这里讲的网络程序的开发,主要是在程序中连接网络,在CF中UDP与TCP与Windows 应用程序相差不多.
设置
mobile要联接网络,先觉条件是要有一个拔号设置,移动拔号设置分为cmwap,cmnet.这个设置非常的重要,cmwap只支持http而不支持tcp和udp,因为这个原因我曾经就花费过许多时间.
开始-->设置-->连接(选项卡)—>连接(按钮)—>高级-->选择网络 在这里你可以选择一个已经有的网络,或者新建一个网络.
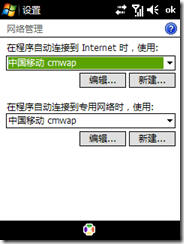
新建连接
1点击新建
2在常规选项卡里输入连接的名字
3在调制解调器选项卡点击新建,弹出新建对话框
4输入新建连接名字,选择电话线路(gprs),点击下一步
5在访问点的名字输入cmwap,或者cmnet 选择下一步
6用户名密码不用填 ,完成连接
在使用时只要选择即可。
具体的设置可以打10086,或者其它运营商咨询。
程序拔号
如果webservice,程序会在没有连接网络时自动连接。但在开发UDP、TCP网络程序时,经常要考虑到是否连接到网络,如果没有则需要程序拔号。现在已经有开源代码实现这个功能。主要有两个类ConnectManager.cs,GPRSManage.cs 这两个人实现方式差不多,都是调用系统方法。
在使用的时候这样调用就可以了。

Code
1
using System;
2
using System.Collections.Generic;
3
using System.Text;
4
using System.Runtime.InteropServices;
5
using System.Threading;
6
using System.Collections;
7
8
namespace Connection
9

{
10
public class ConnectManager
11
{
12
const int S_OK = 0;
13
const uint CONNMGR_PARAM_GUIDDESTNET = 0x1;
14
const uint CONNMGR_PRIORITY_USERINTERACTIVE = 0x08000;
15
const uint INFINITE = 0xffffffff;
16
const uint CONNMGR_STATUS_CONNECTED = 0x10;
17
const int CONNMGR_MAX_DESC = 128; // @constdefine Max size of a network description
18
19
const int CONNMGR_FLAG_PROXY_HTTP = 0x1; // @constdefine HTTP Proxy supported
20
const int CONNMGR_FLAG_PROXY_WAP = 0x2; // @constdefine WAP Proxy (gateway) supported
21
const int CONNMGR_FLAG_PROXY_SOCKS4 = 0x4; // @constdefine SOCKS4 Proxy supported
22
const int CONNMGR_FLAG_PROXY_SOCKS5 = 0x8; // @constdefine SOCKS5 Proxy supported
23
24
const UInt16 IDC_WAIT = 32514;
25
const UInt16 IDC_ARROW = 32512;
26
27
private IntPtr m_hConnection = IntPtr.Zero;
28
29
public ConnectManager()
30
{
31
}
32
33
~ConnectManager()
34
{
35
ReleaseConnection();
36
}
37
38
/**//// <summary>
39
/// 查看连接是否可用
40
/// </summary>
41
/// <returns></returns>
42
public bool GetConnMgrAvailable()
43
{
44
IntPtr hConnMgr = ConnMgrApiReadyEvent();
45
bool bAvailbale = false;
46
uint dwResult = WaitForSingleObject ( hConnMgr, 2000 );
47
48
if (dwResult == 0)
49
{
50
bAvailbale = true;
51
}
52
53
// 关闭
54
if (hConnMgr.ToInt32() != 0) CloseHandle(hConnMgr);
55
56
return bAvailbale;
57
}
58
/**//// <summary>
59
/// 映射URL
60
/// </summary>
61
/// <param name="lpszURL"></param>
62
/// <param name="guidNetworkObject"></param>
63
/// <param name="pcsDesc"></param>
64
/// <returns></returns>
65
public int MapURLAndGUID(string lpszURL, ref GUID guidNetworkObject, ref string pcsDesc)
66
{
67
if (lpszURL == null || lpszURL.Length < 1)
68
return 0;
69
70
uint nIndex = 0;
71
int hResult = ConnMgrMapURL(lpszURL,ref guidNetworkObject, ref nIndex);
72
if (hResult < 0)
73
{
74
throw new Exception("Could not map a request to a network identifier");
75
}
76
else
77
{
78
if (pcsDesc != null)
79
{
80
CONNMGR_DESTINATION_INFO DestInfo = new CONNMGR_DESTINATION_INFO();
81
if (ConnMgrEnumDestinations((int)nIndex, ref DestInfo) >= 0)
82
{
83
pcsDesc = DestInfo.szDescription;
84
}
85
}
86
}
87
88
return (int)nIndex;
89
}
90
/**//// <summary>
91
/// 枚举网络标识符信息
92
/// </summary>
93
/// <param name="lstNetIdentifiers"></param>
94
public List<CONNMGR_DESTINATION_INFO> EnumNetIdentifier()
95
{
96
List<CONNMGR_DESTINATION_INFO> lstNetIdentifiers = new List<CONNMGR_DESTINATION_INFO>();
97
// 得到网络列表
98
for (uint dwEnumIndex = 0; ; dwEnumIndex++)
99
{
100
CONNMGR_DESTINATION_INFO networkDestInfo = new CONNMGR_DESTINATION_INFO();
101
102
if (ConnMgrEnumDestinations((int)dwEnumIndex,ref networkDestInfo) != 0)
103
{
104
break;
105
}
106
lstNetIdentifiers.Add(networkDestInfo);
107
}
108
109
return lstNetIdentifiers;
110
}
111
112
/**//// <summary>
113
/// 建立连接
114
/// </summary>
115
/// <param name="nIndex"></param>
116
/// <returns></returns>
117
public bool EstablishConnection(uint nIndex, uint dwStatus)
118
{
119
ReleaseConnection();
120
// 得到正确的连接信息
121
CONNMGR_DESTINATION_INFO DestInfo = new CONNMGR_DESTINATION_INFO();
122
int hResult = ConnMgrEnumDestinations((int)nIndex, ref DestInfo);
123
124
if (hResult >= 0)
125
{
126
// 初始化连接结构
127
CONNMGR_CONNECTIONINFO ConnInfo = new CONNMGR_CONNECTIONINFO();
128
129
ConnInfo.cbSize = (uint)Marshal.SizeOf(ConnInfo);
130
ConnInfo.dwParams = CONNMGR_PARAM_GUIDDESTNET;
131
ConnInfo.dwFlags = CONNMGR_FLAG_PROXY_HTTP | CONNMGR_FLAG_PROXY_WAP | CONNMGR_FLAG_PROXY_SOCKS4 | CONNMGR_FLAG_PROXY_SOCKS5;
132
ConnInfo.dwPriority = CONNMGR_PRIORITY_USERINTERACTIVE;
133
ConnInfo.guidDestNet = DestInfo.guid;
134
ConnInfo.bExclusive = 0;
135
ConnInfo.bDisabled = 0;
136
137
//uint dwStatus = 0;
138
hResult = ConnMgrEstablishConnectionSync(ref ConnInfo, ref m_hConnection, 15 * 1000, ref dwStatus);
139
if (hResult < 0)
140
{
141
return false;
142
}
143
else
144
{
145
return true;
146
}
147
}
148
149
return false;
150
}
151
152
/**//// <summary>
153
/// 建立连接
154
/// </summary>
155
/// <param name="nIndex"></param>
156
/// <returns></returns>
157
public bool EstablishConnection(GUID guid, uint dwStatus)
158
{
159
ReleaseConnection();
160
// 得到正确的连接信息
161
162
// 初始化连接结构
163
CONNMGR_CONNECTIONINFO ConnInfo = new CONNMGR_CONNECTIONINFO();
164
165
ConnInfo.cbSize = (uint)Marshal.SizeOf(ConnInfo);
166
ConnInfo.dwParams = CONNMGR_PARAM_GUIDDESTNET;
167
ConnInfo.dwFlags = CONNMGR_FLAG_PROXY_HTTP | CONNMGR_FLAG_PROXY_WAP | CONNMGR_FLAG_PROXY_SOCKS4 | CONNMGR_FLAG_PROXY_SOCKS5;
168
ConnInfo.dwPriority = CONNMGR_PRIORITY_USERINTERACTIVE;
169
ConnInfo.guidDestNet = guid;
170
ConnInfo.bExclusive = 0;
171
ConnInfo.bDisabled = 0;
172
173
//uint dwStatus = 0;
174
int hResult = ConnMgrEstablishConnectionSync(ref ConnInfo, ref m_hConnection, 15 * 1000, ref dwStatus);
175
if (hResult < 0)
176
{
177
return false;
178
}
179
else
180
{
181
return true;
182
}
183
184
185
}
186
187
/**//// <summary>
188
/// 连接状态
189
/// </summary>
190
/// <param name="nTimeoutSec"></param>
191
/// <param name="pdwStatus"></param>
192
/// <returns></returns>
193
public bool WaitForConnected(int nTimeoutSec, ref uint pdwStatus)
194
{
195
uint dwStartTime = GetTickCount();
196
bool bRet = false;
197
198
while (GetTickCount() - dwStartTime < (uint)nTimeoutSec * 1000)
199
{
200
if (m_hConnection.ToInt32() != 0)
201
{
202
uint dwStatus = 0;
203
int hr = ConnMgrConnectionStatus(m_hConnection, ref dwStatus);
204
if (dwStatus != 0) pdwStatus = dwStatus;
205
if (hr >= 0)
206
{
207
if (dwStatus == CONNMGR_STATUS_CONNECTED)
208
{
209
bRet = true;
210
break;
211
}
212
}
213
}
214
Thread.Sleep(100);
215
}
216
217
return bRet;
218
}
219
220
/**//// <summary>
221
/// 释放所有连接
222
/// </summary>
223
public void ReleaseConnection()
224
{
225
226
if (m_hConnection.ToInt32() != 0)
227
{
228
ConnMgrReleaseConnection(m_hConnection, 0);
229
m_hConnection = IntPtr.Zero;
230
}
231
}
232
233
[StructLayout(LayoutKind.Sequential)]
234
public struct CONNMGR_CONNECTIONINFO
235
{
236
public uint cbSize;
237
public uint dwParams;
238
public uint dwFlags;
239
public uint dwPriority;
240
public int bExclusive;
241
public int bDisabled;
242
public GUID guidDestNet;
243
public IntPtr hWnd;
244
public uint uMsg;
245
public uint lParam;
246
public uint ulMaxCost;
247
public uint ulMinRcvBw;
248
public uint ulMaxConnLatency;
249
}
250
251
[StructLayout(LayoutKind.Sequential, CharSet = CharSet.Unicode)]
252
public struct CONNMGR_DESTINATION_INFO
253
{
254
public GUID guid; // @field GUID associated with network
255
[MarshalAs(UnmanagedType.ByValTStr,SizeConst = CONNMGR_MAX_DESC)]
256
public string szDescription; // @field Description of network
257
public int fSecure; // @field Is it OK to allow multi-homing on the network
258
}
259
260
public struct GUID
261
{ // size is 16
262
public uint Data1;
263
public UInt16 Data2;
264
public UInt16 Data3;
265
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 8)]
266
public byte[] Data4;
267
}
268
269
[DllImport("coredll.dll")]
270
public static extern uint GetTickCount();
271
272
[DllImport("coredll.dll")]
273
public static extern uint WaitForSingleObject(IntPtr hHandle,uint dwMilliseconds);
274
275
[DllImport("cellcore.dll")]
276
public static extern int ConnMgrMapURL(string pwszURL, ref GUID pguid, ref uint pdwIndex);
277
278
[DllImport("cellcore.dll", CharSet = CharSet.Unicode, SetLastError = true)]
279
unsafe static extern int ConnMgrMapURL(string url, ref Guid guid, int* nIndex);
280
281
[DllImport("cellcore.dll")]
282
public static extern int ConnMgrEstablishConnectionSync(ref CONNMGR_CONNECTIONINFO ci, ref IntPtr phConnection, uint dwTimeout, ref uint pdwStatus);
283
284
[DllImport("cellcore.dll")]
285
private static extern IntPtr ConnMgrApiReadyEvent();
286
287
[DllImport("cellcore.dll")]
288
public static extern int ConnMgrReleaseConnection(IntPtr hConnection, int bCache);
289
290
[DllImport("cellcore.dll")]
291
public static extern int ConnMgrEnumDestinations(int nIndex,ref CONNMGR_DESTINATION_INFO pDestInfo);
292
293
[DllImport("cellcore.dll")]
294
public static extern int ConnMgrConnectionStatus(IntPtr hConnection, // @parm Handle to connection, returned from ConnMgrEstablishConnection
295
ref uint pdwStatus // @parm Returns current connection status, one of CONNMGR_STATUS_*
296
);
297
298
[DllImport("coredll.dll")]
299
private static extern int CloseHandle(IntPtr hObject);
300
}
301
}
302

Code
1
using System;
2
//using System.Collections.Generic;
3
//using System.ComponentModel;
4
//using System.Data;
5
using System.Runtime.InteropServices;
6
using System.Collections;
7
//using System.Net.Sockets;
8
//using System.Windows.Forms;
9
10
namespace GPRSmanage
11

{
12
13
/**//// <summary>
14
/// GPRS连接类
15
/// </summary>
16
public class GPRSmanage
17
{
18
const int CONNMGR_PARAM_GUIDDESTNET = 0x1; // guidDestNet -
19
const int CONNMGR_PARAM_MAXCOST = 0x2; // ulMaxCost -
20
const int CONNMGR_PARAM_MINRCVBW = 0x4; // ulMinRcvBw -
21
const int CONNMGR_PARAM_MAXCONNLATENCY = 0x8;
22
const int CONNMGR_FLAG_PROXY_HTTP = 0x1; // HTTP Proxy
23
const int CONNMGR_FLAG_PROXY_WAP = 0x2; // WAP Proxy (gateway)
24
const int CONNMGR_FLAG_PROXY_SOCKS4 = 0x4; // SOCKS4 Proxy
25
const int CONNMGR_FLAG_PROXY_SOCKS5 = 0x8; // SOCKS5 Proxy
26
const int CONNMGR_PRIORITY_VOICE = 0x20000; //
27
const int CONNMGR_PRIORITY_USERINTERACTIVE = 0x08000; //
28
const int CONNMGR_PRIORITY_USERBACKGROUND = 0x02000; //
29
const int CONNMGR_PRIORITY_USERIDLE = 0x00800; //
30
const int CONNMGR_PRIORITY_HIPRIBKGND = 0x00200; //
31
const int CONNMGR_PRIORITY_IDLEBKGND = 0x00080; //
32
const int CONNMGR_PRIORITY_EXTERNALINTERACTIVE = 0x00020; //
33
const int CONNMGR_PRIORITY_LOWBKGND = 0x00008; //
34
const int CONNMGR_PRIORITY_CACHED = 0x00002; //
35
36
37
const int CONNMGR_STATUS_UNKNOWN = 0x00; //
38
const int CONNMGR_STATUS_CONNECTED = 0x10; //
39
const int CONNMGR_STATUS_DISCONNECTED = 0x20; //
40
const int CONNMGR_STATUS_CONNECTIONFAILED = 0x21; //
41
const int CONNMGR_STATUS_CONNECTIONCANCELED = 0x22; //
42
const int CONNMGR_STATUS_CONNECTIONDISABLED = 0x23; //
43
const int CONNMGR_STATUS_NOPATHTODESTINATION = 0x24; //
44
const int CONNMGR_STATUS_WAITINGFORPATH = 0x25; //
45
const int CONNMGR_STATUS_WAITINGFORPHONE = 0x26; //
46
const int CONNMGR_STATUS_WAITINGCONNECTION = 0x40; //
47
const int CONNMGR_STATUS_WAITINGFORRESOURCE = 0x41; //
48
const int CONNMGR_STATUS_WAITINGFORNETWORK = 0x42; //
49
const int CONNMGR_STATUS_WAITINGDISCONNECTION = 0x80; //
50
const int CONNMGR_STATUS_WAITINGCONNECTIONABORT = 0x81; //
51
52
53
unsafe class CONNMGR_CONNECTIONINFO
54
{
55
public int cbSize = 0x40;
56
public int dwParams = 0;
57
public int dwFlags = 0;
58
public int dwPriority = 0;
59
public int bExclusive = 0;
60
public int bDisabled = 0;
61
public Guid guidDestNet = Guid.Empty;
62
public IntPtr hWnd = IntPtr.Zero;
63
public int uMsg = 0; // ID Message
64
public int lParam = 0; // lParam
65
public int ulMaxCost = 0;
66
public int ulMinRcvBw = 0;
67
public int ulMaxConnLatency = 0;
68
}
69
70
unsafe class CONNMGR_DESTINATION_INFO
71
{
72
public Guid guid = Guid.Empty;
73
public char* szDescription = null;
74
}
75
76
[DllImport("cellcore.dll", CharSet = CharSet.Unicode, SetLastError = true)]
77
unsafe static extern int ConnMgrEnumDestinations(int nIndex, CONNMGR_DESTINATION_INFO destinationInfo);
78
79
[DllImport("cellcore.dll", CharSet = CharSet.Unicode, SetLastError = true)]
80
unsafe static extern int ConnMgrMapURL(string url, ref Guid guid, int* nIndex);
81
82
[DllImport("cellcore.dll", CharSet = CharSet.Unicode, SetLastError = true)]
83
unsafe static extern int ConnMgrEstablishConnection(CONNMGR_CONNECTIONINFO pConnInfo, out IntPtr hConnection);
84
85
[DllImport("cellcore.dll", CharSet = CharSet.Unicode, SetLastError = true)]
86
unsafe static extern int ConnMgrEstablishConnectionSync(CONNMGR_CONNECTIONINFO pConnInfo, out IntPtr hConn, uint timeout, uint* status);
87
88
[DllImport("cellcore.dll", CharSet = CharSet.Unicode, SetLastError = true)]
89
unsafe static extern int ConnMgrConnectionStatus(IntPtr hConnection, uint* status);
90
91
[DllImport("cellcore.dll", CharSet = CharSet.Unicode, SetLastError = true)]
92
unsafe static extern int ConnMgrReleaseConnection(IntPtr hConnection, int bCache);
93
94
[DllImport("coredll.dll")]
95
private static extern int CloseHandle(IntPtr hObject);
96
97
98
public static Hashtable ht = new Hashtable();
99
public IntPtr hConnect;
100
public static unsafe Guid GuidConnection(int nIndex)
101
{
102
Guid guid = Guid.Empty;
103
CONNMGR_DESTINATION_INFO connMgrDestinationInfo = new CONNMGR_DESTINATION_INFO();
104
fixed (char* pDescription = new char[129])
105
{
106
connMgrDestinationInfo.szDescription = pDescription;
107
if (ConnMgrEnumDestinations(nIndex, connMgrDestinationInfo) == 0)
108
guid = new Guid(connMgrDestinationInfo.guid.ToString());
109
}
110
return guid;
111
}
112
public static unsafe Guid GuidConnectionURL(string url)
113
{
114
Guid guid = Guid.Empty;
115
int result = ConnMgrMapURL(url, ref guid, null);
116
if (result != 0) throw new Exception("ConnMgrMapURL()");
117
return guid;
118
}
119
120
public static unsafe IntPtr EstablishConnection(Guid guid)
121
{
122
CONNMGR_CONNECTIONINFO connectionInfo = new CONNMGR_CONNECTIONINFO();
123
connectionInfo.dwParams = CONNMGR_PARAM_GUIDDESTNET;
124
connectionInfo.dwPriority = CONNMGR_PRIORITY_USERINTERACTIVE;
125
connectionInfo.guidDestNet = guid;
126
connectionInfo.bExclusive = 1;
127
IntPtr hConnection = IntPtr.Zero;
128
int result = ConnMgrEstablishConnection(connectionInfo, out hConnection);
129
if (result != 0) throw new Exception("ConnMgrEstablishConnection()");
130
return hConnection;
131
}
132
public static unsafe IntPtr EstablishConnection_noConnect(Guid guid)
133
{
134
CONNMGR_CONNECTIONINFO connectionInfo = new CONNMGR_CONNECTIONINFO();
135
connectionInfo.dwParams = CONNMGR_PARAM_GUIDDESTNET;
136
connectionInfo.dwPriority = CONNMGR_PRIORITY_USERINTERACTIVE;
137
connectionInfo.guidDestNet = guid;
138
connectionInfo.bDisabled = 0;
139
connectionInfo.bExclusive = 1;
140
IntPtr hConnection = IntPtr.Zero;
141
int result = ConnMgrEstablishConnection(connectionInfo, out hConnection);
142
if (result != 0) throw new Exception("ConnMgrEstablishConnection()");
143
return hConnection;
144
}
145
public static unsafe IntPtr EstablishConnectionSync(Guid guid, double timeout)
146
{
147
CONNMGR_CONNECTIONINFO connectionInfo = new CONNMGR_CONNECTIONINFO();
148
connectionInfo.dwParams = CONNMGR_PARAM_GUIDDESTNET;
149
connectionInfo.dwPriority = CONNMGR_PRIORITY_USERINTERACTIVE;
150
connectionInfo.guidDestNet = guid;
151
IntPtr hConnection = IntPtr.Zero;
152
uint tout = (uint)(timeout * 1000 + 1);
153
uint status = 0;
154
int result = ConnMgrEstablishConnectionSync(connectionInfo, out hConnection, tout, &status);
155
if (result != 0) throw new Exception("ConnMgrEstablishConnectionSync()");
156
return hConnection;
157
}
158
159
public static unsafe void ReleaseConnection(IntPtr hConnection)
160
{
161
int result = ConnMgrReleaseConnection(hConnection, 0);
162
if (result != 0) throw new Exception("ConnMgrReleaseConnection()");
163
}
164
165
public void OpenGprs()
166
{
167
try
168
{
169
Guid guid = GuidConnectionURL("http://www.google.cn/");
170
hConnect = EstablishConnection(guid);
171
}
172
catch (Exception ex)
173
{
174
throw new Exception("打开GPRS错误");
175
}
176
}
177
178
public void CloseGprs()
179
{
180
try
181
{
182
Guid guid = GuidConnectionURL("http://www.google.cn/");
183
IntPtr hConnection = EstablishConnection(guid);
184
//IntPtr hConnection = EstablishConnection_noConnect(guid);
185
ReleaseConnection(hConnection);
186
//IsConnected = false;
187
}
188
catch (Exception ex)
189
{
190
throw new Exception("关闭GPRS错误,可能未存在GPRS联接");
191
}
192
}
193
194
public unsafe uint CheckGprsStatus()
195
{
196
uint status = 0;
197
198
Guid guid = GuidConnectionURL("http://www.google.cn/");
199
IntPtr hConnection = EstablishConnection_noConnect(guid);
200
System.Threading.Thread.Sleep(500);
201
int ret = ConnMgrConnectionStatus(hConnection, &status);
202
203
return status;
204
}
205
206
}
207
208
}
209
public uint ConnectMobileNetwork()
{
try
{
uint dwStatus = 0;
string csDesc = "";
ConnectManager.GUID guidNetworkObject = new ConnectManager.GUID();
int nIndex = connectManager.MapURLAndGUID("http://www.google.com.cn%22/, ref guidNetworkObject, ref csDesc);
if (nIndex >= 0)
{
connectManager.EstablishConnection(guidNetworkObject, dwStatus);
}
return dwStatus;
}
catch
{
return 0;
}
}