设计模式-装饰模式(08)
定义
装饰模式的英文原话是:Attach additional responsibilities to an object dynamically keeping the same interface.Decorators provide a flexible alternative to subclass for extending functionality.意思是:动态的给一个对象添加一些额外的职责。就增强功能来说,装饰模式相比于代理模式生成子类更为灵活。
装饰模式有四种角色:
抽象构建(Component)角色:该角色用于规范需要装饰的对象(原始对象)。
具体构建(Concrete Component)角色:该角色实现抽象构件接口,定义一个需要装饰的原始类。
装饰角色(Decorator)角色:该角色持有一个构建对象的实例,并定义一个与抽象构建接口一致的接口。
具体装饰模式(Concrete Decorator)角色:该角色负责对构建对象进行装饰。
/** * 抽象构建 * 原始对象接口 */ public interface Component { public void operation(); } /** * 具体构建 * 原始对象类 */ public class ConcreteComponent implements Component{ @Override public void operation() { //业务代码 System.out.println("原始对象业务代码"); } } /** * 装饰类角色 * 抽象类 */ public abstract class Decorator implements Component { private Component component = null; public Decorator(Component component) { this.component = component; } @Override public void operation() { this.component.operation(); } } /** * 具体修饰 */ public class ConcreteDecorator extends Decorator { public ConcreteDecorator(Component component) { super(component); } //装饰类添加的方法 private void addmethod(){ System.out.println("Decorator添加的方法"); } //重写operation方法 public void operation(){ this.addmethod(); super.operation(); } } //调用 public class DecoratorDemo { public static void main(String[] args) { Component component = new ConcreteComponent(); component.operation(); System.out.println("经过装饰----------"); component = new ConcreteDecorator(component); component.operation(); } }
装饰模式的优点
- 装饰类和被装饰类可以独立发展,而不会相互耦合,即Component类无须知道Decorator类,Decorator类从外部扩展Component类的功能,而Decorator不用知道具体的构建。
- 装饰模式是继承关系的一个替代方案。装饰类Decorator不管装饰多少层,返回的对象还是Component。
- 装饰模式可以动态地扩展一个实现类的功能。
装饰模式的缺点
多层的装饰比较复杂
装饰模式使用场景
- 需要扩展一个类的功能,或给一个类增加附加功能。
- 需要动态地给一个对象增加功能,这些功能可以动态地撤销。
- 需要为一批类进行改装或加装功能。
装饰模式是对继承的有效补充。单纯使用继承时,在某些情况下回增加很多子类,而且灵活性差,也不容易维护。装饰模式可以替代继承,解决类膨胀的问题,如java基础类库中的与输入输出流相关的类大量使用了装饰模式。
如果这篇文章对你有用,可以关注本人微信公众号获取更多ヽ(^ω^)ノ ~
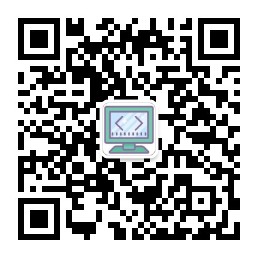