4 using namespace std; 5 const int maxn = 1e4; 6 int a[15], b[15]; 7 set<int> ans; 8 9 int main(){ 10 for(int i = 0; i < 10; i++) cin >> a[i]; 11 for(int i = 0; i < 10; i++) cin >> b[i]; 12 13 ans.insert(1000); //第一种情况 14 int d1, d2, t; 15 for(int i = 0; i < 10; i++){ 16 for(int j = 0; j < 10; j++){ 17 d1 = b[j]-a[i]; //差值 18 19 //交换桶 20 t = a[i]; 21 a[i] = b[j]; 22 b[j] = t; 23 24 25 for(int k = 0; k < 10; k++){ 26 for(int p = 0; p < 10; p++){ 27 d2 = b[p]-a[k]; //第二次不用交换, 直接算差值 28 ans.insert(1000+d1+d2); //第三种情况 29 } 30 } 31 ans.insert(1000+d1); //第二种情况 32 33 //换回来 34 t = a[i]; 35 a[i] = b[j]; 36 b[j] = t; 37 38 } 39 } 40 41 cout << ans.size() << endl; 42 return 0; 43 }