《Java语言程序设计》的课程作业
前言:学习编程刚开始最合适的资料可能是一些现成的 bug-free 代码。由浅入深自己慢慢琢磨,对代码进行编译运行,到修改尝试,到改写添加新功能,到能够独立写出自己的程序。这学期选了门 Java,虽然没上过几节课,不过作业还算是马马虎虎的完成了,贴出来便于今后初学者参考。由于我初学 Java,不能保证代码的编程风格和效率,仅供参考吧。
学习 Java 一开始得确保自己的电脑上有 JRE (Java Runtime Environment),这样才能跑程序。可以去官网下一个,设置好环境变量后通过 command window 进行类 dos 命令的操作:将写好的 Java 程序保存为文本文件 .java,cd 到文件目录下之后 javac test.java(编译),生成 .class 文件。然后 java test 即可运行程序。
对于初学者更为推荐的是 eclipse,这是功能强大的集成环境,其实也不光可以用来写 Java 程序。另外在设计图形用户界面时也可以使用 NetBeans,这是类似 Visual Basic 的所见即所得的编辑软件。在 eclipse 下面新建工程等操作在网上查一下就ok。下面列出这学期的几次作业:
作业1
设计一个类,要求有一些基本属性及两个以上的方法。(可从日常生活中抽取)在类中加入2个不同参数的构造方法。再设计一个类,包含main方法,对上面的类进行应用与测试。该作业要求编程并调试通过。对你设计的类要有详细的文字说明(类所封装的内容,属性、方法的功能与含义等)。

2 {
3 public static void main(String args[])
4 {
5 System.out.println("Hello, Java world!");
6 // define two complex numbers
7 Complex a = new Complex(1.0, 1.0);
8 Complex b = new Complex(-4.0, 3.0);
9 Complex c;
10 // apply basic numerical operations on defined complex objects
11 System.out.println("First one:");
12 a.Show();
13 System.out.println("Second one:");
14 b.Show();
15 System.out.println("Add:");
16 c = Add(a, b);
17 c.Show();
18 System.out.println("Subtract:");
19 c = Subtract(a, b);
20 c.Show();
21 System.out.println("Multiply:");
22 c = Multiply(a, b);
23 c.Show();
24 System.out.println("Divide:");
25 c = Divide(a, b);
26 c.Show();
27 }
28 // definition of basic numerical operations
29 private static Complex Add(Complex a, Complex b)
30 {
31 return new Complex(a.real + b.real, a.imag + b.imag);
32 }
33 private static Complex Subtract(Complex a, Complex b)
34 {
35 return new Complex(a.real - b.real, a.imag - b.imag);
36 }
37 private static Complex Multiply(Complex a, Complex b)
38 {
39 return new Complex(a.real * b.real - a.imag * b.imag, a.real * b.imag + a.imag * b.real);
40 }
41 private static Complex Divide(Complex a, Complex b)
42 {
43 return new Complex((a.real * b.real + a.imag * b.imag) / b.Magnitude(),
44 (a.imag * b.real - a.real * b.imag) / b.Magnitude());
45 }
46 }
47
48 // Complex number class
49 class Complex
50 {
51 // definition of real and imaginary part
52 double real, imag;
53 // construction
54 Complex(double a, double b)
55 {
56 real = a;
57 imag = b;
58 }
59 // calculate angle
60 double Angle()
61 {
62 return Math.atan(imag / real);
63 }
64 // calculate magnitude
65 double Magnitude()
66 {
67 return Math.sqrt(real * real + imag * imag);
68 }
69 // show this number
70 void Show()
71 {
72 System.out.println("Complex Number: " + real + " + " + imag + "i\n");
73 }
74 }
作业2:设计一个处理复数运算的类,该类中至少有两个成员方法:复数加法和复数减法运算。在上述类中添加两个静态方法:求一个复数的模和求一个复数的复角。设计一个子类,该子类继承了上述的类,并且至少拥有自己的两个方法:复数乘法和复数除法。再设计一个类,包含main方法,对上面的类进行应用与测试。

2 {
3 public static void main(String args[])
4 {
5 System.out.println("Hello, Java world!");
6 // define two complex numbers
7 newComplex a = new newComplex(); a.real = 0; a.imag = 1;
8 // apply basic numerical operations on defined complex objects
9 System.out.println("First one:");
10 a.Show();
11 System.out.println("Second one:");
12 System.out.println("Add:");
13 a.Add(0, 1);
14 a.Show();
15 System.out.println("Subtract:");
16 a.Subtract(0, 1);
17 a.Show();
18 System.out.println("Multiply:");
19 a.Multiply(0, 1);
20 a.Show();
21 System.out.println("Divide:");
22 a.Divide(0, 1);
23 a.Show();
24 }
25 }
26
27 // Complex number class
28 class Complex
29 {
30 // definition of real and imaginary part
31 double real, imag;
32 // calculate angle
33 double Angle()
34 {
35 return Math.atan(imag / real);
36 }
37 // calculate magnitude
38 double Magnitude()
39 {
40 return Math.sqrt(real * real + imag * imag);
41 }
42 // show this number
43 void Show()
44 {
45 System.out.println("Complex Number: " + real + " + " + imag + "i\n");
46 }
47 // basic numerical operations
48 void Add(double a, double b)
49 {
50 real = real + a;
51 imag = imag + b;
52 }
53 void Subtract(double a, double b)
54 {
55 real = real - a;
56 imag = imag - b;
57 }
58 }
59
60 //new complex number class
61 class newComplex extends Complex
62 {
63 // more advanced operations
64 void Multiply(double a, double b)
65 {
66 double new_real = real * a - imag * b, new_imag = real * b + imag * a;
67 real = new_real;
68 imag = new_imag;
69 }
70 void Divide(double a, double b)
71 {
72 Complex c = new Complex(); c.real = a; c.imag = b;
73 double new_real = (real * a + imag * b) / c.Magnitude();
74 double new_imag = (imag * a - real * b) / c.Magnitude();
75 real = new_real;
76 imag = new_imag;
77 }
78 }
作业3:编写一个线段类 MyLine,要求如下:主要属性有: x ,y ;类型为Point (查看API)。编写构造方法,如 MyLine(Point p1 , Point p2)。编写5个成员方法。如:检查线段是否位于第一象限check()…求线段的长度 length()…判断两条直线是否相交(另一线段作为参数)。编写一点到该线段(或延长线)的距离,其他方法。注:编写方法时,考虑利用Point类的方法。编写测试程序。

2
3 public class Homework_3
4 {
5 public static void main(String args[])
6 {
7 System.out.println("Hello, Java world!");
8 // define two points
9 Point a = new Point(1, 3);
10 Point b = new Point(3, 1);
11 MyLine l = new MyLine(a, b);
12 // test if in the first quadrant
13 if (l.if_first_Quadrant())
14 System.out.println("This line is in the first quadrant!");
15 else
16 System.out.println("This line is not in the first quadrant!");
17 // print the length of this line
18 System.out.println("The length of this line is " + l.length());
19 // print the mid point of this line
20 Point c = l.midPoint();
21 System.out.println("The mid point is (" + c.getX() + ", " + c.getY() + ")");
22 // print the slope of this line
23 System.out.println("The slope of this line is " + l.direction());
24 // check if the mid point is on the line
25 if (l.if_on_line(c))
26 System.out.println("The mid point is on this line!");
27 else
28 System.out.println("The mid point is not on this line!");
29 }
30 }
31
32 // MyLine class
33 class MyLine
34 {
35 // definition of x and y
36 Point x, y;
37
38 MyLine(Point p1, Point p2)
39 {
40 x = p1; y = p2;
41 }
42
43 // check if this line is in the first Quadrant
44 boolean if_first_Quadrant()
45 {
46 if (x.getX() > 0 && x.getY() > 0 && y.getX() > 0 && y.getY() > 0)
47 return true;
48 else
49 return false;
50 }
51
52 // return the length of this line
53 double length()
54 {
55 return Math.sqrt((x.getX() - y.getX()) * (x.getX() - y.getX()) +
56 (x.getY() - y.getY()) * (x.getY() - y.getY()));
57 }
58
59 // return midPoint on this line
60 Point midPoint()
61 {
62 return new Point ((int)((x.getX() + y.getX()) / 2), (int)(x.getY() + y.getY()) / 2);
63 }
64
65 // return direction of the line
66 double direction()
67 {
68 return (x.getY() - y.getY()) / (x.getX() - y.getX());
69 }
70
71 // check if given point is on this line
72 boolean if_on_line(Point p)
73 {
74 if ((p.getX() < x.getX() && p.getX() < y.getX()) ||
75 (p.getX() > x.getX() && p.getX() > y.getX()))
76 return false;
77 else if ((x.getY() - y.getY()) / (x.getX() - y.getX()) == (x.getY() - p.getY()) / (x.getX() - p.getX()))
78 return true;
79 return false;
80 }
81 }
82
83 // Complex number class
84 class Complex
85 {
86 // definition of real and imaginary part
87 double real, imag;
88 // calculate angle
89 double Angle()
90 {
91 return Math.atan(imag / real);
92 }
93 // calculate magnitude
94 double Magnitude()
95 {
96 return Math.sqrt(real * real + imag * imag);
97 }
98 // show this number
99 void Show()
100 {
101 System.out.println("Complex Number: " + real + " + " + imag + "i\n");
102 }
103 // basic numerical operations
104 void Add(double a, double b)
105 {
106 real = real + a;
107 imag = imag + b;
108 }
109 void Subtract(double a, double b)
110 {
111 real = real - a;
112 imag = imag - b;
113 }
114 }
115
116 //new complex number class
117 class newComplex extends Complex
118 {
119 // more advanced operations
120 void Multiply(double a, double b)
121 {
122 double new_real = real * a - imag * b, new_imag = real * b + imag * a;
123 real = new_real;
124 imag = new_imag;
125 }
126 void Divide(double a, double b)
127 {
128 Complex c = new Complex(); c.real = a; c.imag = b;
129 double new_real = (real * a + imag * b) / c.Magnitude();
130 double new_imag = (imag * a - real * b) / c.Magnitude();
131 real = new_real;
132 imag = new_imag;
133 }
134 }
作业4:完成一个application图形界面程序。用户界面要求见如下两图:
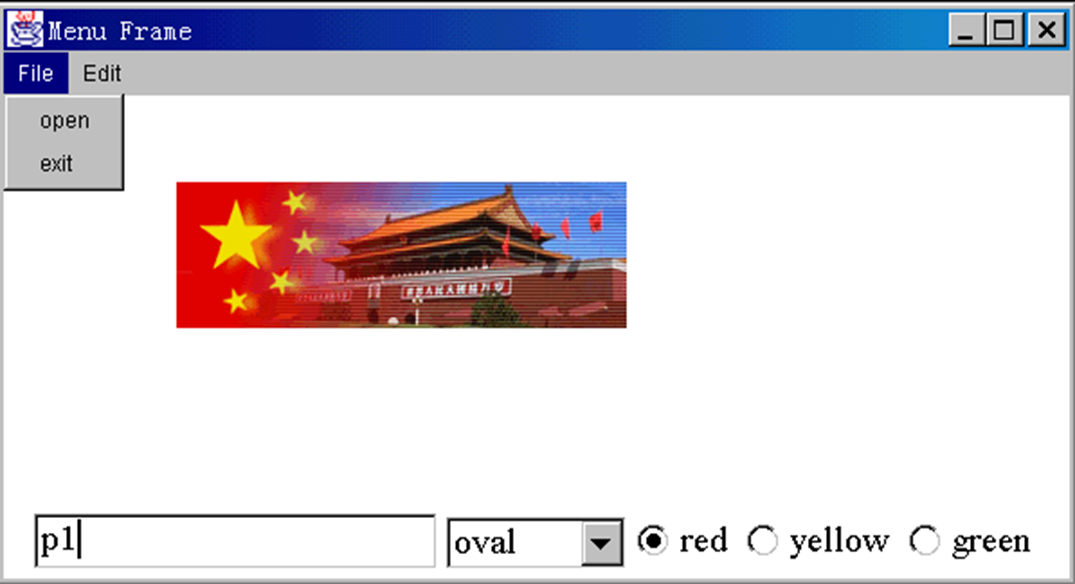
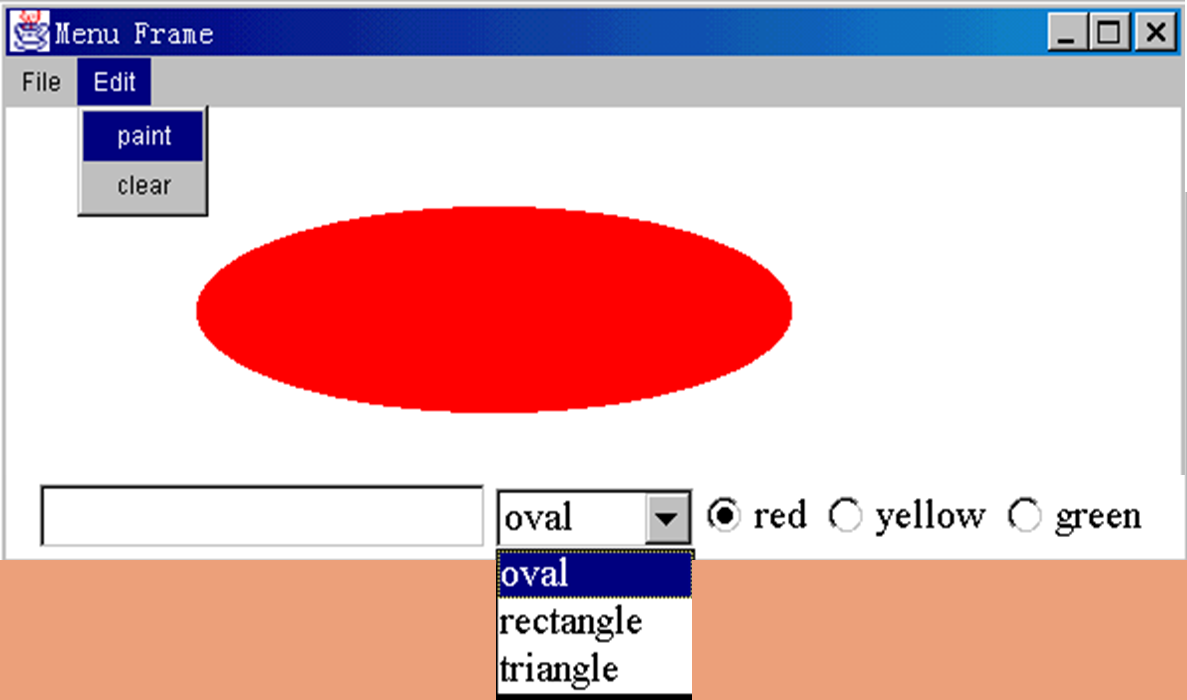

2 import java.awt.event.ActionEvent;
3 import java.awt.event.ActionListener;
4 import javax.swing.*;
5
6 public class Homework_4
7 {
8 // create a simple GUI window
9 public static void main(String[] args)
10 {
11 MyFrame myFrame = new MyFrame();
12 }
13
14 }
15
16 class MyFrame extends JFrame
17 {
18 private JLabel label;
19 private JFileChooser fileChooser;
20 private JTextArea textArea;
21 private JComboBox comboBox;
22 private ButtonGroup buttonGroup;
23 private String color, shape;
24
25 public MyFrame()
26 {
27 // create and setup the window
28 super("Menu Frame");
29 setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
30 setLocation(300, 300);
31 setSize(new Dimension(700, 500));
32 setResizable(false);
33 try
34 {
35 UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
36 }
37 catch (Exception e)
38 {
39 System.out.println("Cannot adapt look and feel.\n");
40 }
41
42 // create menu bar
43 JMenuBar menuBar = new JMenuBar();
44 JMenu fileMenu = new JMenu("File");
45 menuBar.add(fileMenu);
46 JMenu editMenu = new JMenu("Edit");
47 menuBar.add(editMenu);
48 setJMenuBar(menuBar);
49
50 // create and setup open menu item
51 JMenuItem openMenuItem = new JMenuItem("Open");
52 openMenuItem.addActionListener(new ImageOpenListener());
53 fileMenu.add(openMenuItem);
54
55 // create and setup exit menu item
56 JMenuItem exitMenuItem = new JMenuItem("Exit");
57 exitMenuItem.addActionListener(new ActionListener()
58 {
59 public void actionPerformed(ActionEvent e)
60 {
61 System.exit(0);
62 }
63 });
64 fileMenu.add(exitMenuItem);
65
66 // create paint menu item
67 JMenuItem paintMenuItem = new JMenuItem("Paint");
68 paintMenuItem.addActionListener(new ActionListener()
69 {
70 public void actionPerformed(ActionEvent e)
71 {
72 textArea.setText(null);
73 color = buttonGroup.getSelection().getActionCommand();
74 shape = (String) comboBox.getSelectedItem();
75 label.setIcon(new MyShape(400, 300));
76 label.setHorizontalAlignment(SwingConstants.CENTER);
77 }
78 });
79 editMenu.add(paintMenuItem);
80
81 // create clear menu item
82 JMenuItem clearMenuItem = new JMenuItem("Clear");
83 clearMenuItem.addActionListener(new ActionListener()
84 {
85 public void actionPerformed(ActionEvent e)
86 {
87 label.setText(null);
88 label.setIcon(null);
89 textArea.setText(null);
90 }
91 });
92 editMenu.add(clearMenuItem);
93
94 // create label and file chooser to display a image
95 label = new JLabel();
96 getContentPane().add(label, BorderLayout.NORTH);
97 fileChooser=new JFileChooser();
98
99 // create text area
100 textArea = new JTextArea(1, 50);
101 textArea.setEnabled(false);
102
103 // create combo box
104 comboBox = new JComboBox();
105 comboBox.addItem("oval");
106 comboBox.addItem("rectangle");
107 comboBox.addItem("triangle");
108
109 // create radio button
110 JRadioButton radioButtonRed = new JRadioButton("red");
111 radioButtonRed.setActionCommand("red");
112 JRadioButton radioButtonYellow = new JRadioButton("Yellow");
113 radioButtonYellow.setActionCommand("yellow");
114 JRadioButton radioButtonBlue = new JRadioButton("Blue");
115 radioButtonBlue.setActionCommand("blue");
116 buttonGroup = new ButtonGroup();
117 buttonGroup.add(radioButtonRed);
118 buttonGroup.add(radioButtonYellow);
119 buttonGroup.add(radioButtonBlue);
120 radioButtonRed.setSelected(true); // set initial choice for radio button group
121
122 // create a panel containing text area, combo box and radio choice
123 JPanel panel = new JPanel();
124 getContentPane().add(panel, BorderLayout.SOUTH);
125 panel.add(textArea, BorderLayout.WEST);
126 panel.add(comboBox, BorderLayout.CENTER);
127 panel.add(radioButtonRed, BorderLayout.EAST);
128 panel.add(radioButtonYellow, BorderLayout.EAST);
129 panel.add(radioButtonBlue, BorderLayout.EAST);
130
131 // display the window
132 setVisible(true);
133 }
134
135 // create listener for opening images
136 private class ImageOpenListener implements ActionListener
137 {
138 public void actionPerformed(ActionEvent e)
139 {
140 fileChooser.showOpenDialog(MyFrame.this);
141 String imageName = fileChooser.getSelectedFile().getPath();
142 ImageIcon imageIcon = new ImageIcon(imageName);
143 label.setIcon(imageIcon);
144 label.setHorizontalAlignment(SwingConstants.CENTER);
145 textArea.setText(imageName);
146 }
147 }
148
149 // setup MyShape icon to draw different shapes with different colors
150 private class MyShape implements Icon
151 {
152 private int width, height;
153 public MyShape(int wid, int ht)
154 {
155 width = wid;
156 height = ht;
157 }
158 public void paintIcon(Component container, Graphics g, int p, int q)
159 {
160 if (color == "red")
161 {
162 g.setColor(new Color(255, 0, 0));
163 }
164 else if (color == "yellow")
165 {
166 g.setColor(new Color(255, 255, 0));
167 }
168 else if (color == "blue")
169 {
170 g.setColor(new Color(0, 0, 255));
171 }
172 if (shape == "oval")
173 {
174 g.drawOval(p, q, width - 1, height - 1);
175 g.fillOval(p, q, width - 1, height - 1);
176 }
177 else if (shape == "rectangle")
178 {
179 g.drawRect(p, q, width - 1, height - 1);
180 g.fillRect(p, q, width - 1, height - 1);
181 }
182 else if (shape == "triangle")
183 {
184 Polygon polygon = new Polygon();
185 polygon.addPoint(150, 300);
186 polygon.addPoint(550, 300);
187 polygon.addPoint(300, 0);
188 g.drawPolygon(polygon);
189 g.fillPolygon(polygon);
190 }
191 }
192 public int getIconWidth()
193 {
194 return width;
195 }
196 public int getIconHeight()
197 {
198 return height;
199 }
200 }
201 }
编译和运行:
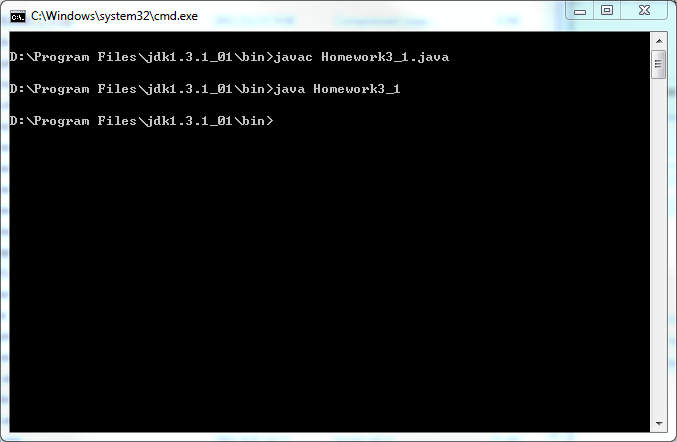
作业3最终的界面展示如下:
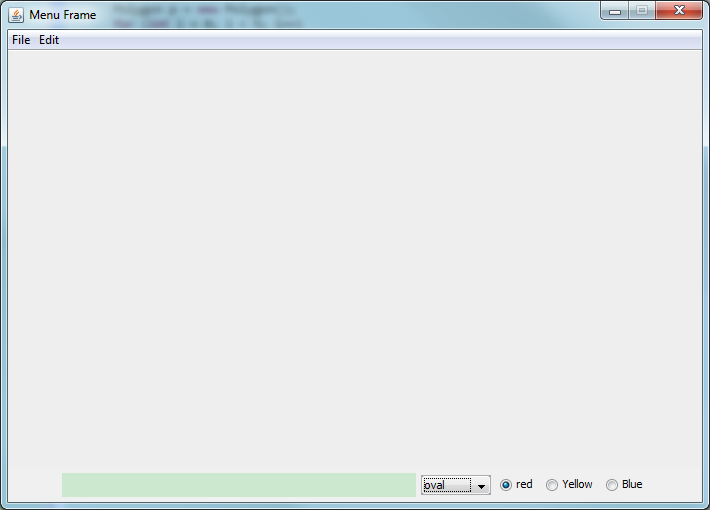
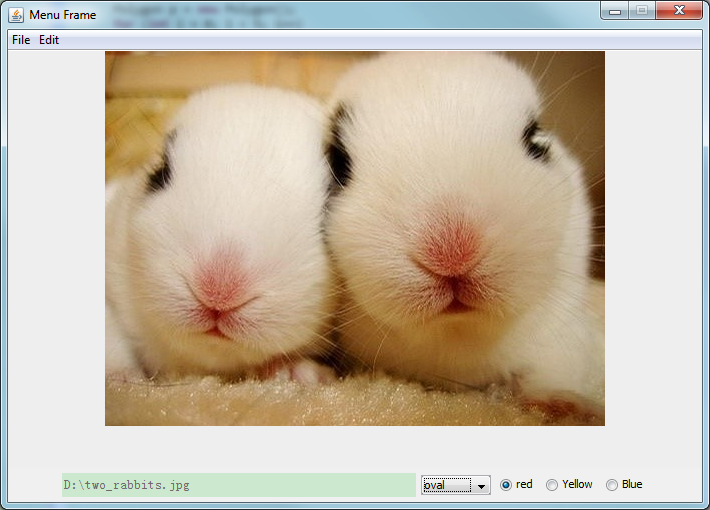
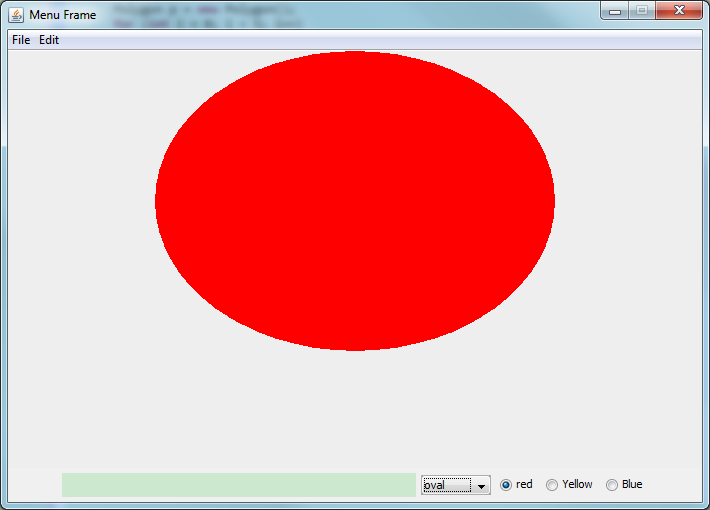
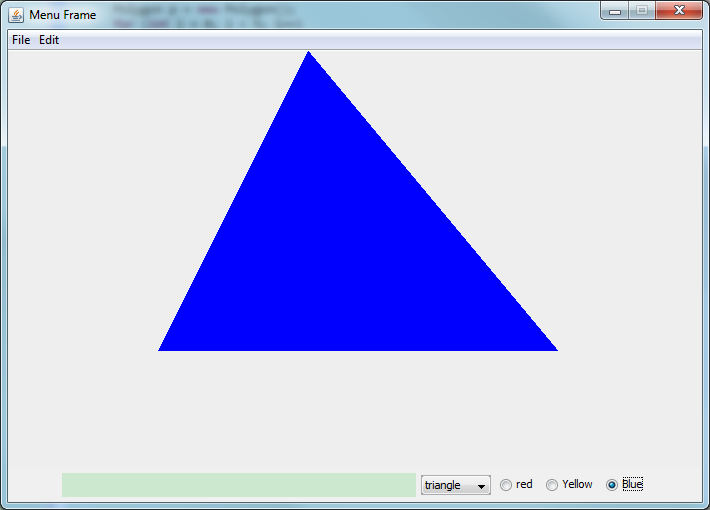
作业5:将作业4中的程序改为 applet 小程序,并编写相应的 HTML 文件。
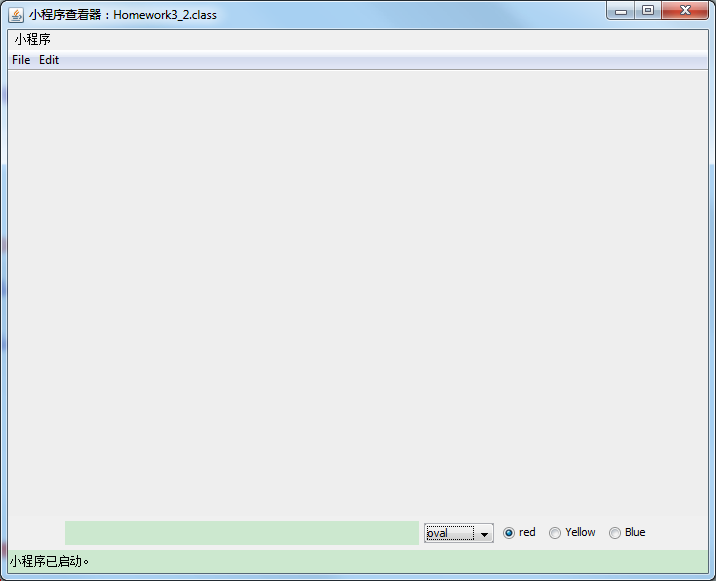
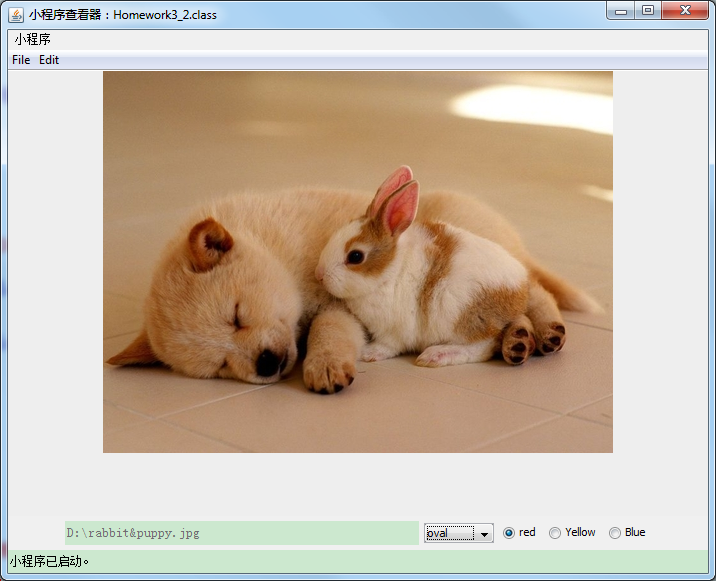
程序要有明确的功能,调试通过并能正常运行。
程序至少要包含以下知识点:
GUI 图形界面
多线程
文件I/O或网络编程
程序代码在500行以上。
作业要求:
1、规划出实现这个项目所需要的类;
2、根据设计要求作出类图及类相应的关系;
3、编写代码实现设计。
[to be continued]
posted on 2012-01-03 17:10 J. V. King 阅读(4623) 评论(0) 编辑 收藏 举报