题目一
题目一:不分行从上到下打印二叉树
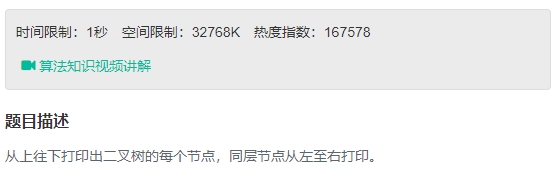
分析
不分行从上到下打印二叉树
源码
public class Solution {
public ArrayList<Integer> PrintFromTopToBottom(TreeNode root) {
ArrayList<Integer> result = new ArrayList<>();
if (root==null){
return result;
}
Queue<TreeNode> queue = new LinkedList<>();
queue.offer(root);
while (queue.size()>0){
TreeNode first = queue.poll();
result.add(first.val);
if(first.left!=null){
queue.offer(first.left);
}
if(first.right!=null){
queue.offer(first.right);
}
}
return result;
}
}
题目二
题目二:分行从上到下打印二叉树

分析
分行从上到下打印二叉树
源码
import java.util.ArrayList;
import java.util.*;
public class Solution {
ArrayList<ArrayList<Integer> > Print(TreeNode pRoot) {
ArrayList<ArrayList<Integer>> arrayLists = new ArrayList<>();
if(pRoot==null){
return arrayLists;
}
Queue<TreeNode> queue = new LinkedList<>();
queue.offer(pRoot);
int printLeft=1;
int toBePrint=0;
ArrayList<Integer> result = new ArrayList<>();
while (!queue.isEmpty()){
TreeNode temp = queue.poll();
if(temp.left!=null){
queue.offer(temp.left);
toBePrint++;
}
if(temp.right!=null){
queue.offer(temp.right);
toBePrint++;
}
if(printLeft>0){
result.add(temp.val);
printLeft--;
}
if(printLeft<=0){
printLeft=toBePrint;
toBePrint=0;
arrayLists.add(result);
result = new ArrayList<>();
}
}
return arrayLists;
}
}
题目三
题目:之字形打印二叉树
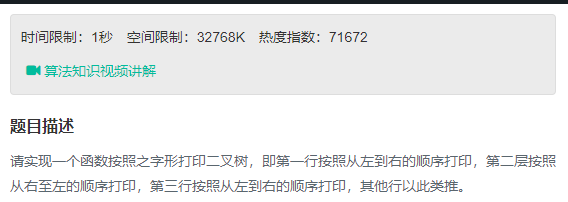
分析
之字形打印二叉树
源码
import java.util.*;
public class Solution {
public ArrayList<ArrayList<Integer> > Print(TreeNode pRoot) {
ArrayList<ArrayList<Integer>> lists = new ArrayList<>();
if(pRoot==null){
return lists;
}
Stack<TreeNode> stack1 = new Stack<>();//奇数层使用的栈
Stack<TreeNode> stack2 = new Stack<>();//偶数层使用的栈
int cur=0;
int next=1;
stack1.push(pRoot);
while (!stack1.isEmpty()||!stack2.isEmpty()){
if(cur==0&&!stack1.isEmpty()){
ArrayList<Integer> list = new ArrayList<>();
while (!stack1.isEmpty()){
TreeNode temp=stack1.pop();
list.add(temp.val);
if(temp.left!=null){
stack2.push(temp.left);
}
if(temp.right!=null){
stack2.push(temp.right);
}
}
lists.add(list);
}
else if (cur==1&&!stack2.isEmpty()){
ArrayList<Integer> list = new ArrayList<>();
while (!stack2.isEmpty()){
TreeNode temp=stack2.pop();
list.add(temp.val);
if(temp.right!=null){
stack1.push(temp.right);
}
if(temp.left!=null){
stack1.push(temp.left);
}
}
lists.add(list);
}
cur=1-cur;
next=1-next;
}
return lists;
}
}