1 #include<cstdio> 2 #include<cstring> 3 #include<queue> 4 #define MAXN 2000010 5 #define MAXM 50000 6 #define MAXL 128 7 using namespace std; 8 char str[MAXN], dic[MAXM][60]; 9 int size, cnt[MAXM]; 10 struct node { 11 int pos, fail, next[MAXL]; 12 void Init() { 13 pos = fail = 0; 14 memset(next, 0, sizeof(next)); 15 } 16 }; 17 node tree[MAXM]; 18 void Insert(char *s, int pos) { 19 int now, t; 20 for (now = 0; *s; s++) { 21 t = *s; 22 if (!tree[now].next[t]) { 23 tree[++size].Init(); 24 tree[now].next[t] = size; 25 } 26 now = tree[now].next[t]; 27 } 28 tree[now].pos = pos; 29 } 30 void BFS() { 31 int now, i, p; 32 queue<int> q; 33 q.push(0); 34 while (!q.empty()) { 35 now = q.front(); 36 q.pop(); 37 for (i = 'A'; i <= 'Z'; i++) { 38 if (tree[now].next[i]) { 39 p = tree[now].next[i]; 40 if (now) 41 tree[p].fail = tree[tree[now].fail].next[i]; 42 q.push(p); 43 } else 44 tree[now].next[i] = tree[tree[now].fail].next[i]; 45 } 46 } 47 } 48 void Match(char *s) { 49 int now, t, p; 50 for (now = 0; *s; s++) { 51 t = *s; 52 now = tree[now].next[t]; 53 for (p = now; p; p = tree[p].fail) 54 cnt[tree[p].pos]++; 55 } 56 } 57 int main() { 58 int n, i; 59 while (~scanf("%d", &n)) { 60 tree[size = 0].Init(); 61 memset(cnt, 0, sizeof(cnt)); 62 for (i = 1; i <= n; i++) { 63 scanf(" %s", dic[i]); 64 Insert(dic[i], i); 65 } 66 BFS(); 67 scanf(" %s", str); 68 Match(str); 69 for (i = 1; i <= n; i++) { 70 if (cnt[i]) 71 printf("%s: %d\n", dic[i], cnt[i]); 72 } 73 } 74 return 0; 75 }
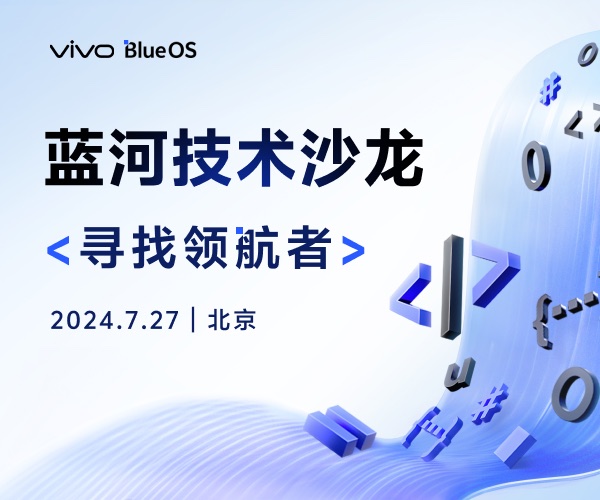