1 #include<cstdio> 2 #include<cstring> 3 #define MIN(a,b) ((a)>(b)?(b):(a)) 4 #define MAXN 5010 5 int tree[MAXN<<2],a[MAXN]; 6 inline void PushUp(int rt) 7 { 8 tree[rt]=tree[rt<<1]+tree[rt<<1|1]; 9 } 10 void Update(int x,int L,int R,int rt) 11 { 12 if(L==R) 13 tree[rt]=1; 14 else 15 { 16 int mid=(L+R)>>1; 17 if(mid>=x) 18 Update(x,L,mid,rt<<1); 19 else 20 Update(x,mid+1,R,rt<<1|1); 21 PushUp(rt); 22 } 23 } 24 int Query(int x,int y,int L,int R,int rt) 25 { 26 if(x<=L&&R<=y) 27 return tree[rt]; 28 else 29 { 30 int mid,ans; 31 ans=0; 32 mid=(L+R)>>1; 33 if(x<=mid) 34 ans+=Query(x,y,L,mid,rt<<1); 35 if(y>mid) 36 ans+=Query(x,y,mid+1,R,rt<<1|1); 37 return ans; 38 } 39 } 40 int main() 41 { 42 int n,i,ans,temp; 43 while(~scanf("%d",&n)) 44 { 45 memset(tree,0,sizeof(tree)); 46 for(ans=i=0;i<n;i++) 47 { 48 scanf("%d",&a[i]); 49 a[i]++; 50 ans+=Query(a[i],n,1,n,1); 51 Update(a[i],1,n,1); 52 } 53 temp=ans; 54 for(i=0;i<n;i++) 55 { 56 temp+=n+1-(a[i]<<1); 57 ans=MIN(ans,temp); 58 } 59 printf("%d\n",ans); 60 } 61 return 0; 62 }
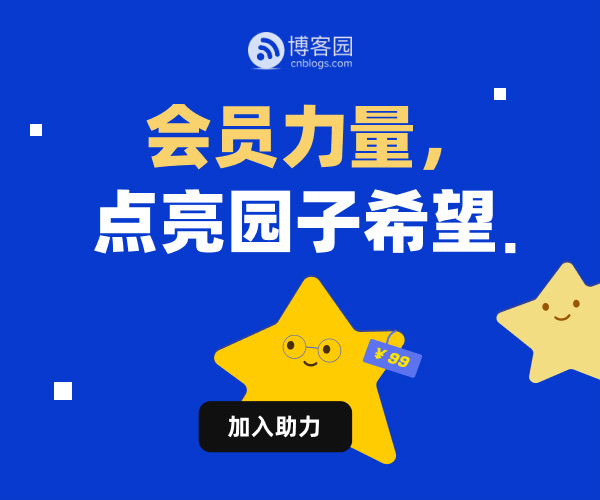