1 #include<cstdio> 2 #include<cstring> 3 #define MAXN 32010 4 int tree[MAXN<<2],ans[MAXN]; 5 inline void PushUp(int rt) 6 { 7 tree[rt]=tree[rt<<1]+tree[rt<<1|1]; 8 } 9 void Update(int x,int L,int R,int rt) 10 { 11 if(L==R) 12 tree[rt]++; 13 else 14 { 15 int mid=(L+R)>>1; 16 if(x<=mid) 17 Update(x,L,mid,rt<<1); 18 else 19 Update(x,mid+1,R,rt<<1|1); 20 PushUp(rt); 21 } 22 } 23 int Query(int x,int y,int L,int R,int rt) 24 { 25 if(x<=L&&R<=y) 26 return tree[rt]; 27 int mid=(L+R)>>1,ans=0; 28 if(x<=mid) 29 ans+=Query(x,y,L,mid,rt<<1); 30 if(y>mid) 31 ans+=Query(x,y,mid+1,R,rt<<1|1); 32 return ans; 33 } 34 int main() 35 { 36 int n,i,x,y; 37 while(~scanf("%d",&n)) 38 { 39 memset(ans,0,sizeof(ans)); 40 memset(tree,0,sizeof(tree)); 41 for(i=0;i<n;i++) 42 { 43 scanf("%d%d",&x,&y); 44 x++; 45 ans[Query(1,x,1,MAXN,1)]++; 46 Update(x,1,MAXN,1); 47 } 48 for(i=0;i<n;i++) 49 printf("%d\n",ans[i]); 50 } 51 return 0; 52 }
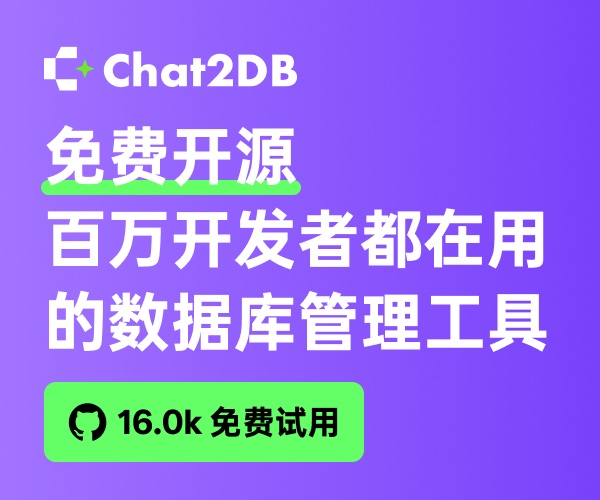
