- How to make a Windows Phone 8 app with Windows Azure Mobile Service
Windows Azure Mobile Service推出已经有一段时间了,这可能是这段时间以来Windows Azure上最受关注的一个Feature,试用了一下Mobile Service,觉得还不错,分享下试用的过程,供大家参考。
新建一个Mobile Service
1. 登陆到Windows Azure Portal,创建一个Mobile Service
2. 创建一个Mobile Service:MyTodos,并为之新建一个数据库,注意数据库服务器的区域和Mobile Service的区域最好相同,这样可以减少网络延迟和流量费用 (当然也可以使用已有的数据库实例,Mobile Service会把新建的数据表都放到MyTodos这个Schema下,所以不用担心和原有的表重名)
3. 等待几分钟后,Mobile Service创建完成后,点击MyTodos进行配置:
a) 平台这里可以看到,Mobile Service支持Windows/ WP8/Android/iOS/Html5的应用,这里我们选择Windows Phone8,注意需要安装两个SDK – Windows Phone 8 SDK和Mobile Service SDK;
b) 单击“创建TodoItem表”在刚才的数据库中创建一个TodoItem表,当然也可以自己到数据库里创建。
4. 浏览到配置这个页面,注意启用“动态架构”,这样在开发阶段,我们给Mobile Service传什么样的entity,Mobile Service会自动为我们创建相应的字段,但等到发布阶段,出于安全的考虑,最好是把“动态架构”关闭。
5. 回到MyTodos的首页,下载Azure自动生成的代码
6. 打开刚下载的代码,这时候我们已经得到了一个完整的Windows Phone 8的应用,来看看Mobile Service都生成了什么代码
a) MobileServiceClient:这就是Mobile Service SDK里面用来访问Mobile Service的客户端
b) TodoItem以及相应的Insert/Update/Refresh等方法:注意这些方法都是使用.Net 4.5的异步编程,这也是Mobile Service所推荐的编程模式。
7. 运行测试一下这个小App,添加一些TodoItem,浏览之前建的数据库,可以看到,还没有写一行代码,就已经得到了一个完整的WP8 App。
添加用户验证
接下来添加用户验证,Mobile Service支持MicrosoftAccount(也就是LiveID)/Google/Facebook/Twritter四种用户验证方式,本文只使用了MicrosoftAccount。
1. 要使用MicrosofAccount,首先要到Live Connect Developer Center(http://go.microsoft.com/fwlink/p/?linkid=262039&clcid=0x409 )注册一下我们的App,注意“手机客户端应用”选择“是”。
2. 将注册得到的客户端ID和客户端密钥填到Mobile Service上,并保存
3. 现在设置一下数据权限,将TodoItem表设为只有验证过的用户才能读写,并保存
4. 再运行一下App,这次应该收到一个Unauthorized异常,因为当前的用户没有登录
5. 接下来为App添加登录的代码
a) 在MainPage.xaml里为MainPage添加一个Login button
b) 注释掉OnNavigatedTo里面的代码

protected override void OnNavigatedTo(NavigationEventArgs e) { //RefreshTodoItems(); }
c) 修改App.xaml.cs里面的ApplicationKey

public static MobileServiceClient MobileService = new MobileServiceClient( "https://mytodos.azure-mobile.net/", "用注册app时得到的客户端密钥" );
d) 将下面这段代码添加到MainPage class里

private MobileServiceUser user; private async System.Threading.Tasks.Task Authenticate() { if (user == null) { string message = "You must log in. Login Required"; try { user = await App.MobileService.LoginAsync (MobileServiceAuthenticationProvider.MicrosoftAccount); message = string.Format("You are now logged in - {0}", user.UserId); } catch (InvalidOperationException ex) { message = ex.Message; } MessageBox.Show(message); } } private async void BtnLogin_Click(object sender, RoutedEventArgs e) { await Authenticate(); if (null != this.user) { RefreshTodoItems(); } }
运行看看效果,现在又可以刷新和添加TodoItem了
6. 如果需要得到更多的用户信息,则需要下载并安装LiveSDK( http://msdn.microsoft.com/en-us/live/ff621310 ),并为MyTodos添加引用到Microsoft.Live
a) 按下面的代码修改MainPage.Authenticate

private async System.Threading.Tasks.Task Authenticate() { if (user == null) { string message = "You must log in. Login Required"; try { string clientId = "用注册app时得到的客户端ID"; LiveAuthClient liveIdClient = new LiveAuthClient(clientId); LiveLoginResult result = await liveIdClient.LoginAsync(new[] { "wl.basic", "wl.photos" }); if (result.Status == LiveConnectSessionStatus.Connected) { LiveConnectClient client = new LiveConnectClient(result.Session); var me = await client.GetAsync("me"); user = await App.MobileService.LoginAsync(result.Session.AuthenticationToken); //(MobileServiceAuthenticationProvider.MicrosoftAccount); this.TitleTextBlock.Text = this.TitleTextBlock.Text + " - welcome, " + me.Result["first_name"].ToString() + "." + me.Result["last_name"].ToString(); message = string.Format("You are now logged in - {0}", user.UserId); } else { message = string.Format("Connect to microsoft account failed. Status: {0}", result.Status); } } catch (LiveAuthException authEx) { message = string.Format("{0}:{1}", authEx.GetType().Name, HttpUtility.UrlDecode(authEx.Message)); } catch (LiveConnectException connEx) { message = string.Format("{0}:{1}", connEx.GetType().Name, HttpUtility.UrlDecode(connEx.Message)); } catch (InvalidOperationException ex) { message = ex.Message; } MessageBox.Show(message); } }
运行效果如下,可以看到登录的页面与之前稍有差别,但这次能得到更多的用户信息
添加推送通知
1. 添加代码如下
a) 在App.xaml.cs中添加如下代码

public static HttpNotificationChannel CurrentChannel { get; private set; } private static readonly string ChannelName = "MyTodosPushChannel"; private void AcquirePushChannel() { CurrentChannel = HttpNotificationChannel.Find(ChannelName); if (CurrentChannel == null) { CurrentChannel = new HttpNotificationChannel(ChannelName); CurrentChannel.Open(); CurrentChannel.BindToShellTile(); CurrentChannel.BindToShellToast(); } CurrentChannel.ShellToastNotificationReceived += CurrentChannel_ShellToastNotificationReceived; } void CurrentChannel_ShellToastNotificationReceived(object sender, NotificationEventArgs e) { string msg = ""; foreach (var kv in e.Collection) { msg = msg + "\r\n" + kv.Key.ToString() + ": " + kv.Value; } Deployment.Current.Dispatcher.BeginInvoke(() => { MessageBox.Show(msg); }); }
b) 在Application_Launching中调用AcquirePushChannel

private void Application_Launching(object sender, LaunchingEventArgs e) { AcquirePushChannel(); }
c) 给TodoItem class添加一个property

[DataMember(Name = "channel")] public string Channel { get; set; }
d) 修改MainPage.xaml.cs的ButtonSave_Click,代码如下。由于我们启用的动态架构,所以Save的时候Mobile Service会为新添加的Channel属性创建一个对应的数据库字段。

private void ButtonSave_Click(object sender, RoutedEventArgs e) { var todoItem = new TodoItem { Text = TodoInput.Text, Channel = App.CurrentChannel.ChannelUri.ToString() }; InsertTodoItem(todoItem); }
2. 给App开启推送
3. 回到Azure的Portal,修改Mobile Service服务端script并保存,详细参数可以参考这里:
http://msdn.microsoft.com/en-us/library/windowsazure/jj871025.aspx
代码如下:

function insert(item, user, request) { request.execute({ success: function () { request.respond(); // send flip tile push.mpns.sendFlipTile(item.channel, { title: item.text }, { success: function (pushResponse) { console.log("Sent flip tile push:", pushResponse); } }); // send toast push.mpns.sendToast(item.channel, { text1: 'MyTodos', text2: item.text }, { success: function(pushResponse){ console.log("Sent toast push:", pushResponse); } }); } }); }
4. 把MyTodos pin到主界面上,运行app,观察效果
总结
Azure Mobile Service极大地简化了server端的代码开发,尤其是对于比较小的应用来说,更是非常方便,使App开发者可以专注于前端代码。
附:
客户端源代码在这里
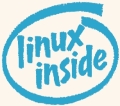