中国移动OneNet平台上传GPS数据JSON格式
最终目的输出
POST /devices/3225187/datapoints HTTP/1.1
api-key: R9xO5NZm6oVI4YBHvCPKEqtwYtMA
Host: api.heclouds.com
Content-Length:81
{"datastreams":[{"id":"location","datapoints":[{"value":{"lon":106,"lat":29}}]}]}
1. 使用arduino代码组合出来HTTP头
使用OneNet官方提供的Httppacket库
#include <HttpPacket.h>
HttpPacketHead packet;
void setup() {
// put your setup code here, to run once:
Serial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for Leonardo only
}
// put your main code here, to run repeatedly:
// char *p = "{\"datastreams\":[{\"id\":\"sys_time\",\"datapoints\":[{\"value\":50}]}]}";
char *p = "{\"datastreams\":[{\"id\":\"location\",\"datapoints\":[{\"value\":{\"lon\":106,\"lat\":29}}]}]}";
packet.setHostAddress("api.heclouds.com");
packet.setDevId("3225187"); //device_id
packet.setAccessKey("R9xO5NZm6oVI4YBHvCPKEqtwYtMA"); //API_KEY
// packet.setDataStreamId("<datastream_id>"); //datastream_id
// packet.setTriggerId("<trigger_id>");
// packet.setBinIdx("<bin_index>");
/*create the http message about add datapoint */
packet.createCmdPacket(POST, TYPE_DATAPOINT, p);
if (strlen(packet.content))
Serial.print(packet.content);
Serial.print(p);
Serial.println("\n");
}
2.使用JSON库合成JSON数据
#include <ArduinoJson.h>
void setup() {
Serial.begin(9600);
StaticJsonBuffer<200> jsonBuffer;
JsonObject& lon_lat = jsonBuffer.createObject();
lon_lat["lon"] = 106;
lon_lat["lat"] = 29;
JsonObject& value = jsonBuffer.createObject();
value["value"] = lon_lat;
JsonObject& id_datapoints = jsonBuffer.createObject();
id_datapoints["id"] = "location";
JsonArray& datapoints = id_datapoints.createNestedArray("datapoints");
datapoints.add(value);
JsonObject& myJson = jsonBuffer.createObject();
JsonArray& datastreams = myJson.createNestedArray("datastreams");
datastreams.add(id_datapoints);
myJson.printTo(Serial);
Serial.print("\r\n\r\n");
//格式化输出
myJson.prettyPrintTo(Serial);
char p[200];
Serial.print("\r\n---------\r\n");
int num = myJson.printTo(p,sizeof(p));
Serial.print(p);
Serial.print("\r\n============\r\n");
Serial.print(num);
}
void loop() {
// not used in this example
}
串口输出效果
{"datastreams":[{"id":"location","datapoints":[{"value":{"lon":106,"lat":29}}]}]}
{
"datastreams": [
{
"id": "location",
"datapoints": [
{
"value": {
"lon": 106,
"lat": 29
}
}
]
}
]
}
---------
{"datastreams":[{"id":"location","datapoints":[{"value":{"lon":106,"lat":29}}]}]}
============
81
3. 综合HTTP头和JSON输出需要的POST请求
#include <HttpPacket.h>
#include <ArduinoJson.h>
HttpPacketHead packet;
void setup() {
// put your setup code here, to run once:
Serial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for Leonardo only
}
//合成POST请求
StaticJsonBuffer<200> jsonBuffer;
JsonObject& lon_lat = jsonBuffer.createObject();
lon_lat["lon"] = 106;
lon_lat["lat"] = 29;
JsonObject& value = jsonBuffer.createObject();
value["value"] = lon_lat;
JsonObject& id_datapoints = jsonBuffer.createObject();
id_datapoints["id"] = "location";
JsonArray& datapoints = id_datapoints.createNestedArray("datapoints");
datapoints.add(value);
JsonObject& myJson = jsonBuffer.createObject();
JsonArray& datastreams = myJson.createNestedArray("datastreams");
datastreams.add(id_datapoints);
char p[200];
int num = myJson.printTo(p,sizeof(p));
packet.setHostAddress("api.heclouds.com");
packet.setDevId("3225187"); //device_id
packet.setAccessKey("R9xO5NZm6oVI4YBHvCPKEqtwYtMA"); //API_KEY
// packet.setDataStreamId("<datastream_id>"); //datastream_id
// packet.setTriggerId("<trigger_id>");
// packet.setBinIdx("<bin_index>");
/*create the http message about add datapoint */
packet.createCmdPacket(POST, TYPE_DATAPOINT, p);
if (strlen(packet.content))
Serial.print(packet.content);
Serial.print(p);
成功输出
POST /devices/3225187/datapoints HTTP/1.1
api-key: R9xO5NZm6oVI4YBHvCPKEqtwYtMA
Host: api.heclouds.com
Content-Length:81
{"datastreams":[{"id":"location","datapoints":[{"value":{"lon":106,"lat":29}}]}]}
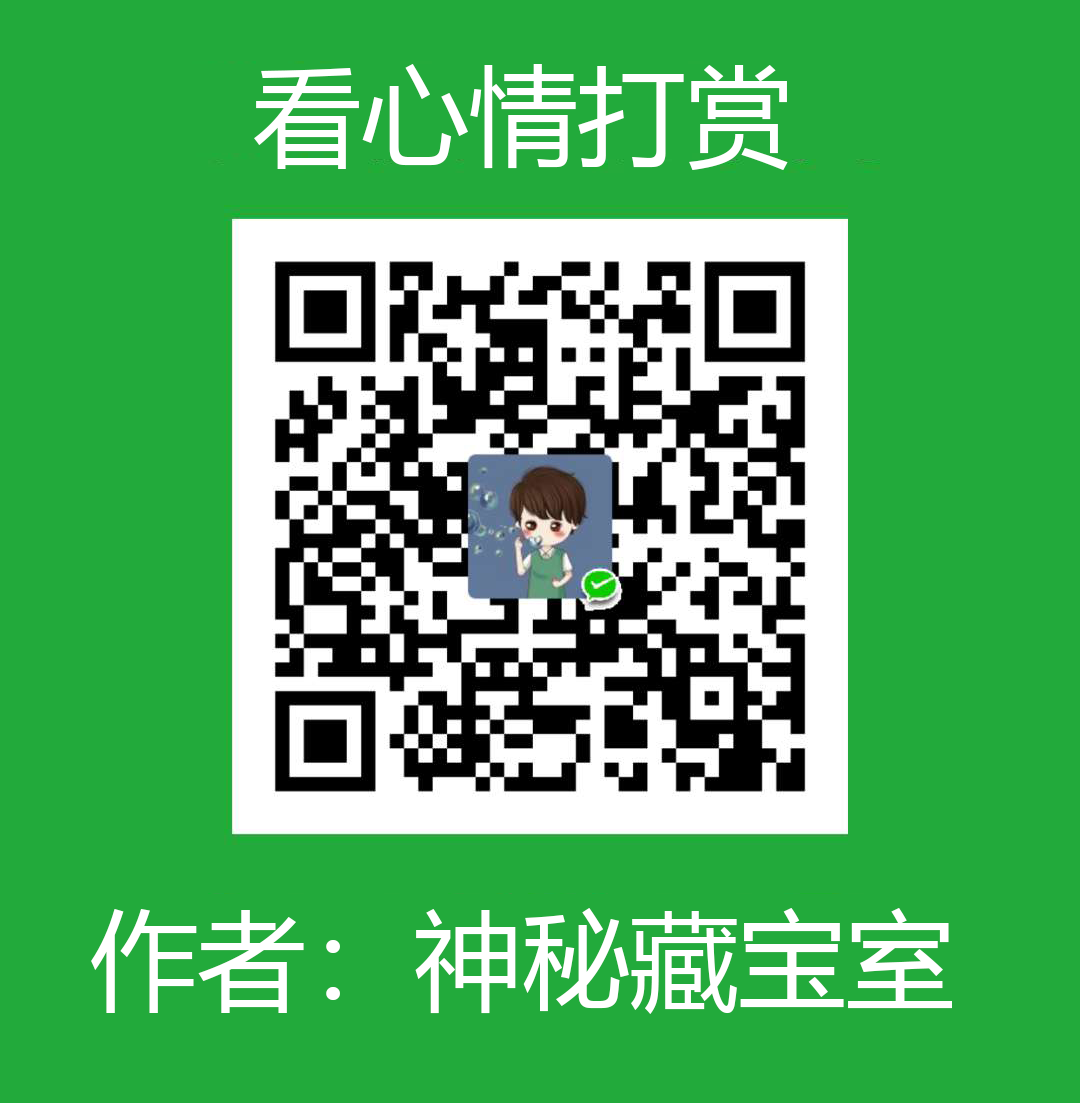
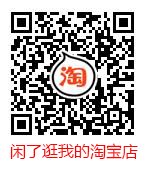
文章对您有帮助,开心可以打赏我,金额随意,欢迎来赏!
需要电子方面开发板/传感器/模块等硬件可以到我的淘宝店逛逛