1 #include<cstdio> 2 #include<stack> 3 #define MAXN 50010 4 #define MAX(a,b) ((a)>(b)?(a):(b)) 5 using namespace std; 6 stack<int>st; 7 struct node 8 { 9 int left,right; 10 }; 11 node tree[MAXN<<2]; 12 void Build(int L,int R,int rt) 13 { 14 tree[rt].left=tree[rt].right=R-L+1; 15 if(L!=R) 16 { 17 int mid=(L+R)>>1; 18 Build(L,mid,rt<<1); 19 Build(mid+1,R,rt<<1|1); 20 } 21 } 22 inline void PushUp(int mid,int L,int R,int rt) 23 { 24 tree[rt].left=tree[rt<<1].left; 25 tree[rt].right=tree[rt<<1|1].right; 26 if(tree[rt].left==mid-L+1) 27 tree[rt].left+=tree[rt<<1|1].left; 28 if(tree[rt].right==R-mid) 29 tree[rt].right+=tree[rt<<1].right; 30 } 31 void Update(int x,int val,int L,int R,int rt) 32 { 33 if(L==R) 34 tree[rt].left=tree[rt].right=val; 35 else 36 { 37 int mid=(L+R)>>1; 38 if(x<=mid) 39 Update(x,val,L,mid,rt<<1); 40 else 41 Update(x,val,mid+1,R,rt<<1|1); 42 PushUp(mid,L,R,rt); 43 } 44 } 45 int Query(int x,int L,int R,int rt) 46 { 47 if(L==R) 48 return tree[rt].left; 49 int mid=(L+R)>>1; 50 if(x<=mid) 51 { 52 if(tree[rt<<1].left==mid-L+1) 53 return tree[rt<<1].left+tree[rt<<1|1].left; 54 else if(L+tree[rt<<1].left>x) 55 return tree[rt<<1].left; 56 else if(x>mid-tree[rt<<1].right) 57 return tree[rt<<1].right+tree[rt<<1|1].left; 58 else 59 return Query(x,L,mid,rt<<1); 60 } 61 else 62 { 63 if(tree[rt<<1|1].right==R-mid) 64 return tree[rt<<1|1].right+tree[rt<<1].right; 65 else if(x<mid+1+tree[rt<<1|1].left) 66 return tree[rt<<1].right+tree[rt<<1|1].left; 67 else if(x>R-tree[rt<<1|1].right) 68 return tree[rt<<1|1].right; 69 else 70 return Query(x,mid+1,R,rt<<1|1); 71 } 72 } 73 int main() 74 { 75 int n,m,x; 76 char ch; 77 while(~scanf("%d%d",&n,&m)) 78 { 79 Build(1,n,1); 80 for(;!st.empty();st.pop()); 81 while(m--) 82 { 83 scanf(" %c",&ch); 84 if(ch=='D') 85 { 86 scanf("%d",&x); 87 st.push(x); 88 Update(x,0,1,n,1); 89 } 90 else if(ch=='Q') 91 { 92 scanf("%d",&x); 93 printf("%d\n",Query(x,1,n,1)); 94 } 95 else 96 { 97 if(!st.empty()) 98 { 99 Update(st.top(),1,1,n,1); 100 st.pop(); 101 } 102 } 103 } 104 } 105 return 0; 106 }
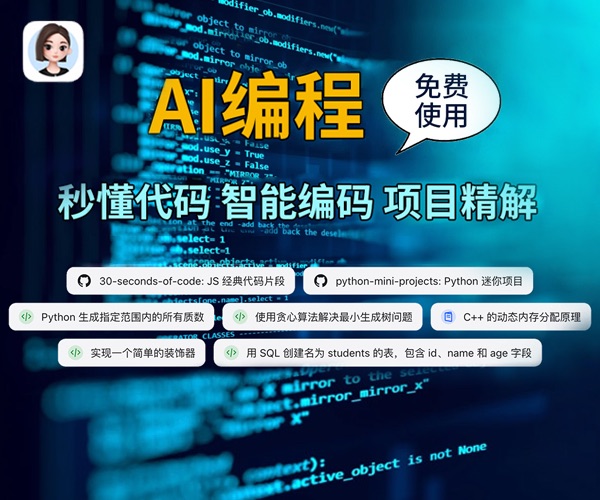